Bash Introduction
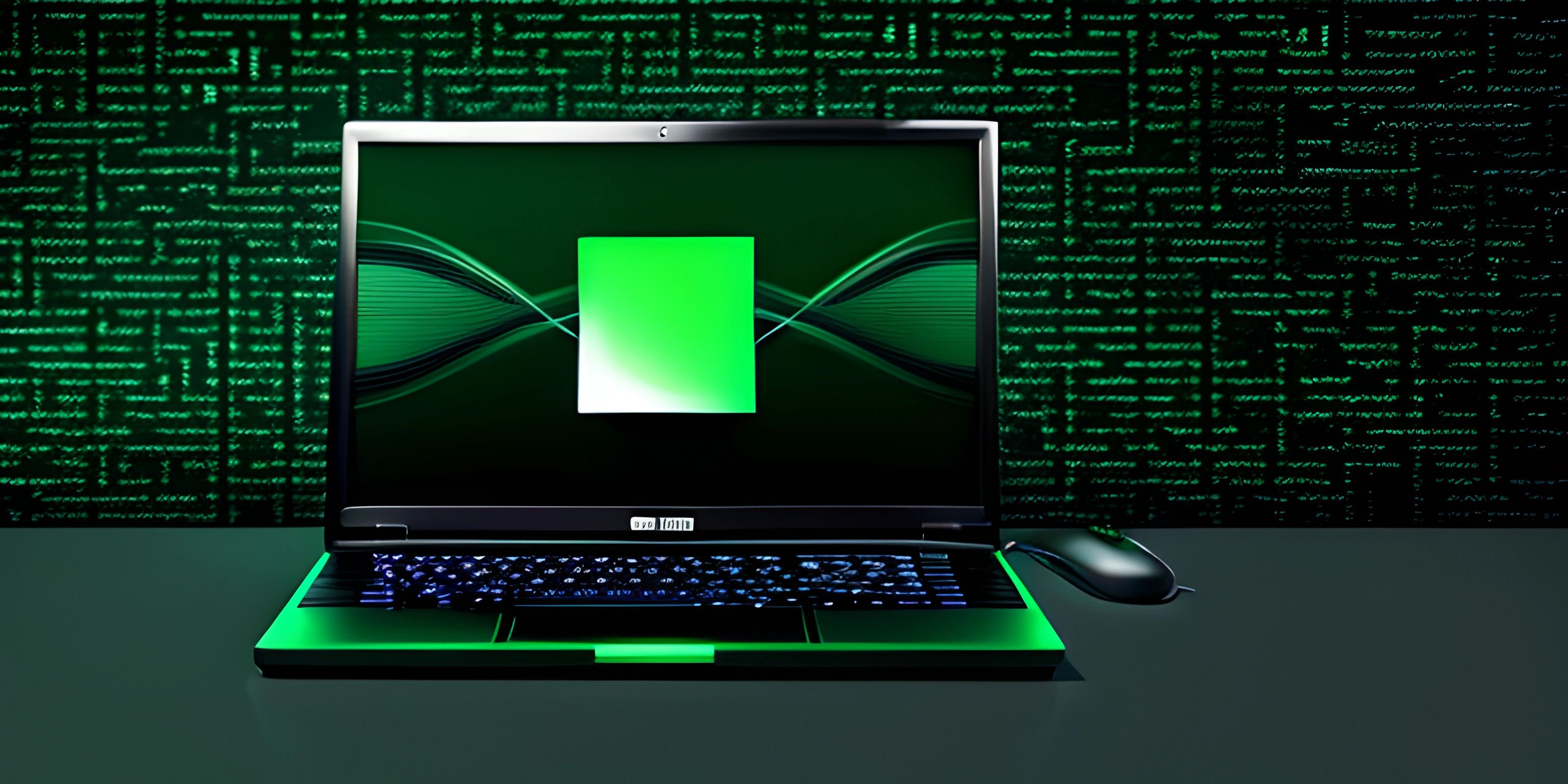
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Bash, short for Bourne-Again SHell, is a Unix shell that has become the default command-line interpreter for many Linux distributions and macOS. It's the successor to the original Bourne Shell (sh
) and offers a range of features that make it a powerful tool for scripting and everyday command-line use. Let's dive into some of the key features and concepts that make Bash stand out.
