Backtracking Algorithms
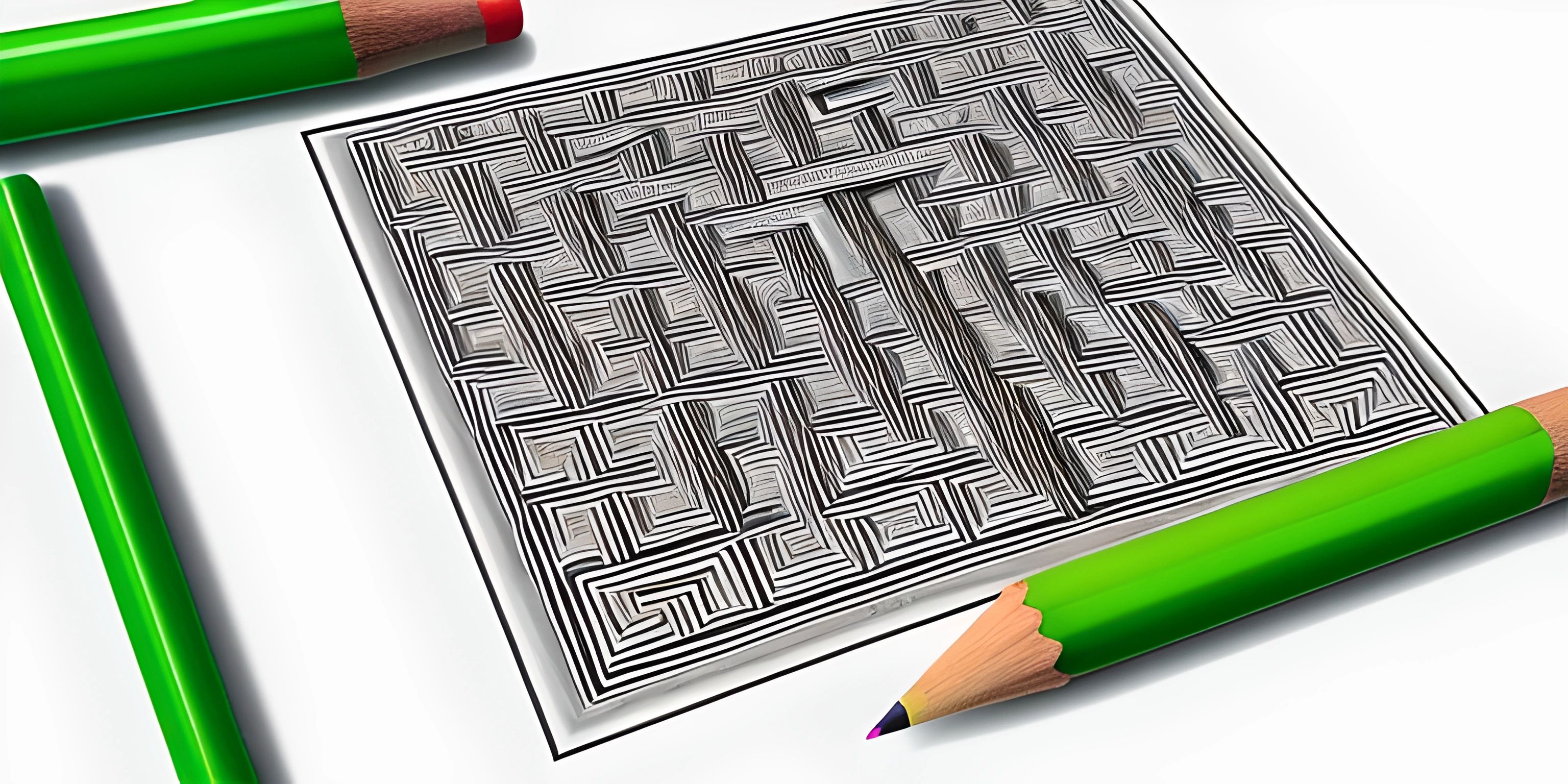
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Backtracking algorithms are like a Swiss Army knife in the world of problem-solving. They're versatile, powerful, and can get you out of some pretty tricky situations. So, strap in as we delve into the labyrinth of backtracking algorithms and unravel the mysteries of solving complex problems.
What is Backtracking?
Backtracking is a general algorithm for finding all (or some) solutions to a problem that incrementally builds candidates to the solutions and abandons a candidate ("backtracks") as soon as it determines that the candidate cannot be extended to a valid solution. In other words, it's like exploring a maze by choosing a path and going as far as you can. If you hit a dead end, you retrace your steps and try another path.
Backtracking is often applied to recursive algorithms and is a form of depth-first search.
How Does Backtracking Work?
Let's imagine you're trying to solve a maze. You start at the entrance and have to decide which direction to go. You could use backtracking to help you make this decision. Here's how it might work:
- Choose a direction.
- Move in that direction.
- If you've reached the exit, congratulations! You've solved the maze.
- If you've reached a dead end or a previously visited location, backtrack to the previous decision point.
- If there are more unexplored directions at the decision point, go back to step 1. Otherwise, continue backtracking.
This process continues until you either find the exit or exhaust all possible paths. In programming terms, this typically involves using recursion to explore each possible decision.
Example: Solving a Sudoku Puzzle
Let's apply backtracking to solve a classic problem: Sudoku. A Sudoku puzzle consists of a 9x9 grid, with some cells containing numbers. The objective is to fill in the remaining cells with numbers from 1 to 9, such that each row, column, and 3x3 subgrid contains each number exactly once.
Here's a high-level overview of how we might use backtracking to solve a Sudoku puzzle:
- Start at the first empty cell.
- Try placing a number (1-9) in the cell.
- If the number is valid (doesn't violate the rules of Sudoku), move on to the next empty cell.
- If you've filled in all the cells, you've solved the puzzle!
- If you run out of valid numbers for the current cell, backtrack to the previous cell and try a different number.
- Repeat steps 2-5 until the puzzle is solved or no more valid configurations can be found.
By systematically exploring all possible placements of numbers, the backtracking algorithm efficiently navigates through the search space of possible solutions.
Here's a simple Python example of a backtracking algorithm for solving a Sudoku puzzle:
def is_valid(puzzle, row, col, num): # Check if number is valid in row, column, and subgrid for i in range(9): if puzzle[row][i] == num or puzzle[i][col] == num: return False if puzzle[3 * (row // 3) + i // 3][3 * (col // 3) + i % 3] == num: return False return True def solve_sudoku(puzzle): row, col = find_empty_cell(puzzle) if row == -1 and col == -1: return True for num in range(1, 10): if is_valid(puzzle, row, col, num): puzzle[row][col] = num if solve_sudoku(puzzle): return True puzzle[row][col] = 0 return False def find_empty_cell(puzzle): for row in range(9): for col in range(9): if puzzle[row][col] == 0: return row, col return -1, -1
Though this is just one example, many other problems can be tackled using backtracking, such as the N-Queens problem, graph coloring, and more. So, next time you find yourself in a complex jam, remember the power of backtracking and step back to find the right path forward.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Recursion Intro (psst, it's free!).