What is a System Call?
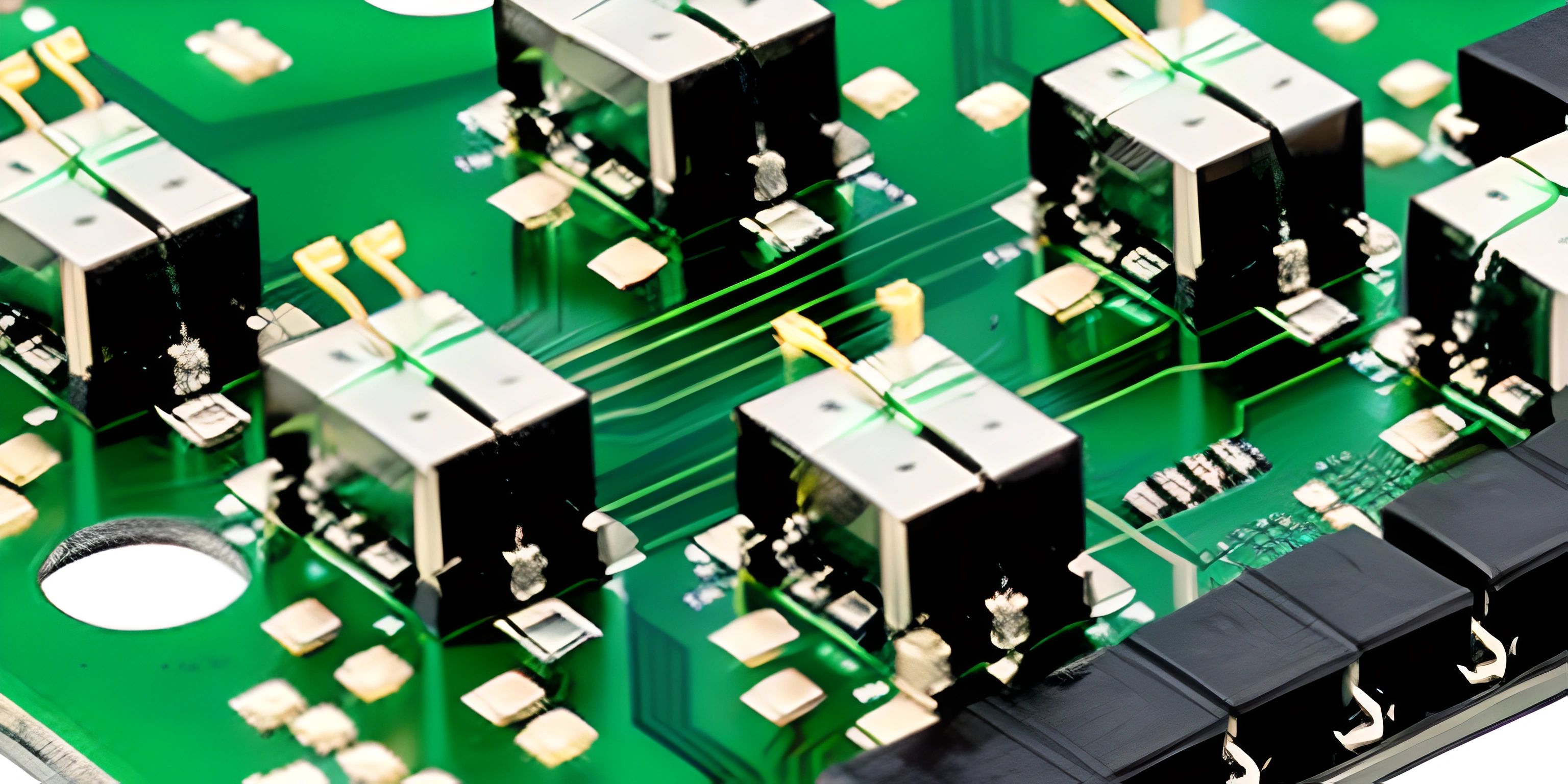
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
System calls are the bridge that connects user applications with the powerful, yet mysterious, world of the operating system. They're like a secret handshake between your program and the operating system (OS), allowing them to communicate and work together.
The Two Worlds: User Space and Kernel Space
To understand system calls, we must first explore the two separate realms within a computer system: user space and kernel space. In the land of computing, these two worlds coexist but are separated by a magical boundary.
User space is where all the applications we know and love reside, like browsers, text editors, and video games. The inhabitants of this realm have limited powers, as their actions are restricted by the operating system to ensure stability and security.
On the other side of the border lies kernel space, the realm of the operating system's kernel. The kernel is the heart of the OS, and it's responsible for managing resources, such as memory and processing power. In this domain, the kernel has unrestricted access to the hardware, wielding great powers to control the system.
Crossing the Boundary with System Calls
Although user applications have limited powers, they often need to request services provided by the kernel. This is where system calls come into play. System calls are the means by which user applications can request services from the kernel, effectively crossing the boundary between the two worlds.
For example, when a user application wants to read a file or send data over the internet, it cannot do so directly. Instead, it must ask the kernel for help. The application makes a system call, and the kernel performs the requested task on its behalf.
Here's a simple illustration using pseudocode:
open_file("important_document.txt")
In this example, the user application wants to open a file called "important_document.txt." It makes a system call to request the kernel to perform the file opening. The kernel then takes care of the nitty-gritty details, like locating the file on the disk and managing the file handles.
Types of System Calls
There are various types of system calls that cater to a wide range of services, such as file management, process control, and inter-process communication. Some common examples include:
open()
: Open a fileread()
: Read data from a filewrite()
: Write data to a filefork()
: Create a new processexec()
: Execute a new program
Different operating systems may offer different system calls, and the way they're implemented can vary. However, the general concept remains the same: user applications need system calls to request services from the kernel.
In Summary
System calls are the magical gateway that connects user applications with the powerful world of the operating system's kernel. By making system calls, applications can request a variety of services, such as reading files or managing processes. This essential communication mechanism ensures that our computer systems remain stable, secure, and efficient.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is a system call?
A system call is a way for programs to request services from the operating system, such as accessing files, creating new processes, or managing memory. They act as an interface between the user space (where programs run) and the kernel space (where the operating system manages resources and enforces security).
Why do we need system calls?
System calls are essential because they provide a controlled and secure way for programs to interact with the operating system and access system resources. Without system calls, programs would have unrestricted access to the system, leading to potential security risks and instability.
Can you provide an example of a system call?
Sure! A common example of a system call is the open
function in Unix-based systems, which is used to open a file. Here's a simple example in C:
#include <fcntl.h> #include <unistd.h> int main() { int file_descriptor; file_descriptor = open("example.txt", O_RDONLY); // Perform operations on the file using the file_descriptor close(file_descriptor); return 0; }
This code opens the file "example.txt" in read-only mode and then closes it after performing some operations.
How are system calls implemented?
System calls are typically implemented through software interrupts or special instructions in the processor's instruction set. When a program makes a system call, the processor switches to kernel mode, where the operating system handles the request and returns the result to the program.
What is the difference between library calls and system calls?
Library calls are functions provided by libraries, such as the C Standard Library, that can be used by programs to perform various tasks. System calls, on the other hand, are functions provided by the operating system that allow programs to access system resources and services. While some library functions may internally use system calls, not all library calls are system calls.