Rust Modules and Packages
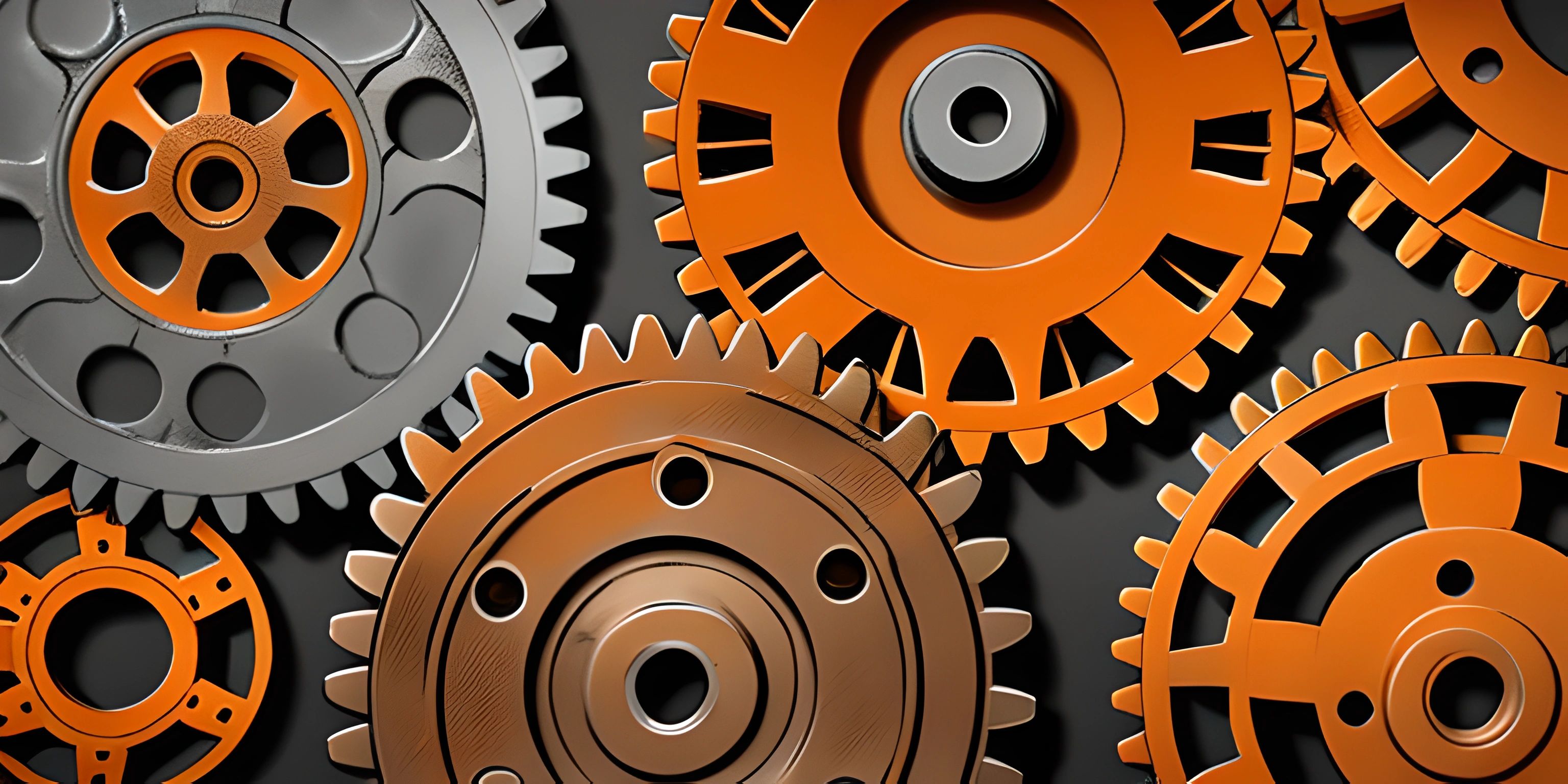
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Organizing code in Rust can be a breeze once you get the hang of modules and packages. Let's dive into these concepts and learn how to manage your Rust code effectively.
Rust Modules
In Rust, a module is a way to group related functionality together. Modules help you keep your code organized and maintainable by providing a clear structure and separation of concerns.
Creating a Module
To create a module, simply use the mod
keyword followed by the module name. Inside the curly braces, you can write all the functions, structures, and other code that belong to the module. Here's an example:
mod math { pub fn add(x: i32, y: i32) -> i32 { x + y } pub fn subtract(x: i32, y: i32) -> i32 { x - y } }
This creates a math
module with two public functions, add
and subtract
. The pub
keyword makes these functions accessible from outside the module.
Using a Module
To use a function or other item from a module, you can use the ::
operator. Here's how you can use the add
function from the math
module:
fn main() { let result = math::add(2, 3); println!("2 + 3 = {}", result); }
Rust Packages
A Rust package is a collection of one or more crates that are distributed together. A crate is a compilation unit that includes your Rust code along with its dependencies.
Creating a Package
When you create a new Rust project using cargo new
, you're actually creating a new package. The package has a Cargo.toml
file, which contains metadata about the package and its dependencies.
Here's an example of a simple Cargo.toml
file for a package named my_package
:
[package] name = "my_package" version = "0.1.0" edition = "2021" [dependencies]
Adding Dependencies
To add a dependency to your package, simply list it under the [dependencies]
section in the Cargo.toml
file. For example, to add the popular serde
crate, you would write:
[dependencies] serde = "1.0.130"
When you build your package, Cargo will download and compile the specified dependencies, making them available for use in your code.
Putting It All Together
Rust modules and packages work together to help you organize and manage your code effectively. By using modules to group related functionality and packages to manage dependencies, you can create clean, maintainable code that's easy to understand and modify.
Now that you have a solid understanding of Rust modules and packages, it's time to start organizing your own code! Don't forget to check out the Rust documentation for more information on these topics. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Structs and Traits (psst, it's free!).
FAQ
What are Rust modules and why are they important?
Rust modules are a way to organize and manage your code, making it more readable and maintainable. They allow you to group related functionality and types together, providing a clear structure and keeping your codebase clean. Modules help you avoid naming conflicts and make it easier to navigate and understand your code.
How do I create a Rust module?
Creating a Rust module is simple. In your source code, use the mod
keyword followed by the module name. Inside the curly braces {}
, you can define functions, structs, and other items related to that module. Here's an example:
mod my_module { pub fn greet() { println!("Hello from my_module!"); } }
What is a Rust package and how is it different from a module?
A Rust package is a collection of one or more related Rust modules, managed by the Cargo package manager. While a module helps organize related code within a single file or directory, a package groups multiple modules together and can be shared and distributed through the Cargo package registry. A package contains a Cargo.toml
file, which specifies dependencies, metadata, and build configuration.
How do I create a Rust package and add modules to it?
To create a Rust package, run the command cargo new package_name
. This will create a new directory with the given package name, containing a Cargo.toml
file and a src
directory with a default main.rs
or lib.rs
file. To add modules to the package, create a new file with the module name (e.g., my_module.rs
) in the src
directory, and use the mod
keyword in main.rs
or lib.rs
to import the module:
mod my_module; // Import the module from my_module.rs file fn main() { my_module::greet(); }
How can I control the visibility of items in a Rust module?
By default, items (functions, structs, etc.) inside a Rust module are private and can only be accessed within the same module. To make an item publicly accessible, use the pub
keyword before its definition:
mod my_module { pub fn public_function() { println!("This function is public!"); } fn private_function() { println!("This function is private!"); } }