Java File I/O
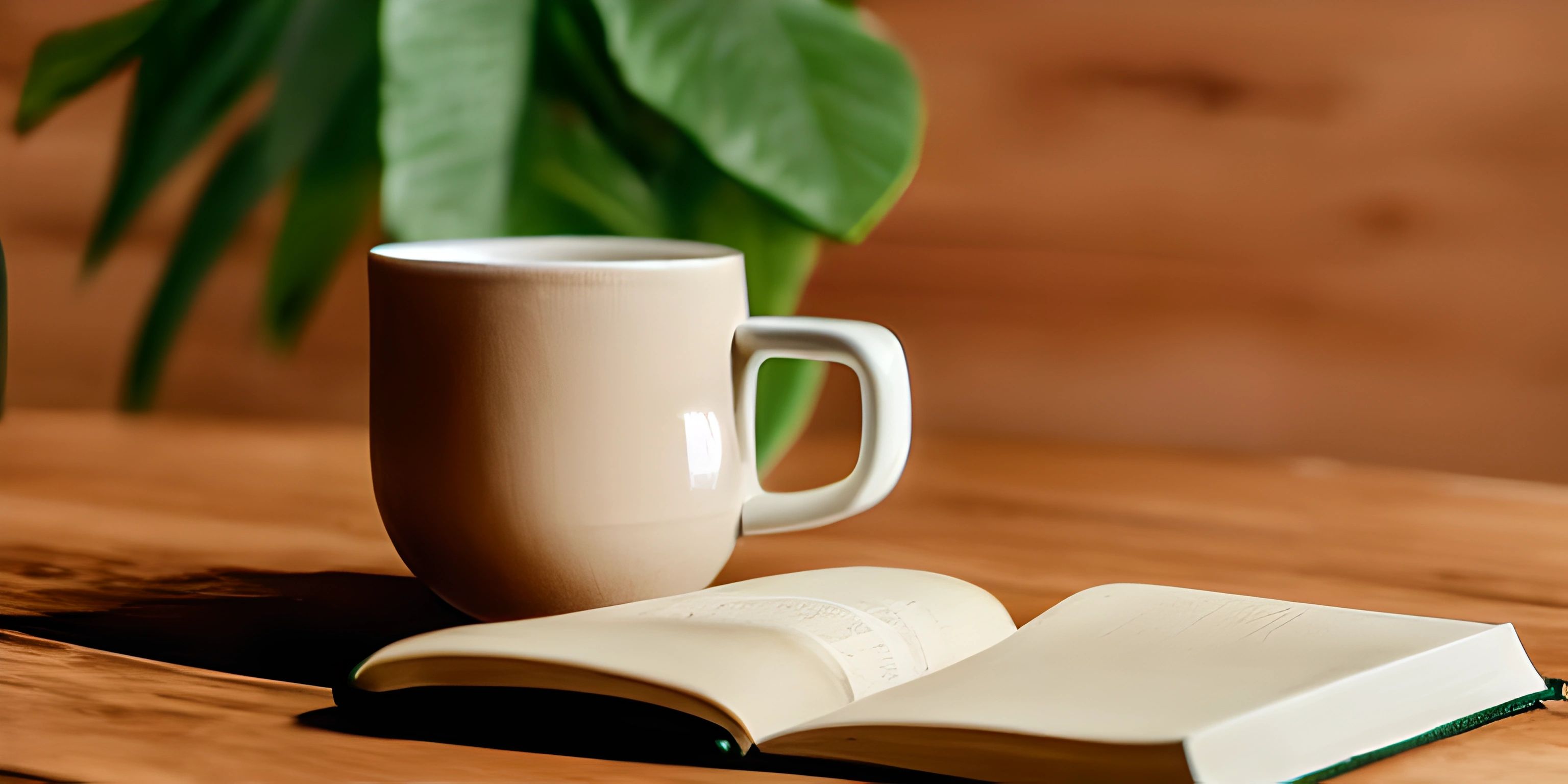
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Handling files in Java is an essential skill for any programmer. Whether you're reading data from a configuration file or writing logs to a file, understanding file I/O (input/output) is crucial. In this article, we'll cover the basics of reading and writing files in Java, using the FileReader, FileWriter, BufferedReader, and BufferedWriter classes.
Reading Files in Java
To read files in Java, you'll need to use the FileReader and BufferedReader classes. The FileReader class is responsible for getting the raw data from the file, while the BufferedReader class helps read the data more efficiently.
Here's an example of how to read a file in Java:
import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; public class ReadFile { public static void main(String[] args) { try { FileReader fileReader = new FileReader("example.txt"); BufferedReader bufferedReader = new BufferedReader(fileReader); String line; while ((line = bufferedReader.readLine()) != null) { System.out.println(line); } bufferedReader.close(); } catch (IOException e) { e.printStackTrace(); } } }
In this example, we first import the necessary classes. Then, we create a FileReader object by passing the file's name ("example.txt") to its constructor. After that, we create a BufferedReader object and pass the FileReader object to its constructor.
The readLine()
method of the BufferedReader class reads a line of text from the file. We use a while loop to read all the lines in the file until readLine()
returns null, indicating the end of the file. Finally, we close the BufferedReader using the close()
method.
Writing Files in Java
Writing files in Java is similar to reading files. We use the FileWriter and BufferedWriter classes to handle file writing. The FileWriter class writes data to the file, while the BufferedWriter class helps write data more efficiently.
Here's an example of how to write data to a file in Java:
import java.io.BufferedWriter; import java.io.FileWriter; import java.io.IOException; public class WriteFile { public static void main(String[] args) { try { FileWriter fileWriter = new FileWriter("output.txt"); BufferedWriter bufferedWriter = new BufferedWriter(fileWriter); String content = "This is an example of writing to a file in Java."; bufferedWriter.write(content); bufferedWriter.newLine(); bufferedWriter.close(); } catch (IOException e) { e.printStackTrace(); } } }
In this example, we first import the necessary classes. Then, we create a FileWriter object by passing the output file's name ("output.txt") to its constructor. After that, we create a BufferedWriter object and pass the FileWriter object to its constructor.
We then write a string content
to the file using the write()
method of the BufferedWriter class. The newLine()
method adds a newline character to the file. Finally, we close the BufferedWriter using the close()
method.
Conclusion
In this article, we've learned how to read and write files in Java using the FileReader, FileWriter, BufferedReader, and BufferedWriter classes. These classes make it simple and efficient to work with files in Java, making file I/O an essential skill for any Java programmer.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Basic Concepts (psst, it's free!).