x86 Assembly NASM System Calls
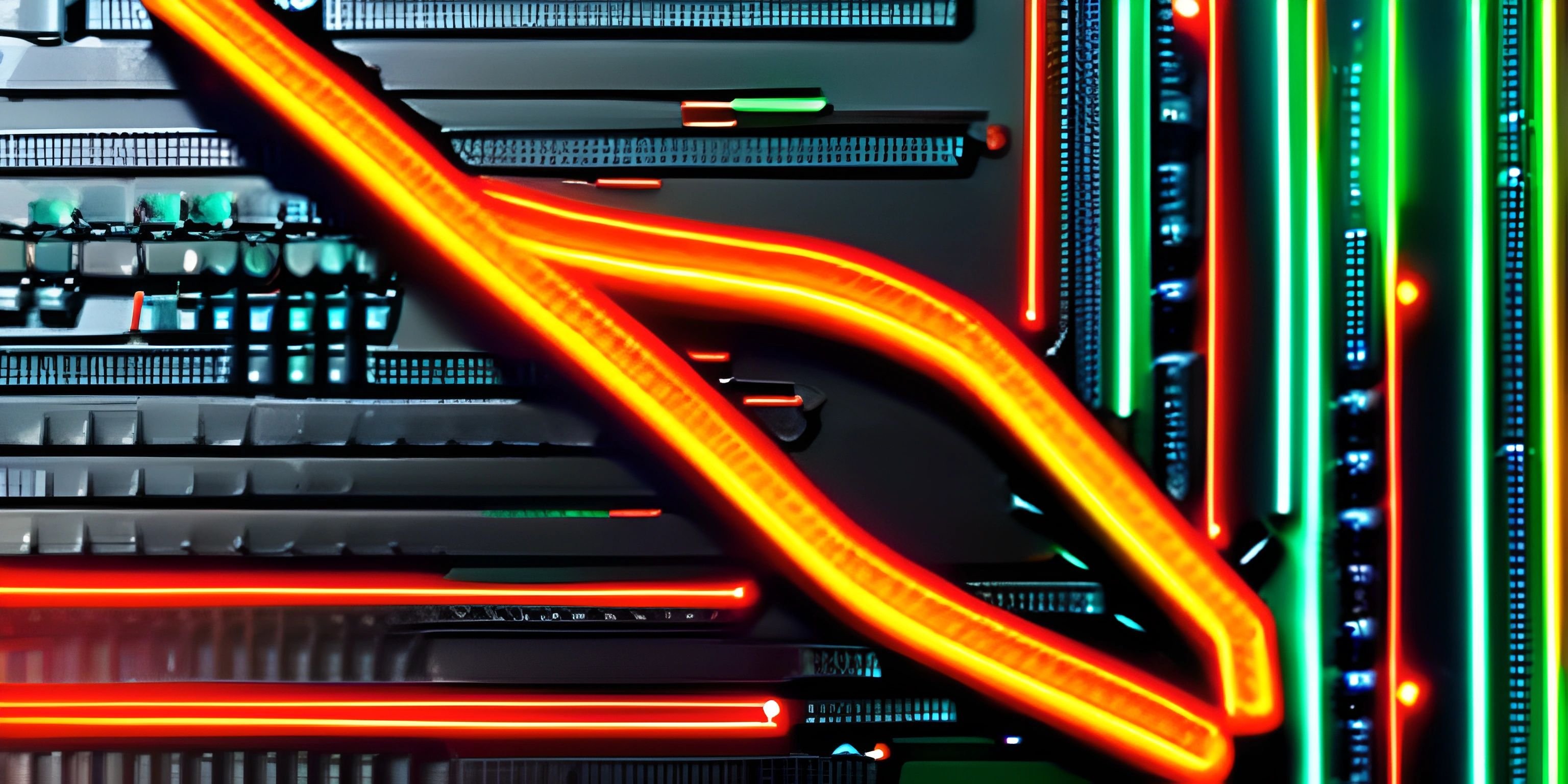
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of x86 assembly language programming, system calls play a crucial role in communicating with the operating system. They allow your program to request services, like console output, from the OS. In this article, we'll dive into the nuts and bolts of using system calls with NASM assembler.
What is a System Call?
A system call is a request made by a program to the operating system for a specific service. When your program needs to perform an action it can't do on its own, like printing text to the console, it relies on a system call to ask the OS for help.
