Working with LLVM IR to Implement Assembly in Rust
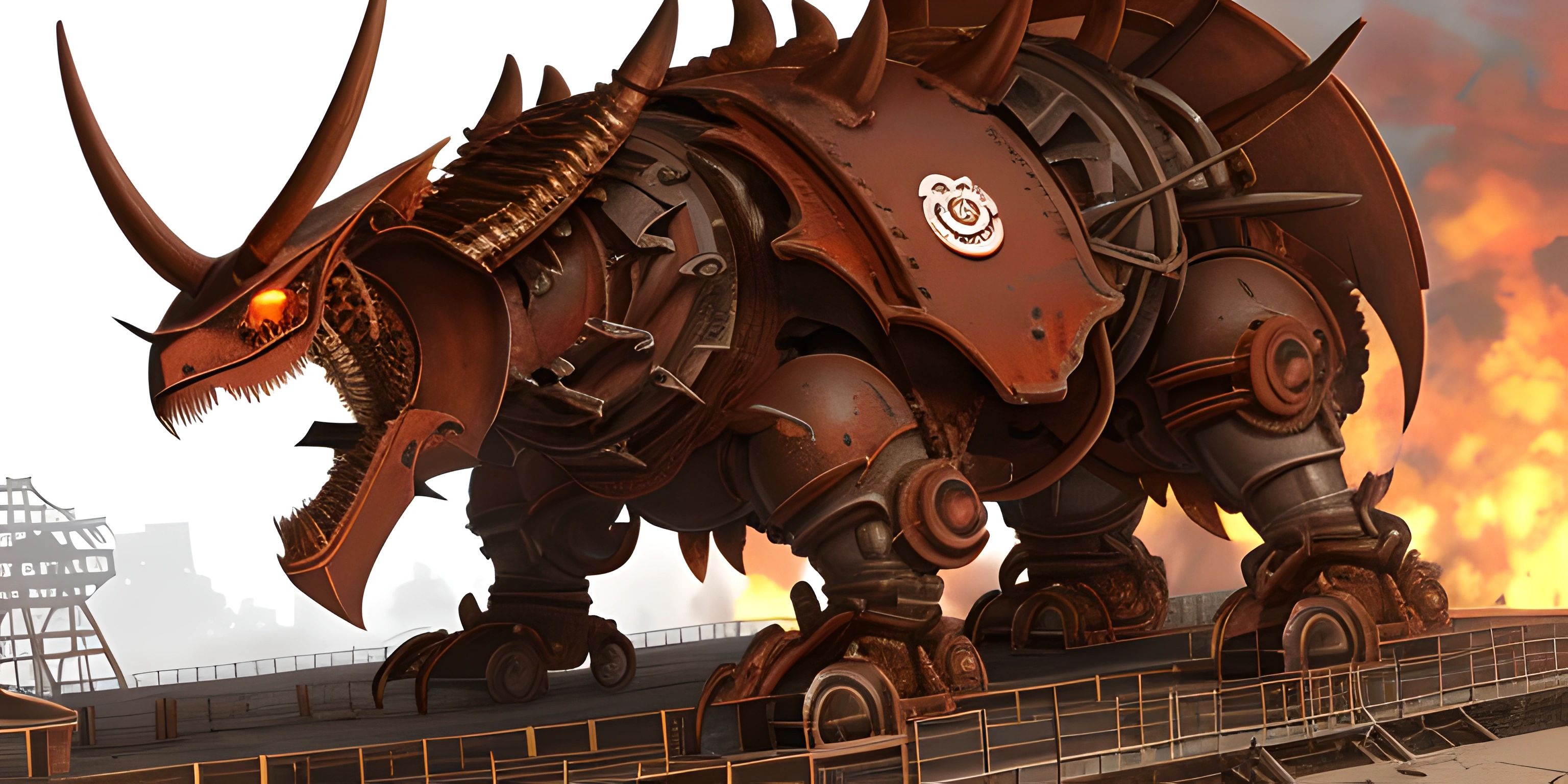
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Diving into the world of programming languages, you might have stumbled upon Assembly, a low-level programming language often used for tasks like writing bootloaders and firmware. But what if you're a fan of the Rust programming language and you want to work with Assembly? Fear not: enter LLVM Intermediate Representation (IR).
What is LLVM IR?
LLVM is a compiler infrastructure that provides a set of libraries and tools for building, optimizing, and generating machine code. LLVM IR is its intermediate language, a low-level programming language that serves as a common representation of your code throughout the different compilation stages.
