L-Systems in Generative Art
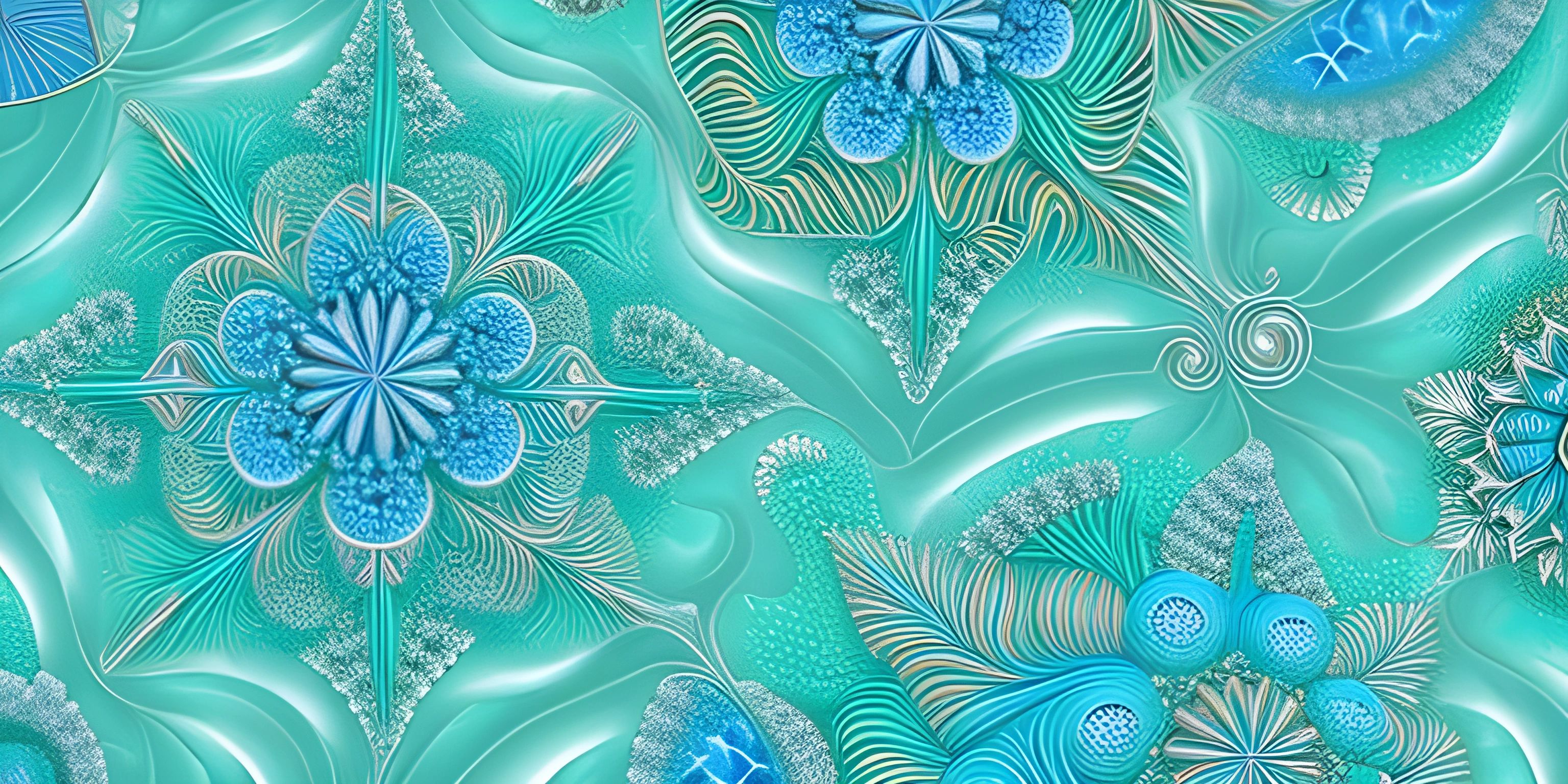
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine being able to create intricate, mesmerizing fractal patterns with just a few lines of code. Sounds like magic, right? Welcome to the world of L-Systems, or Lindenmayer Systems, a mathematical formalism that can generate complex and beautiful structures often seen in nature. These systems are a powerful tool for anyone interested in generative art.
What Are L-Systems?
L-Systems were originally conceived by the biologist Aristid Lindenmayer in 1968 to model the growth processes of plant cells. They are now widely used in computer graphics to create fractal-like images.
How Do L-Systems Work?
At their core, L-Systems use a set of rules (productions) to replace elements of a string with new elements. This iterative process continues for a specified number of steps or until a certain condition is met. Think of it as a botanical recipe: you start with an initial ingredient (axiom) and apply a series of transformations (productions) to grow your final structure.
