Handling and Preventing ClassCastException in Java
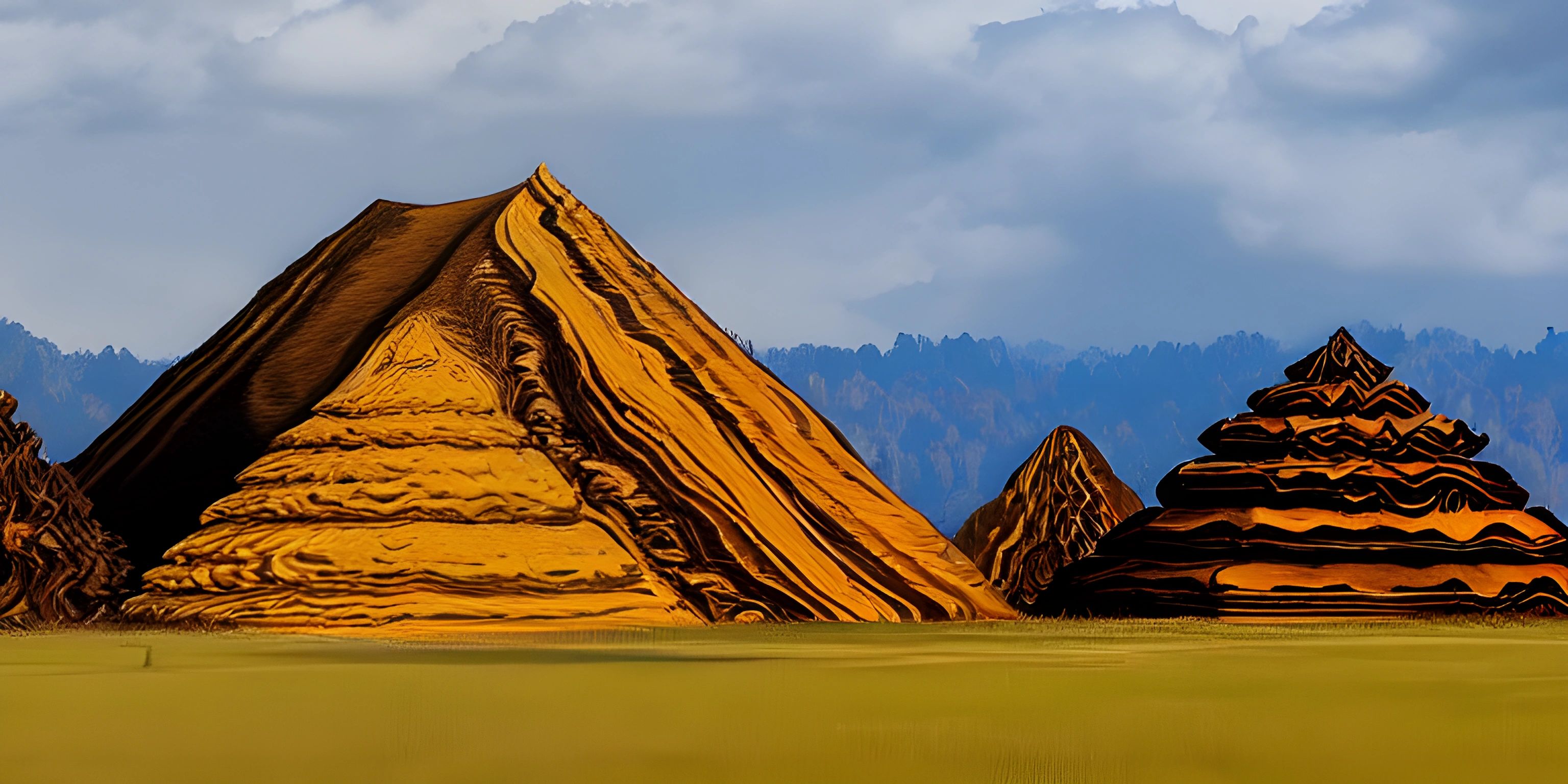
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
ClassCastException is a common runtime error in Java that occurs when an object is improperly cast to a type it does not belong to. In this article, we'll explore what causes ClassCastException, how to handle it, and how to prevent it from occurring in the first place.
Understanding ClassCastException
ClassCastException is a subclass of RuntimeException and is thrown when the Java Virtual Machine (JVM) performs an illegal cast. A cast is a way of changing the type of an object to another type. Usually, it happens when you try to cast an object of one class to a type of another class that's not in the same inheritance hierarchy.
Here's an example of code that will trigger a ClassCastException:
Object obj = new String("Hello Cratecode!"); Integer num = (Integer) obj; // ClassCastException
In this example, we have an object obj
of type String. We're attempting to cast it to an Integer, which is not in the same inheritance hierarchy. This will cause a ClassCastException at runtime.
Handling ClassCastException
One way to handle ClassCastException is by using a try-catch block. When you expect a ClassCastException to occur, wrap the code that may cause the exception in a try block, and catch the exception in a catch block.
Object obj = new String("Hello Cratecode!"); try { Integer num = (Integer) obj; } catch (ClassCastException e) { System.out.println("ClassCastException encountered: " + e.getMessage()); }
In this example, if a ClassCastException occurs, the code inside the catch block will be executed, allowing your program to continue running without crashing.
Preventing ClassCastException
The best way to avoid ClassCastException is to check the type of an object before casting. You can use the instanceof
keyword to determine if an object is an instance of a specific class or interface.
Object obj = new String("Hello Cratecode!"); if (obj instanceof Integer) { Integer num = (Integer) obj; } else { System.out.println("Cannot cast to Integer"); }
In this example, we first check if obj
is an instance of Integer before attempting to cast it. This prevents the ClassCastException from occurring.
Conclusion
ClassCastException can be a common pitfall for Java programmers. To avoid it, always ensure that you're casting objects to types that are in the same inheritance hierarchy. By using try-catch blocks and the instanceof
keyword, you can handle and prevent ClassCastException in your Java programs.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Chat App Backend (psst, it's free!).
FAQ
What is ClassCastException?
ClassCastException is a runtime exception in Java that occurs when an object is improperly cast to a type it does not belong to, usually when trying to cast an object of one class to a type of another class that's not in the same inheritance hierarchy.
How can I handle ClassCastException?
You can handle ClassCastException by using a try-catch block. Wrap the code that may cause the exception in a try block and catch the exception in a catch block. This will allow your program to continue running without crashing if a ClassCastException occurs.
How can I prevent ClassCastException?
You can prevent ClassCastException by checking the type of an object before casting it. Use the instanceof
keyword to determine if an object is an instance of a specific class or interface, and only attempt the cast if the object is an instance of the target type. This will help prevent ClassCastException from occurring.