Rust's Global Allocator
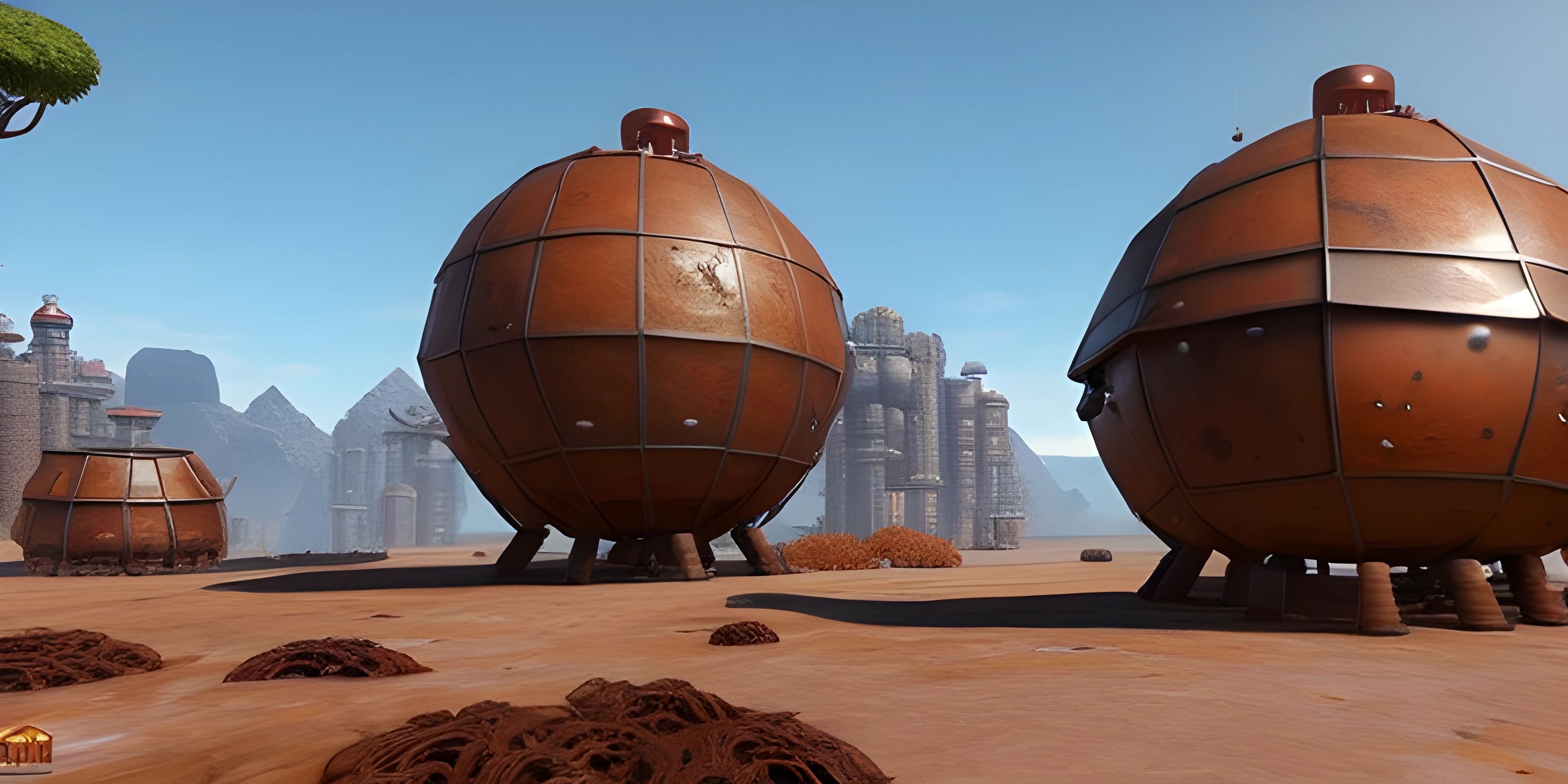
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Memory allocation in programming is like being in a hardware store with a shopping list. You've got tasks to do, and you need the right tools and materials. In the case of programming, these 'tools and materials' equate to chunks of memory space.
In Rust, one of these 'store clerks' is the Global Allocator, a critical system component that oversees the allocation and deallocation of memory. It's the one who hands you the hammer when you need it, and takes it back when you're done.
Rust's Global Allocator
The Rust programming language has a unique way of managing memory allocation. It uses a feature known as a Global Allocator, which is responsible for providing memory to your applications.
Working with Rust's Global Allocator
When you want to use large chunks of memory in Rust, you call upon the services of the Global Allocator. It's like asking the store clerk for a box of nails: you tell them what you need, and they provide it from the store's inventory.
In Rust, the Global Allocator is defined at the program level and is used for dynamic memory allocation. Here's a basic example of allocating memory in Rust:
use std::alloc::{GlobalAlloc, Layout, System}; struct MyAllocator; unsafe impl GlobalAlloc for MyAllocator { unsafe fn alloc(&self, layout: Layout) -> *mut u8 { System.alloc(layout) } unsafe fn dealloc(&self, ptr: *mut u8, layout: Layout) { System.dealloc(ptr, layout) } } #[global_allocator] static GLOBAL: MyAllocator = MyAllocator;
This code defines a new Allocator, MyAllocator
, which directly uses Rust's default system allocator for its operations. The #[global_allocator]
attribute is used to specify MyAllocator
as the global allocator for the entire program.
Remember, the Global Allocator is like the store clerk handling out the materials. In this case, MyAllocator
is our store clerk, and it's using the existing system allocator, System
, to get its job done. If the system allocator is like the warehouse in the back, MyAllocator
is the friendly face at the counter, helping to manage your needs.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is Rust's Global Allocator?
Rust's Global Allocator is a system component that oversees the allocation and deallocation of memory in a Rust program. It is defined at the program level and is used for dynamic memory allocation.
How do I define a Global Allocator in Rust?
You define a Global Allocator in Rust by creating a struct that implements the GlobalAlloc
trait, and then using the #[global_allocator]
attribute to specify your struct as the Global Allocator for your program.
Can I use the system allocator within my custom allocator?
Yes, you can use the system allocator within your custom allocator. The system allocator can be accessed with System.alloc(layout)
for allocation and System.dealloc(ptr, layout)
for deallocation.
How does memory allocation work in Rust?
In Rust, memory allocation is handled by the Global Allocator. When your program needs memory, it makes a request to the Global Allocator, which then provides the requested memory.
Why is memory management important in Rust?
Memory management is crucial in Rust because it ensures efficient use of system resources. By managing memory properly, you can prevent memory leaks and other performance issues in your Rust programs.