Arrays and Linked Lists: Usage and Performance
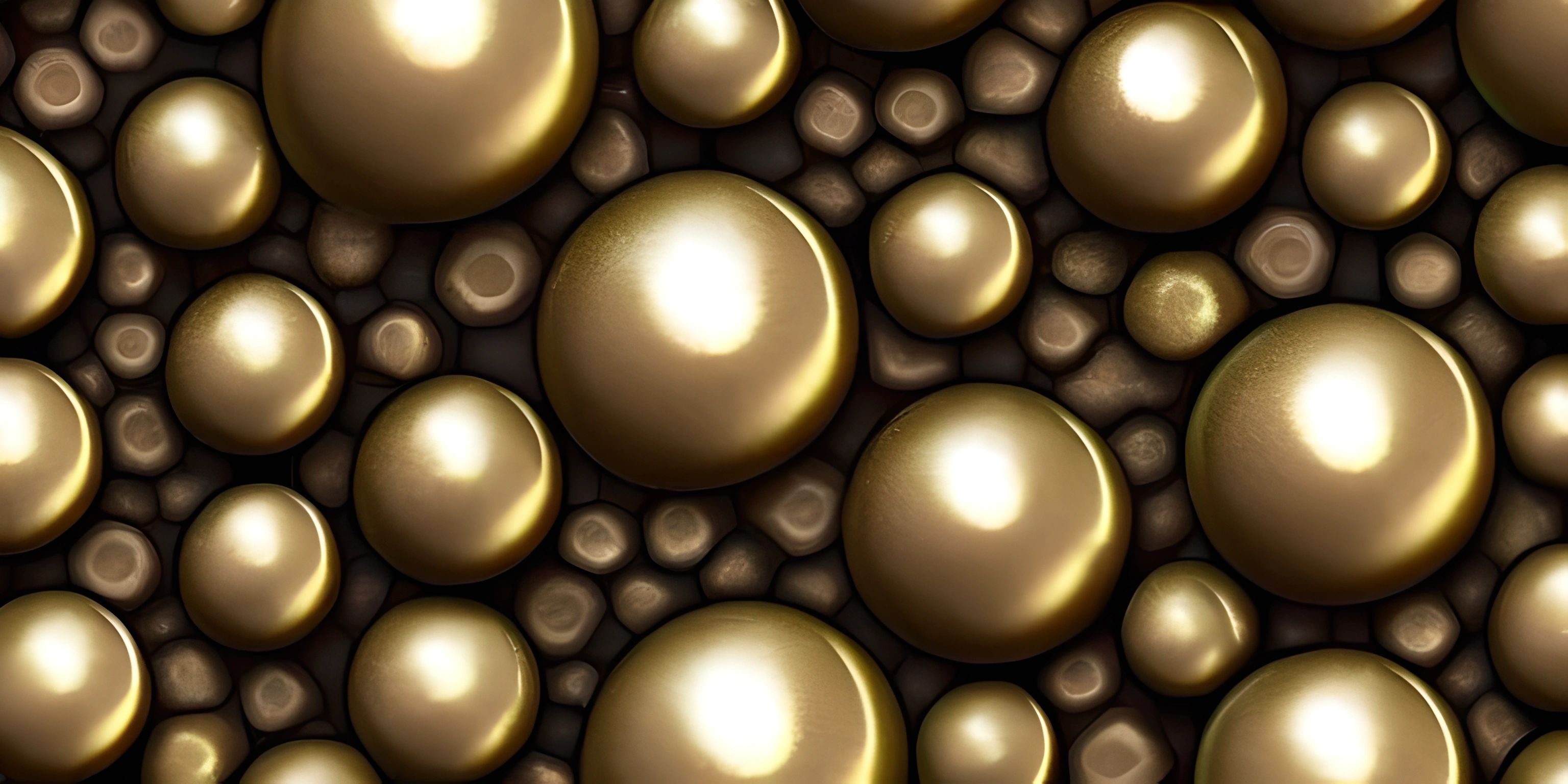
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When it comes to organizing data in programming, arrays and linked lists are two popular data structures that can help you achieve that goal. But how do you decide which one to use in a particular situation? In this article, we'll compare arrays and linked lists in terms of their usage and performance characteristics.
Arrays
An array is a contiguous block of memory that stores a fixed number of elements of the same data type. Their simplicity and constant-time access to elements make them a popular choice for many situations.
Performance Characteristics
- Access: Arrays provide constant-time access (O(1)) to elements by their index, which is a significant advantage if you need to read or modify data quickly.
