Rust Vec Basics
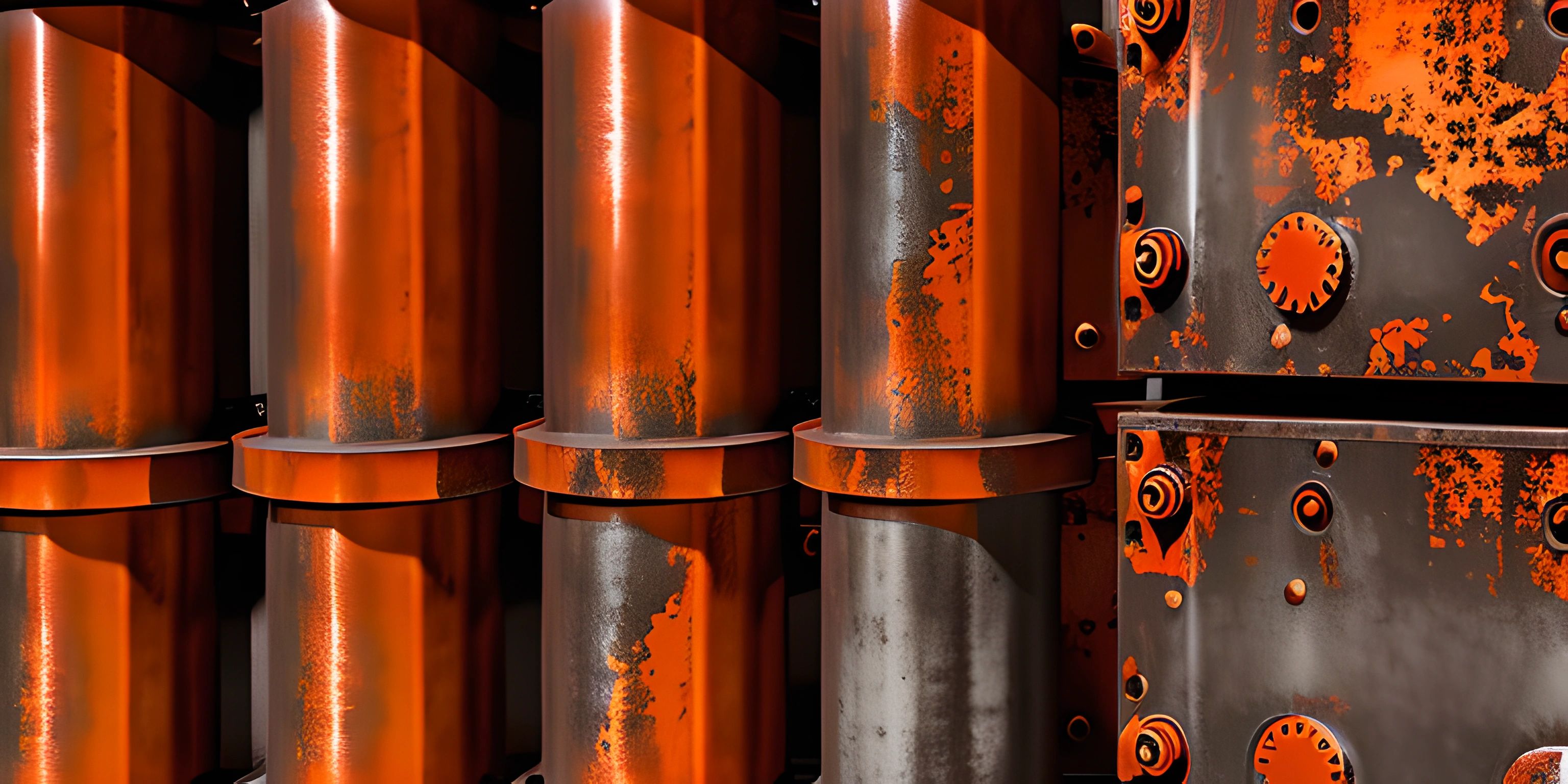
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Vectors are a vital part of the Rust programming language, providing you with a versatile and dynamic data structure that can grow and shrink as needed. Buckle up as we explore the basics of working with vectors in Rust and dive into some common operations and manipulations that will come in handy during your Rust programming journey!
