Java Exceptions
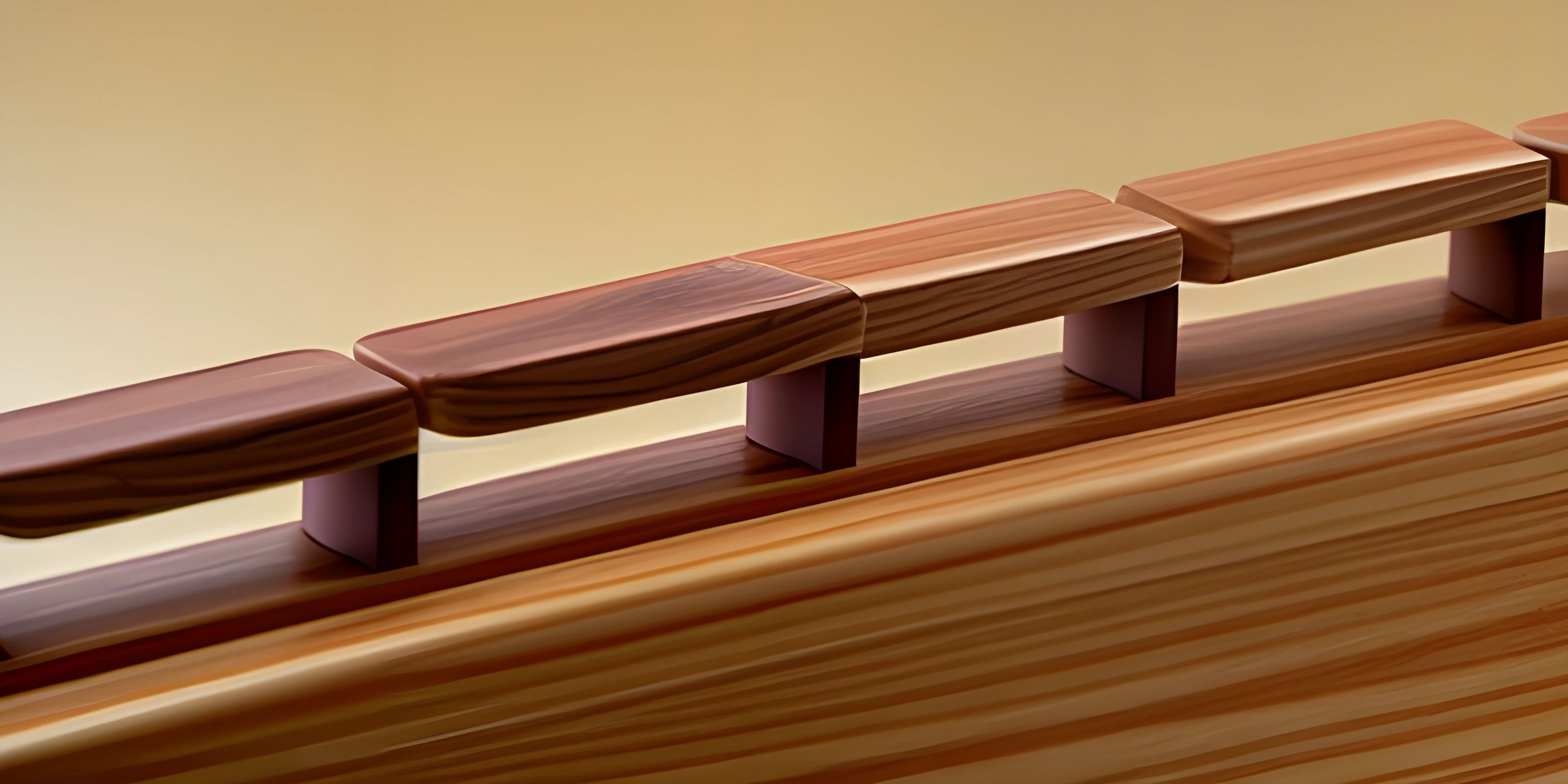
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In Java, exceptions are events that occur during the execution of a program, often disrupting its normal flow. These exceptions are important to manage because they can cause your program to crash or behave unexpectedly. Let's dig into understanding Java exceptions and how to handle them.
Types of Exceptions
There are mainly two types of exceptions in Java: checked exceptions and unchecked exceptions. Exceptions in Java are objects that inherit from the Throwable
class, which is further divided into Exception
and Error
classes.
Checked Exceptions
Checked exceptions are those that are checked by the Java compiler during the compilation process. They are typically caused by external factors, like issues with the file system or network connections. Examples of checked exceptions include IOException
, FileNotFoundException
, and ClassNotFoundException
.
When you write code that may cause a checked exception, the Java compiler requires you to handle the exception using a try-catch block or declare it using the throws
keyword.
Unchecked Exceptions
Unchecked exceptions, also known as runtime exceptions, are not checked by the Java compiler during compilation. They often result from incorrect programming logic, such as dividing by zero or accessing an element outside the bounds of an array. Examples of unchecked exceptions include NullPointerException
, ArrayIndexOutOfBoundsException
, and ArithmeticException
.
Unlike checked exceptions, you don't need to handle unchecked exceptions explicitly, although doing so can help prevent your application from crashing unexpectedly.
Handling Exceptions
To handle exceptions in Java, we use try-catch blocks and the finally
keyword. Let's explore these techniques.
Try-Catch Block
A try-catch block is used to surround a block of code that might throw an exception. If an exception occurs within the try block, the program jumps to the corresponding catch block to handle the exception.
Here's an example demonstrating the use of a try-catch block:
public class ExceptionHandlingExample { public static void main(String[] args) { try { int result = 10 / 0; System.out.println("Result: " + result); } catch (ArithmeticException e) { System.out.println("Caught an exception: " + e.getMessage()); } } }
In this example, the division operation within the try block causes an ArithmeticException
since we're dividing by zero. The catch block captures the exception and prints a message, preventing the application from crashing.
Finally Block
The finally
block is an optional block that follows the try-catch block. It contains code that must be executed, regardless of whether an exception occurred or not. This is particularly useful for cleaning up resources, such as closing files or network connections.
Here's an example demonstrating the use of a finally block:
public class FinallyExample { public static void main(String[] args) { try { int result = 10 / 2; System.out.println("Result: " + result); } catch (ArithmeticException e) { System.out.println("Caught an exception: " + e.getMessage()); } finally { System.out.println("This will always be executed."); } } }
In this example, regardless of whether an exception occurs, the code within the finally block will be executed.
Now that you've learned about Java exceptions, their types, and how to handle them, you're ready to create more robust and resilient applications. Good luck and happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Enums (psst, it's free!).
FAQ
What are the two main types of exceptions in Java?
The two main types of exceptions in Java are checked exceptions and unchecked exceptions. Checked exceptions are checked by the compiler during compilation, while unchecked exceptions, also known as runtime exceptions, are not checked by the compiler.
How do you handle exceptions in Java?
In Java, exceptions are handled using try-catch blocks and the optional finally block. The try block contains the code that might throw an exception. If an exception occurs, the program moves to the corresponding catch block to handle the exception. The finally block is used for code that must be executed regardless of whether an exception occurred or not.
What is the difference between the `Exception` and `Error` classes in Java?
Both Exception
and Error
classes inherit from the Throwable
class in Java. The Exception
class represents checked and unchecked exceptions that can be caught and handled by the programmer. The Error
class represents serious issues, such as VirtualMachineError
, that usually cannot be caught or handled by the programmer, and often result in the termination of the Java Virtual Machine (JVM).