- Insertion: Inserting an element into an array is an O(n) operation, as elements may need to be shifted to make room for the new element.
- Deletion: Deleting an element from an array is also an O(n) operation, as elements may need to be shifted to fill the gap left by the removed element.
- Memory: Arrays have a fixed size, so their memory usage is predictable. However, resizing an array can be an expensive operation.
Linked Lists
A linked list is a data structure made up of nodes, where each node holds a data element and a reference (or pointer) to the next node in the sequence. Linked lists offer dynamic sizing and efficient insertion and deletion operations, at the cost of slower access times.
Performance Characteristics
- Access: Accessing elements in a linked list takes O(n) time, as you may need to traverse the entire list to find the desired element.
- Insertion: Inserting an element into a linked list is an O(1) operation if you have a reference to the insertion point, which can be advantageous in certain situations.
- Deletion: Deleting an element from a linked list is also an O(1) operation if you have a reference to the element, making it faster than array deletions.
- Memory: Linked lists use more memory than arrays due to the storage of references in each node, but they can grow or shrink dynamically as needed.
When to Use Each
Here are some general guidelines to help you decide whether to use an array or a linked list:
-
Use arrays when:
- You need constant-time access to elements by their index.
- The size of the data set is fixed or predictable.
- Memory usage is a concern.
-
Use linked lists when:
- You require frequent insertion or deletion operations.
- The size of the data set is dynamic or unpredictable.
- Constant-time access to elements is not necessary.
Keep in mind that these guidelines are not set in stone, and the choice between arrays and linked lists will depend on the specific requirements of your program. As you gain experience, you'll develop a better understanding of which data structure is best for a given situation.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Palindromes (psst, it's free!).
FAQ
What are the key differences between arrays and linked lists?
Arrays and linked lists are two popular data structures with different characteristics:
- Memory allocation: Arrays are contiguous memory blocks while linked lists use scattered memory as their nodes can be located anywhere in memory.
- Access time: Arrays provide constant-time access (O(1)) to elements, whereas linked lists have a linear access time (O(n)) as they require traversal from the first element.
- Insertion and deletion: Linked lists support faster insertion and deletion operations (O(1)) when compared to arrays, which may require shifting elements (O(n)).
When should I choose an array over a linked list, or vice versa?
Arrays are preferable when:
- You need constant-time access to elements.
- The size of the data structure is fixed or known in advance.
- Memory usage is a concern, as arrays are more memory-efficient than linked lists. Linked lists are preferable when:
- You require frequent insertions and deletions.
- The size of the data structure is dynamic and unpredictable.
- You need a data structure that can be easily split or merged.
Can you provide an example of array and linked list implementation in Python?
Sure! Here's an example of implementing an array and a linked list in Python:
# Array implementation my_array = [1, 2, 3, 4, 5] # Linked list implementation class Node: def __init__(self, data): self.data = data self.next = None class LinkedList: def __init__(self): self.head = None my_linked_list = LinkedList() my_linked_list.head = Node(1) second_node = Node(2) third_node = Node(3) my_linked_list.head.next = second_node second_node.next = third_node
How can I perform common operations such as insertion and deletion on an array and a linked list?
Here are examples of insertion and deletion operations on an array and a linked list:
# ARRAY # Insertion at index 2 my_array.insert(2, 10) # [1, 2, 10, 3, 4, 5] # Deletion at index 3 del my_array[3] # [1, 2, 10, 4, 5] # LINKED LIST def insert_node_at_position(self, data, position): new_node = Node(data) if position == 0: new_node.next = self.head self.head = new_node else: current = self.head for _ in range(position - 1): if current is None: raise IndexError("Position out of range") current = current.next new_node.next = current.next current.next = new_node LinkedList.insert_node_at_position = insert_node_at_position my_linked_list.insert_node_at_position(10, 2) def delete_node_at_position(self, position): if self.head is None: raise ValueError("List is empty") if position == 0: self.head = self.head.next else: current = self.head for _ in range(position - 1): if current is None: raise IndexError("Position out of range") current = current.next if current.next is None: raise IndexError("Position out of range") current.next = current.next.next LinkedList.delete_node_at_position = delete_node_at_position my_linked_list.delete_node_at_position(3)
Why do linked lists have a linear access time?
Linked lists have a linear access time (O(n)) because each element, or node, in the list contains a reference to the next node. To access a specific node, you need to start at the head (first) node and traverse through the list until you reach the desired node. Unlike arrays, which have constant-time access due to their contiguous memory allocation, linked lists cannot directly access elements by their index.