Creating Interactive P5.js Sketches
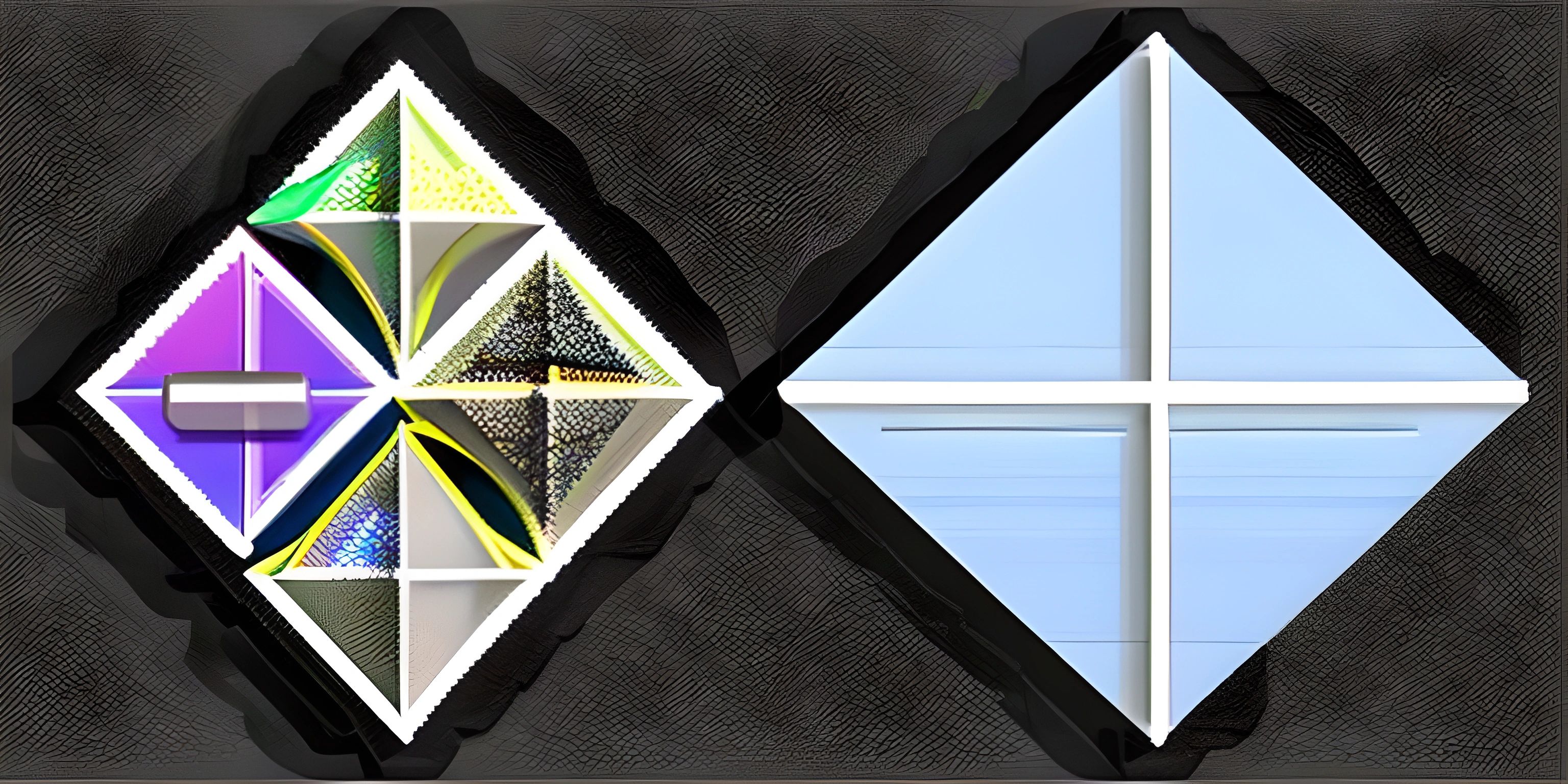
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you've ever dreamed of creating interactive digital art, then p5.js is here to make that dream come true. In this tutorial, we'll be exploring the fantastic world of p5.js and learning how to create interactive sketches that respond to user input. Grab a cup of your favorite creativity fuel, and let's dive right in!
What is p5.js?
p5.js is a JavaScript library that makes it easy to create interactive graphics and animations in the browser. It's based on the core principles of Processing, a popular programming environment for artists, designers, and creative coders. With p5.js, you can create interactive sketches using simple functions and a straightforward syntax.