Features
Bash comes with a wealth of built-in features that make it a versatile tool for both users and developers. Here are a few notable ones:
Command History
Bash maintains a history of commands you've executed, allowing you to quickly recall and edit previous commands. This can be a huge time saver, especially when working on complex tasks or debugging scripts.
Command Aliasing
Bash allows you to create aliases, which are custom shortcuts for longer commands. For example, you might create an alias to quickly navigate to a frequently accessed directory or to run a common command with specific options.
Job Control
Bash supports robust job control, which enables you to run multiple processes concurrently, pause and resume them, and manage their execution in the background or foreground.
Scripting
Bash is not just a command interpreter; it's also a powerful scripting language that allows you to automate tasks, write complex programs, and create reusable functions.
Basic Syntax
Bash scripts are essentially sequences of commands executed line-by-line. You can create a Bash script by writing your commands in a plain text file and adding a shebang at the beginning of the file:
#!/bin/bash echo "Hello, world!"
This script will simply print "Hello, world!" to the console when executed. The shebang #!/bin/bash
tells the system to run the script using the Bash shell.
Variables
Bash supports variables, which store values that can be used and manipulated throughout your scripts. Variable assignment in Bash uses the =
operator without spaces around it:
#!/bin/bash greeting="Hello, world!" echo $greeting
In this example, we assign the string "Hello, world!" to the variable greeting
and then use the echo
command to print its value.
Control Structures
Bash supports various control structures, such as if
statements and for
loops, which allow you to add logic and flow control to your scripts:
#!/bin/bash for i in {1..5}; do if [ $((i % 2)) -eq 0 ]; then echo "Even: $i" else echo "Odd: $i" fi done
This script iterates through numbers 1 to 5 and prints whether each number is even or odd using a combination of for
loop and if
statement.
Functions
Bash allows you to create reusable functions, which can be called with arguments and can return values:
#!/bin/bash function greet() { local name="$1" echo "Hello, $name!" } greet "Alice" greet "Bob"
This script defines a function called greet
that takes one argument (a name) and prints a personalized greeting. The local
keyword is used to define a local variable within the function.
Bash is a versatile and powerful shell that provides a wide range of features and capabilities. With a good understanding of its syntax and features, you can harness the full potential of Bash to simplify your command-line tasks and create complex scripts.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Upgrading our Calculator (psst, it's free!).
FAQ
What is Bash and why is it important?
Bash, short for Bourne Again SHell, is a Unix shell and command-line interface for interacting with an operating system. It's important because it allows users to execute commands, navigate directories, manage files, and run scripts, providing a powerful way to interact with the system directly.
Can you provide a simple example of a Bash command?
Sure! A common Bash command is ls
, which lists the contents of a directory. Here's an example:
ls
Running this command in your terminal will display a list of files and directories in your current working directory.
How do I create and execute a Bash script?
To create a Bash script, follow these steps:
- Open a text editor and write your script starting with the shebang line:
#!/bin/bash
- Add your commands below the shebang line. For example, to create a simple script that prints "Hello, World!" to the console:
#!/bin/bash echo "Hello, World!"
- Save your file with the
.sh
extension, e.g.,hello.sh
. - Make your script executable by running the command:
chmod +x hello.sh
- Execute the script by typing
./
followed by the script name:
./hello.sh
What are some useful features of Bash for scripting?
Bash offers several features that make it a powerful scripting tool, including:
- Variables: Store and manipulate data in your script.
- Flow control: Use
if
,for
,while
, andcase
statements to control the flow of your script. - Functions: Create reusable blocks of code.
- Command substitution: Embed the output of one command into another.
- Pipes and redirection: Connect commands together and redirect input and output.
How can I learn more about Bash commands and scripting techniques?
To learn more about Bash, you can:
- Read the official Bash documentation: Bash Reference Manual
- Explore online tutorials and guides on Bash scripting.
- Practice writing and executing Bash scripts to gain hands-on experience.
- Join community forums and discussion groups to seek help and share knowledge with other Bash users.