NASM Assembler
NASM is a popular assembler for x86 assembly language, known for its flexibility and compatibility with various platforms. It provides a simple and clean syntax, making it easier to write and understand assembly code.
Making a System Call
To make a system call in x86 assembly, you'll need to follow three steps:
- Set up the registers with appropriate values.
- Execute the
int 0x80
instruction to trigger the system call. - Handle the return value, if necessary.
Let's go through these steps in more detail.
Step 1: Set up the registers
Before making a system call, you need to set up the registers with the required values. The most important register to set is the EAX register, which specifies the system call number. You'll also need to set other registers depending on the specific system call you're making.
For console output, we'll use the sys_write
system call (number 4). We'll also need to set the EBX register to the file descriptor (1 for stdout), the ECX register to the address of the data to be printed, and the EDX register to the length of the data.
Here's an example of setting up the registers for console output:
; Set up the registers for sys_write mov eax, 4 ; sys_write system call number mov ebx, 1 ; file descriptor for stdout mov ecx, msg ; address of the message to be printed mov edx, len ; length of the message
Step 2: Trigger the system call
Once the registers are set up, you can trigger the system call using the int 0x80
instruction. This instruction generates a software interrupt, signaling the OS to handle the system call.
; Trigger the system call int 0x80
Step 3: Handle the return value
After the system call is executed, the OS usually returns a value in the EAX register. For sys_write
, the return value is the number of bytes written. You can use this value as needed, or simply ignore it if it's not important for your program.
; Handle the return value (optional) mov [bytes_written], eax
Example: Printing Hello, World!
Here's a complete example of using a system call to print "Hello, World!" in x86 assembly with NASM:
section .data msg db 'Hello, World!', 0xA ; The message with a newline character len equ $ - msg ; Calculate the length of the message section .bss bytes_written resd 1 ; Reserve space for the return value section .text global _start _start: ; Set up the registers for sys_write mov eax, 4 ; sys_write system call number mov ebx, 1 ; file descriptor for stdout mov ecx, msg ; address of the message to be printed mov edx, len ; length of the message ; Trigger the system call int 0x80 ; Handle the return value (optional) mov [bytes_written], eax ; Exit the program using sys_exit system call mov eax, 1 ; sys_exit system call number xor ebx, ebx ; set ebx to 0 (exit status) int 0x80
Now you know how to use system calls for console output in x86 assembly language programming with NASM. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is a system call in x86 assembly language programming with NASM?
A system call is a method used by programs to request services from the operating system. In x86 assembly language programming with NASM, system calls are utilized to perform tasks such as console output, file handling, and process management. They provide an interface between the program and the operating system, allowing the programmer to access system-level functions.
How do I make a system call in x86 assembly using NASM?
To make a system call in x86 assembly using NASM, you need to follow these steps:
- Load the system call number into the EAX register.
- Load the required arguments for the system call into the appropriate registers (EBX, ECX, etc.).
- Issue the
int 0x80
instruction to trigger the system call. Here's an example of a basic system call for writing to the console:
mov eax, 4 ; System call number for write mov ebx, 1 ; File descriptor (stdout) mov ecx, msg ; Address of the message to write mov edx, msg_len ; Length of the message int 0x80 ; Trigger the system call
How can I find the system call numbers for x86 assembly programming with NASM?
System call numbers for x86 assembly programming with NASM can be found in the Linux kernel's source code, specifically in the unistd_32.h
header file. The system call numbers are defined as constants, which can be used in your assembly code. Alternatively, you can search online for a list of x86 system calls to find the specific call numbers you need.
How do I exit a program using a system call in x86 assembly with NASM?
To exit a program using a system call in x86 assembly with NASM, you can use the exit
system call. Here's an example of how to exit a program with a return code of 0 (success):
mov eax, 1 ; System call number for exit xor ebx, ebx ; Return code 0 (success) int 0x80 ; Trigger the system call
Can I make system calls in x86-64 assembly programming with NASM?
Yes, you can make system calls in x86-64 assembly programming with NASM as well. The process is similar to x86 assembly, but with some differences in register names and the system call instruction. Instead of int 0x80
, you'll use the syscall
instruction, and you'll use the RAX, RDI, RSI, and RDX registers for the system call number and arguments.