Rust Hashmaps
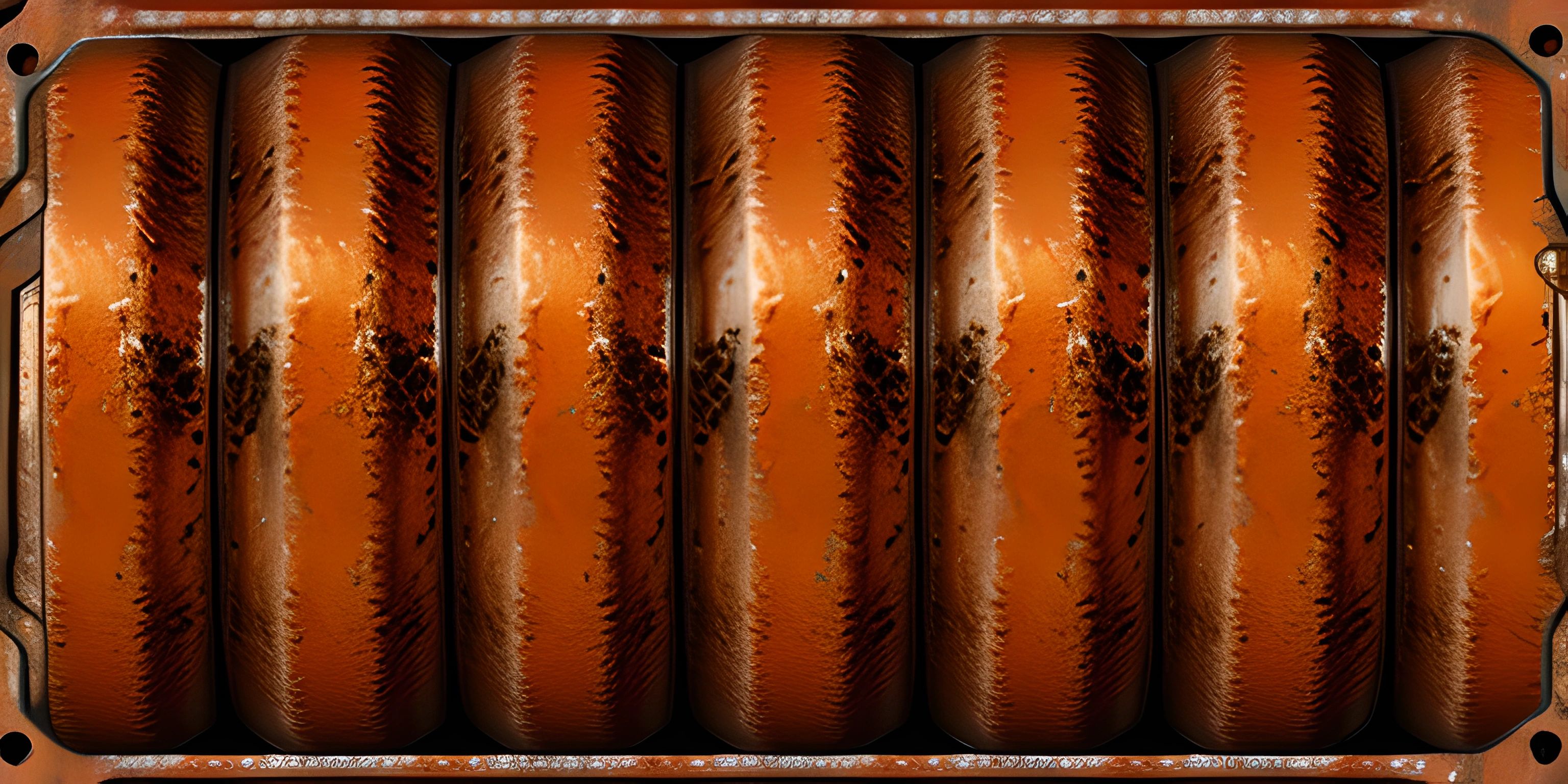
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you've ever wanted a data structure that lets you quickly find values based on unique keys, like a phone book or a dictionary, then you've come to the right place. In Rust, we have the wonderful hashmap to help us achieve just that.
What is a Hashmap?
A hashmap, also known as a hash table, is a data structure that allows you to store and retrieve values based on unique keys. It has complexities of O(1)
for insertion, deletion, and look-up operations, making it an efficient choice for large datasets.