Setting up a p5.js sketch
To get started, you'll need to set up a basic p5.js sketch using the setup()
and draw()
functions. The setup()
function runs once when the program starts, and the draw()
function runs continuously, creating a loop that updates the canvas with new visuals.
Here's a simple p5.js sketch that draws a circle on the canvas:
function setup() { createCanvas(600, 400); } function draw() { background(255); ellipse(300, 200, 100, 100); }
In this example, createCanvas(600, 400)
sets the size of the canvas to 600 pixels wide and 400 pixels high. The background(255)
function sets the background color to white, and ellipse(300, 200, 100, 100)
draws a circle at the center of the canvas.
Adding interactivity with user input
Now that we have a basic p5.js sketch, let's make it interactive by responding to user input. We can use p5.js's built-in functions for detecting mouse and keyboard events.
Mouse input
To make our circle follow the mouse cursor, we can replace the static coordinates (300, 200)
with the built-in variables mouseX
and mouseY
:
function draw() { background(255); ellipse(mouseX, mouseY, 100, 100); }
Now, the circle will follow the mouse cursor as you move it around the canvas. Magic!
Keyboard input
Let's add some keyboard interactivity to our sketch. We'll use the keyPressed()
function to detect when a key is pressed, and change the circle's color based on the key.
let circleColor = 0; function setup() { createCanvas(600, 400); } function draw() { background(255); fill(circleColor); ellipse(mouseX, mouseY, 100, 100); } function keyPressed() { if (key === "r" || key === "R") { circleColor = "red"; } else if (key === "g" || key === "G") { circleColor = "green"; } else if (key === "b" || key === "B") { circleColor = "blue"; } }
In this example, we've introduced a variable circleColor
to store the current color of the circle. The fill(circleColor)
function sets the color of the circle before it's drawn. In the keyPressed()
function, we check which key was pressed, and update the circleColor
variable accordingly.
Now, when you press the "R", "G", or "B" key, the circle's color will change to red, green, or blue, respectively.
Conclusion
Congratulations! You've just created an interactive p5.js sketch that responds to user input. Of course, this is just the tip of the iceberg when it comes to the possibilities of p5.js. You can create more complex animations, use built-in functions to generate shapes and patterns, and even integrate external data sources to create dynamic visualizations.
So, keep exploring, experimenting, and creating – the world of interactive art awaits!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).
FAQ
What is p5.js and why should I use it for creating interactive sketches?
P5.js is a JavaScript library that makes it easy to create graphics and interactive multimedia experiences for the web. It's perfect for creating interactive sketches because it simplifies the process of drawing on the screen, handling user input, and working with multimedia elements. With its easy-to-learn syntax and extensive documentation, p5.js is a great choice for beginners and experienced developers alike.
How can I get started with creating an interactive p5.js sketch?
To start creating an interactive p5.js sketch, you'll need to:
- Include the p5.js library in your HTML file using a script tag.
- Create a JavaScript file (e.g., sketch.js) and link it to your HTML file.
- Write the basic structure of a p5.js sketch, which includes the
setup()
anddraw()
functions. - Use p5.js functions to draw shapes, add colors, and handle user input for interactivity.
How can I add user input to my p5.js sketch?
To add user input to your p5.js sketch, you can use event listener functions such as mousePressed()
, keyPressed()
, and mouseMoved()
. These functions will be called when the corresponding event occurs, allowing you to modify your sketch based on user input. For example:
function mousePressed() { ellipse(mouseX, mouseY, 50, 50); }
This code will draw a circle at the mouse cursor's location whenever the mouse button is pressed.
Can I use p5.js to work with multimedia elements like images and sounds?
Yes, p5.js provides a set of functions to work with multimedia elements like images, sounds, and videos. For example, you can use the loadImage()
function to load an image file, and the image()
function to draw it on the canvas. Similarly, you can use the loadSound()
function to load a sound file, and the play()
function to play it. Check the p5.js documentation for more details and examples.
How can I make my p5.js sketch responsive to window resizing?
To make your p5.js sketch responsive to window resizing, you can use the windowResized()
function. This function is called automatically when the browser window is resized. Inside this function, you can use the resizeCanvas()
function to adjust the size of the canvas based on the new window dimensions. For example:
function windowResized() { resizeCanvas(windowWidth, windowHeight); }
This code will resize the canvas to fill the entire browser window when the window is resized.