Creating a Vector
In Rust, you can create a vector using the Vec
struct from the standard library. To create an empty vector, you can use the new
method:
let mut my_vec: Vec<i32> = Vec::new();
But hold on! What if you already have some values you want to store in the vector? No problem! You can use the vec!
macro instead:
let mut my_vec = vec![1, 2, 3, 4, 5];
Accessing Vector Elements
Now that we've created a vector, let's learn how to access its elements. You can use the familiar square bracket syntax to do this:
let first_element = my_vec[0]; // This will give us the first element (1) in the vector.
But beware! Using square brackets can lead to a panic if you try to access an index that doesn't exist. To avoid that, you can use the get
method, which returns an Option
:
let maybe_element = my_vec.get(100); // This will give us a None, instead of panicking.
Adding Elements to a Vector
Vectors can grow as needed, so adding new elements is a breeze. You can use the push
method to add elements to the end of a vector:
my_vec.push(6); // Now my_vec contains [1, 2, 3, 4, 5, 6].
Removing Elements from a Vector
Removing elements from a vector is just as straightforward. You can use the pop
method to remove the last element of the vector and return it as an Option
:
let last_element = my_vec.pop(); // This will give us Some(6) and leave my_vec with [1, 2, 3, 4, 5].
Iterating Over a Vector
To iterate over a vector, you can use a for
loop:
for element in &my_vec { println!("{}", element); }
This will print out each element in the vector on a separate line. If you want to modify the elements in the vector while iterating, you can use the iter_mut
method:
for element in my_vec.iter_mut() { *element += 1; }
This will mutate each element in the vector, adding 1 to it.
Conclusion
Vectors are an essential and versatile part of Rust programming, and mastering their basic operations and manipulations is crucial. With these skills, you can now manage dynamic collections of data with ease. Don't forget to explore other Rust collection types and continue your journey into the wonderful world of Rust programming!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Lifetimes (psst, it's free!).
FAQ
What is a Vec in Rust and why is it useful?
A Vec
in Rust is a dynamic array, which means it can grow or shrink in size during runtime. It's useful because it allows you to store and manage a collection of elements of the same type, providing efficient manipulation and access to the data. It's particularly useful for situations where you don't know the number of elements beforehand, or when the collection size might change over time.
How do you create a new Vec in Rust?
To create a new Vec
in Rust, you can use the vec!
macro or the Vec::new()
function. The vec!
macro allows you to create a Vec
with initial values, while Vec::new()
creates an empty Vec
. Here are examples of both methods:
let numbers = vec![1, 2, 3, 4, 5]; // Using the vec! macro let empty_numbers: Vec<i32> = Vec::new(); // Using the Vec::new() function
How can you add elements to a Vec in Rust?
To add elements to a Vec
in Rust, use the push()
method. This method appends an element to the end of the Vec
. Here's an example:
let mut numbers = vec![1, 2, 3]; numbers.push(4); // numbers now contains [1, 2, 3, 4]
How do you access elements in a Vec in Rust?
You can access elements in a Vec
using indexing or the get()
method. Indexing uses square brackets and can cause a panic if the index is out of bounds, while the get()
method returns an Option
, which is Some(value)
if the index is valid and None
if it's out of bounds. Here are examples of both methods:
let numbers = vec![1, 2, 3, 4, 5]; // Using indexing let first_number = numbers[0]; // first_number is 1 // Using the get() method let second_number = numbers.get(1); // second_number is Some(2)
How do you remove elements from a Vec in Rust?
You can remove elements from a Vec
using the remove()
method, which takes an index as an argument and removes the element at that position. Be aware that this method can cause a panic if the index is out of bounds. Here's an example:
let mut numbers = vec![1, 2, 3, 4, 5]; numbers.remove(1); // numbers now contains [1, 3, 4, 5]