Creating a Hashmap
Rust's standard library provides the HashMap
struct to help you create and manage hashmaps. To start using it, you'll first need to import it from the std::collections
module:
use std::collections::HashMap;
Now, let's create an empty hashmap:
let mut my_hashmap = HashMap::new();
Adding Data
To add data to your hashmap, use the insert()
method. It takes two arguments: the key and the value. Let's add some key-value pairs to our hashmap:
my_hashmap.insert("apple", 3); my_hashmap.insert("banana", 2); my_hashmap.insert("orange", 5);
Accessing Data
To access a value in the hashmap, you can use the get()
method and provide the key you're looking for:
let apple_count = my_hashmap.get("apple"); println!("There are {} apples.", apple_count.unwrap());
Note the use of unwrap()
here. This is because the get()
method returns an Option<&V>
. If the key is not present in the hashmap, get()
will return None
. Using unwrap()
allows us to get the actual value if it exists, but may panic if it's None
. To handle this safely, consider using pattern matching with match
or the if let
syntax.
Modifying Data
To modify the value associated with a key, you can use the entry()
method, followed by the or_insert()
method. This will return a mutable reference to the value, allowing you to update it:
let apple_count = my_hashmap.entry("apple").or_insert(0); *apple_count += 1;
Removing Data
To remove a key-value pair from the hashmap, use the remove()
method and provide the key:
my_hashmap.remove("apple");
Iterating Over a Hashmap
You can iterate over the key-value pairs in a hashmap using a for
loop:
for (key, value) in &my_hashmap { println!("{}: {}", key, value); }
Keep in mind that the order in which the key-value pairs are iterated is not guaranteed to be consistent, as hashmaps don't maintain any specific order.
Advantages of Hashmaps in Rust
Hashmaps offer several advantages in Rust, such as:
- Fast operations: With an average time complexity of
O(1)
for insertion, deletion, and look-up, hashmaps are an efficient choice for managing large datasets. - Memory safety: Rust's ownership system and borrowing rules help prevent common issues like dangling pointers and data races, ensuring safe concurrent access to hashmaps.
- Type safety: Rust enforces strict type checking, making sure you can only insert and retrieve values of the correct type.
That's a quick tour of hashmaps in Rust. Now you're ready to create your own phone book, dictionary, or any other key-value storage system with ease! Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is a hashmap in Rust?
A hashmap is a data structure in Rust, specifically a part of the std::collections
module, which allows you to store values with associated keys. It uses a hashing function to quickly look up values by their keys. Hashmaps provide efficient O(1) insertion, deletion, and lookup operations on average, making them a popular choice when fast access to data is crucial.
How do I create a hashmap in Rust?
To create a hashmap in Rust, you'll first need to import the HashMap
type from the std::collections
module. Then, you can create a new hashmap using the HashMap::new()
method. Here's an example:
use std::collections::HashMap; fn main() { let mut my_hashmap = HashMap::new(); my_hashmap.insert("key1", "value1"); my_hashmap.insert("key2", "value2"); println!("{:?}", my_hashmap); }
How do I access a value in a Rust hashmap using a key?
To access a value in a Rust hashmap, use the get
method and provide the key as an argument. The get
method returns an Option<&V>
type, where V
is the value type. This means that it returns Some(&value)
if the key is present in the hashmap, and None
if it is not. Here's an example:
use std::collections::HashMap; fn main() { let mut my_hashmap = HashMap::new(); my_hashmap.insert("key1", "value1"); my_hashmap.insert("key2", "value2"); match my_hashmap.get("key1") { Some(value) => println!("The value for key1 is: {}", value), None => println!("There is no value for key1"), } }
How do I remove a key-value pair from a hashmap in Rust?
You can remove a key-value pair from a hashmap in Rust using the remove
method. Provide the key as an argument, and if the key is present in the hashmap, the method will remove the associated key-value pair and return Some(value)
. If the key is not present, it will return None
. Here's an example:
use std::collections::HashMap; fn main() { let mut my_hashmap = HashMap::new(); my_hashmap.insert("key1", "value1"); my_hashmap.insert("key2", "value2"); match my_hashmap.remove("key1") { Some(value) => println!("Removed value: {}", value), None => println!("There is no value for key1"), } }
How can I iterate over the key-value pairs in a Rust hashmap?
To iterate over the key-value pairs in a Rust hashmap, you can use a for
loop along with the iter()
method. This method returns an iterator over the key-value pairs as tuples. Here's an example:
use std::collections::HashMap; fn main() { let mut my_hashmap = HashMap::new(); my_hashmap.insert("key1", "value1"); my_hashmap.insert("key2", "value2"); for (key, value) in my_hashmap.iter() { println!("{}: {}", key, value); } }