Making AJAX Requests with the Fetch API in JavaScript
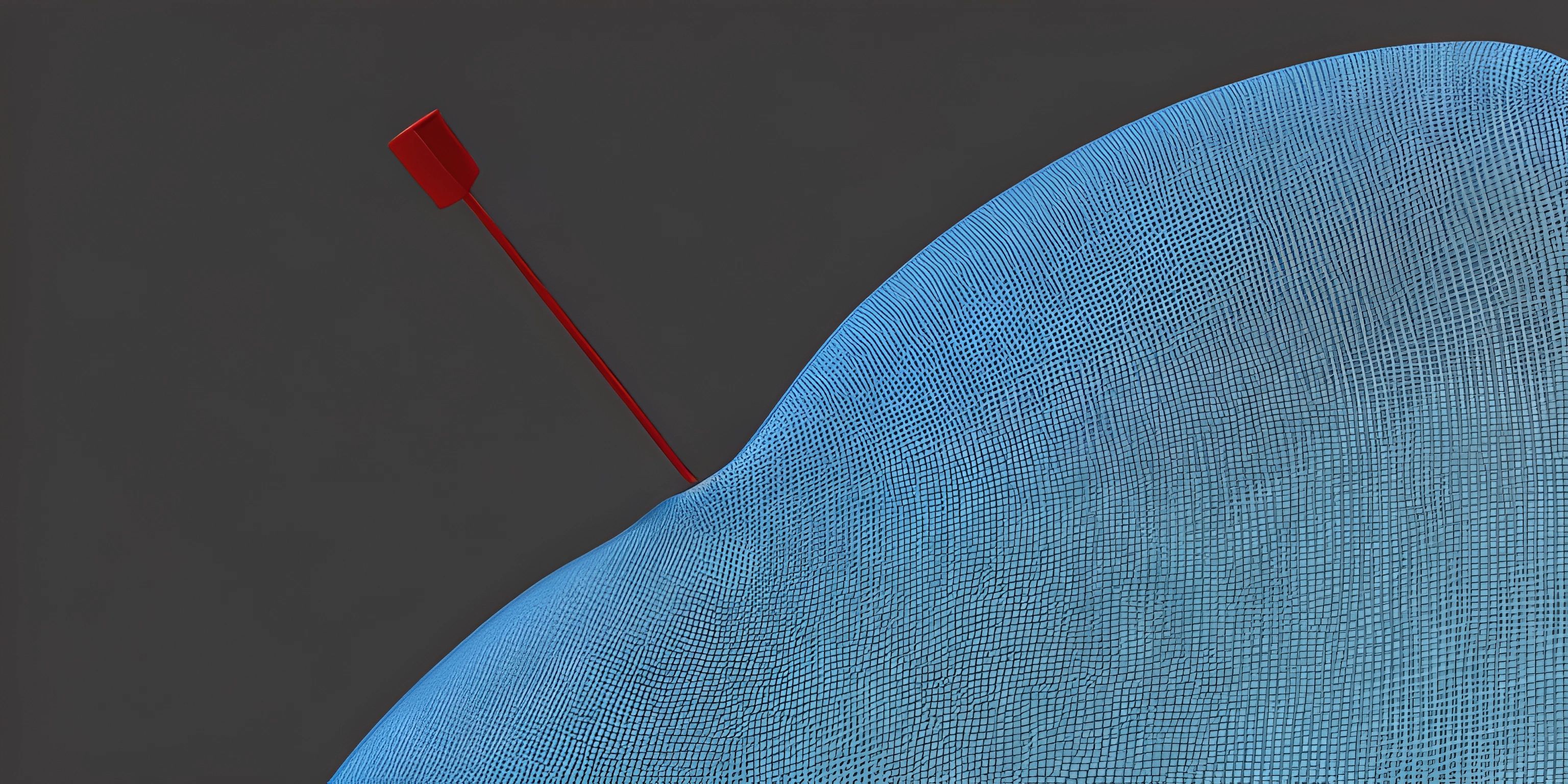
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In this article, we will learn how to make AJAX requests using the Fetch API in JavaScript. AJAX stands for Asynchronous JavaScript and XML. It allows you to send and receive data from a server without reloading the entire page, making your web applications more responsive and dynamic.
What is the Fetch API?
The Fetch API is a modern, native JavaScript API that provides an easy and flexible way to make AJAX requests. It is a more powerful and efficient alternative to the older XMLHttpRequest method, which was the traditional way of making AJAX requests.
Basic Syntax
The basic syntax of the Fetch API is as follows:
fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error("Error:", error));
Here's a breakdown of the syntax:
fetch(url)
initiates the request to the specified URL.- The
then()
method is used to handle the response. The response is often in JSON format, so we use theresponse.json()
method to convert it into a JavaScript object. - The
then()
method is called again to handle the data received from the server. In this example, we simply log the data to the console. - The
catch()
method is used to handle any errors that occur during the request.
Example: Fetching Data from an API
Let's see a practical example of using the Fetch API to fetch data from an API. In this example, we'll fetch a list of users from the JSONPlaceholder API.
fetch("https://jsonplaceholder.typicode.com/users") .then(response => response.json()) .then(data => { data.forEach(user => { console.log(`${user.name} (${user.email})`); }); }) .catch(error => console.error("Error:", error));
In this example, we fetch the list of users and log their names and email addresses to the console.
Conclusion
The Fetch API is a powerful and flexible way to make AJAX requests in JavaScript. It allows you to easily communicate with APIs and update your web pages without reloading, making your web applications more dynamic and responsive. Experiment with different APIs and use the Fetch API to enhance your web projects.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Async Rust (psst, it's free!).
FAQ
What is the Fetch API and why would I use it in JavaScript?
The Fetch API is a modern, powerful, and flexible approach to making AJAX requests in JavaScript. It is used for asynchronously fetching and updating data from a server, allowing you to update your web pages without reloading them. This results in a faster and smoother user experience.
How do I make a simple GET request using the Fetch API?
To make a simple GET request using the Fetch API, you can use the following code snippet: fetch("https://api.example.com/data") .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error)); This code makes a request to the specified URL and expects a JSON response. It then logs the received data to the console.
How do I make a POST request using the Fetch API?
To make a POST request using the Fetch API, you need to provide additional options to the fetch
function, like this:
const data = {
key1: "value1",
key2: "value2"
};
fetch("https://api.example.com/data", {
method: "POST",
headers: {
"Content-Type": "application/json"
},
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This code sends a POST request with a JSON payload to the specified URL and logs the received response.
How can I handle errors and exceptions with the Fetch API?
To handle errors and exceptions with the Fetch API, you can use the catch
method of the returned Promise:
fetch("https://api.example.com/data")
.then(response => {
if (!response.ok) {
throw new Error(HTTP error! Status: ${response.status}
);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error(error));
This code checks if the response status is OK (response.ok
), and if not, it throws an error. The catch
method of the Promise handles the error and logs it to the console.
Can I use the Fetch API with other HTTP methods like PUT, DELETE, etc.?
Yes, you can use the Fetch API with other HTTP methods like PUT, DELETE, and more by specifying the method
property in the options object passed to the fetch
function. For example:
fetch("https://api.example.com/data/123", {
method: "DELETE",
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This code sends a DELETE request to the specified URL, and logs the received response.