Using LLVM IR, you can implement Assembly language in Rust, opening up a world of possibilities for performance optimizations, cross-platform compatibility, and low-level system programming.
Getting Started with LLVM IR in Rust
To start working with LLVM IR in Rust, you'll need to install the llvm-sys
and inkwell
crates. The llvm-sys
crate provides Rust bindings to LLVM's C API, while inkwell
is a higher-level, idiomatic Rust wrapper around llvm-sys
.
Add the following dependencies to your Cargo.toml
file:
[dependencies] llvm-sys = "110.0" inkwell = "0.3"
Now, let's dive into a simple example to see how to generate LLVM IR from Rust code.
-
Initialize the LLVM context:
First, you need to create an instance of the
inkwell::context::Context
struct, which represents an isolated LLVM context.use inkwell::context::Context; let context = Context::create();
-
Create an LLVM module:
Next, you'll create an LLVM module, which is a container for all the functions and global variables in your program.
let module = context.create_module("my_module");
-
Define your Assembly function:
Now it's time to define your Assembly function using LLVM IR. For instance, let's create a simple function that adds two integers.
use inkwell::types::BasicTypeEnum; let i32_type = context.i32_type(); let fn_type = i32_type.fn_type(&[BasicTypeEnum::Int(i32_type.into()), BasicTypeEnum::Int(i32_type.into())], false); let function = module.add_function("add", fn_type, None);
-
Implement the function body:
With the function defined, you can now implement its body using LLVM IR Builder.
use inkwell::builder::Builder; let entry = context.append_basic_block(function, "entry"); let builder = context.create_builder(); builder.position_at_end(entry); let a = function.get_nth_param(0).unwrap().into_int_value(); let b = function.get_nth_param(1).unwrap().into_int_value(); let sum = builder.build_int_add(a, b, "sum"); builder.build_return(Some(&sum));
-
Generate the LLVM IR:
Finally, convert the module into LLVM IR using the
print_to_string
method.let ir_code = module.print_to_string(); println!("{}", ir_code);
Now you have successfully generated LLVM IR from Rust code! You can continue to explore and experiment with low-level programming concepts, combining the power of Rust and Assembly to create high-performance, low-level applications.
Conclusion
Implementing Assembly in Rust using LLVM IR provides numerous benefits, such as performance optimizations and cross-platform compatibility. By using the llvm-sys
and inkwell
crates, you can easily generate LLVM IR from Rust code and dive into the world of low-level programming. With this newfound knowledge, you're ready to tackle complex projects and reach new heights in your Rust programming journey.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What is LLVM Intermediate Representation (IR)?
LLVM Intermediate Representation (IR) is a low-level programming language that serves as a common, intermediate format for various compiler optimizations and transformations. It is designed to be both human-readable and machine-friendly, making it easier to understand and work with during the development process. By using LLVM IR, you can implement Assembly language in Rust projects and benefit from its optimization features.
How do I implement Assembly language in Rust using LLVM IR?
To implement Assembly language in Rust using LLVM IR, you will need to follow these steps:
- Install the LLVM toolchain and Rust LLVM integration.
- Write your Assembly code as an LLVM IR file.
- Use Rust's
llvm_asm!
macro to include the LLVM IR in your Rust project. - Compile your Rust code with the LLVM IR file, and you'll have Assembly language working within your Rust project.
What are the benefits of using LLVM IR for implementing Assembly in Rust?
Some benefits of using LLVM IR for implementing Assembly in Rust include:
- Improved readability: LLVM IR provides a human-readable format for Assembly code, making it easier to understand and maintain.
- Compiler optimizations: LLVM IR allows you to take advantage of various compiler optimizations and transformations, resulting in more efficient code.
- Cross-platform compatibility: LLVM IR is platform-independent, which means your Assembly code can be compiled for different target architectures without any modifications.
- Integration with Rust: By using the
llvm_asm!
macro, you can seamlessly integrate Assembly code written in LLVM IR with your Rust projects.
Can I use LLVM IR for other languages besides Rust?
Yes, LLVM IR is a versatile intermediate representation that can be used with various programming languages, not just Rust. Many compilers and language frontends are built on top of LLVM, including Clang (for C and C++), Swift, and Julia. By using LLVM IR, you can implement Assembly language in projects written in these languages and take advantage of the same benefits that Rust projects enjoy.