Here’s a basic example:
- Axiom:
A
- Production Rule:
A -> AB
,B -> A
Starting with A
, the first iteration gives us AB
, the second gives us ABA
, and so forth.
Turtle Graphics
To turn these strings into images, we often use turtle graphics. Turtle graphics is a method for programming vector graphics using a relative cursor (the "turtle") that moves around the screen based on commands.
Here's a visual representation of how turtle graphics can interpret L-System strings:
- F: Move forward
- +: Turn right
- -: Turn left
Let's dive into an example to see how these elements come together.
Coding an L-System
We’ll use Python for our example because it has excellent libraries like turtle
that make drawing easy. First, install the turtle module if you haven't already:
pip install PythonTurtle
Now, let's write a simple L-System that generates a fractal tree.
import turtle # Define the L-System rules rules = { "F": "F+F-F-F+F" } # Generate the L-System string def l_system(axiom, rules, iterations): current_string = axiom for _ in range(iterations): next_string = "" for char in current_string: next_string += rules.get(char, char) current_string = next_string return current_string # Draw the L-System string using turtle graphics def draw_l_system(t, instructions, angle, distance): for cmd in instructions: if cmd == "F": t.forward(distance) elif cmd == "+": t.right(angle) elif cmd == "-": t.left(angle) # Main function def main(): axiom = "F" iterations = 4 angle = 90 distance = 10 l_string = l_system(axiom, rules, iterations) screen = turtle.Screen() t = turtle.Turtle() t.speed(0) draw_l_system(t, l_string, angle, distance) screen.mainloop() if __name__ == "__main__": main()
Here’s what’s happening:
- Define Rules: Our rule here is simple: replace
F
withF+F-F-F+F
. - Generate String: We start with
F
and apply the rules iteratively to generate the L-System string. - Draw String: Use turtle graphics to interpret the string and draw the pattern.
Customizing Your L-System
The beauty of L-Systems lies in their flexibility. By tweaking the rules, axiom, angle, and distance, you can create a vast array of patterns. Here are a few examples:
-
Dragon Curve
- Axiom:
FX
- Rules:
X -> X+YF+
,Y -> -FX-Y
- Angle: 90 degrees
- Axiom:
-
Koch Snowflake
- Axiom:
F++F++F
- Rules:
F -> F-F++F-F
- Angle: 60 degrees
- Axiom:
Try modifying the script with these new rules and see what you come up with!
Applications of L-Systems in Generative Art
L-Systems aren't just for creating fractal trees. They're a powerful tool for a variety of applications:
- Botanical Structures: Simulate the organic growth of plants, trees, and other flora.
- Fractal Patterns: Generate intricate, self-similar patterns.
- Urban Planning: Model city layouts and street designs.
- Textiles and Patterns: Create complex, repeating designs for fabrics.
Fun Fact: Fractals in Nature
Ever noticed the repeating patterns in ferns, snowflakes, or coastlines? These natural fractals can often be modeled using L-Systems. The ability to mimic such complexity makes L-Systems invaluable in scientific simulations and generative art.
Advanced Techniques
For those looking to push the boundaries, here are a few advanced techniques:
Context-Sensitive L-Systems
While basic L-Systems replace one symbol at a time, context-sensitive L-Systems consider neighboring symbols. This allows for more complex and realistic growth patterns.
Stochastic L-Systems
In stochastic L-Systems, production rules are chosen randomly from a set of rules. This introduces variability, making each iteration unique—ideal for organic and natural-looking patterns.
Parametric L-Systems
Parametric L-Systems add yet another layer by incorporating parameters that influence how rules are applied. This offers even greater control and flexibility in pattern generation.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Color Mandelbrot Set (psst, it's free!).
FAQ
What are L-Systems?
L-Systems, or Lindenmayer Systems, are a mathematical formalism used to model the growth processes of plants and generate fractal patterns. They use a set of rules to iteratively transform a string of symbols.
How do turtle graphics work with L-Systems?
Turtle graphics interpret L-System strings by moving a cursor (the "turtle") according to commands in the string, such as moving forward or turning. This allows the generation of complex, visual patterns from simple rules.
Can I use L-Systems in other programming languages?
Absolutely! While our example used Python, L-Systems can be implemented in any language that supports string manipulation and graphics. Languages like JavaScript, C++, and Processing are also popular choices.
What are some practical applications of L-Systems?
Beyond generative art, L-Systems are used in botanical simulations, urban planning, and even scientific modeling. They are versatile tools for generating complex, recursive patterns found in nature and artificial environments.
What are some advanced L-System techniques?
Advanced techniques include context-sensitive L-Systems, which consider neighboring symbols; stochastic L-Systems, which introduce randomness; and parametric L-Systems, which use parameters to influence rule application. These methods allow for more intricate and varied pattern generation.