Generative Art with p5.js
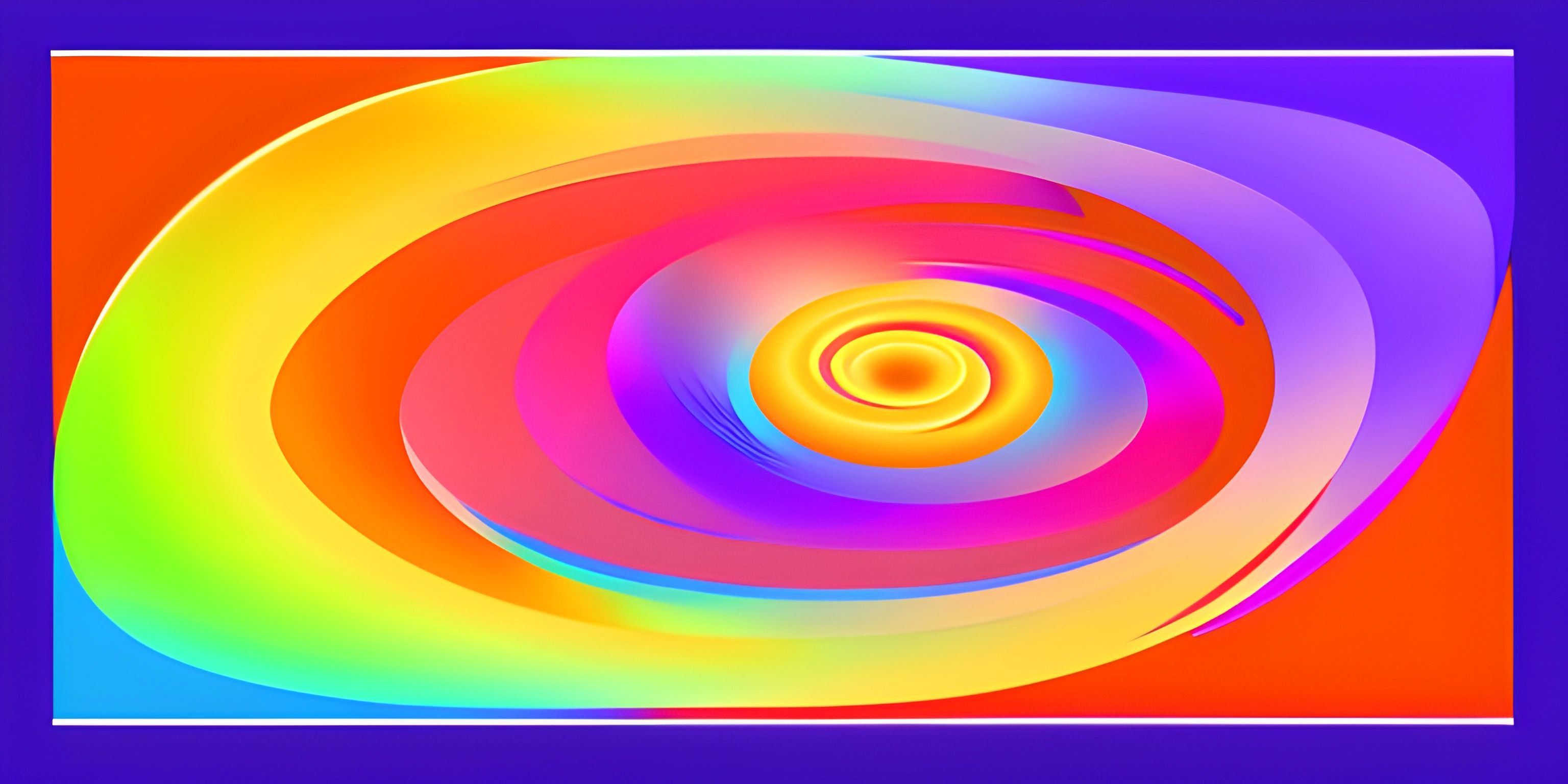
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Dive into the colorful world of generative art using p5.js, a powerful library designed to make coding graphics and creative works accessible to everyone. You'll soon discover that with a few lines of code, you can create mesmerizing visuals that come alive on your screen.
What is p5.js?
p5.js is a JavaScript library that makes it easy to create graphics and interactive art in the browser. It's designed to simplify the process of drawing on the web and make it accessible to everyone, even if you're new to programming. P5.js is built on the idea of Processing, a language and community focused on making code more accessible for artists, designers, and educators.
Setting up p5.js
To get started with p5.js, you'll need to include the library in your HTML file. You can either download the library from the official website or use a CDN link, like the one below:
<script src="https://cdnjs.cloudflare.com/ajax/libs/p5.js/1.4.0/p5.js"></script>
Add this script tag to your HTML file, and you're ready to begin your journey into the world of generative art!
Creating a Canvas
First, let's create a canvas where we'll be drawing our art. In p5.js, you can do this using the createCanvas()
function. This function is usually called inside the setup()
function, which runs once when the program starts:
function setup() { createCanvas(800, 600); }
This code creates a canvas with a width of 800 pixels and a height of 600 pixels. The canvas will automatically display in your browser, ready for you to draw on.
Drawing Shapes
Now that we have our canvas, let's start drawing some shapes. P5.js provides a variety of functions for drawing shapes, such as circle()
, rect()
, and triangle()
. Let's draw a simple circle on our canvas:
function draw() { circle(400, 300, 50); }
This code draws a circle with a center at (400, 300) and a radius of 50 pixels. The draw()
function is called repeatedly, creating a loop that allows us to continuously update our artwork.
Adding Colors
Generative art wouldn't be complete without a touch of color. P5.js offers a range of functions to set the colors of your shapes, like fill()
and stroke()
. To set the fill color of our circle, we can use the fill()
function:
function draw() { fill(255, 0, 0); // Red color circle(400, 300, 50); }
The fill()
function takes three arguments representing the red, green, and blue (RGB) values of the color. In this case, we've set it to red.
Making it Generative
To create generative art, we need to introduce some randomness into our code. P5.js provides a helpful function called random()
that generates random numbers. Let's use this function to create a circle with a random position and color:
function draw() { let x = random(width); let y = random(height); let r = random(255); let g = random(255); let b = random(255); fill(r, g, b); circle(x, y, 50); }
Now our circle will appear at a random position with a random color each time the draw()
function is called, making our art truly generative.
Wrapping Up
Congratulations, you've created your first generative artwork using p5.js! This is just the tip of the iceberg, as there are countless creative possibilities waiting to be explored. Start experimenting with different shapes, colors, and interactions to unleash your inner artist and bring your digital canvas to life.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Art with Code (psst, it's free!).
FAQ
What is p5.js and how can it be used in creating generative art?
p5.js is a JavaScript library that makes it easy to create graphics and interactive applications in the browser. It's inspired by Processing, a popular programming language for artists and designers. With p5.js, you can create generative art by using its various functions to draw shapes, colors, and patterns on a canvas, with the ability to include randomness or user interaction to produce unique and evolving visuals.
How do I set up a p5.js project?
To set up a p5.js project, follow these steps:
- Download the p5.js library from the official website (https://p5js.org/download/).
- Create an HTML file and include the p5.js library in the head section using a script tag:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <script src="p5.js"></script> </head> <body> </body> </html>
- Create a JavaScript file and link it to your HTML file using a script tag:
<head> <!-- ... --> <script src="p5.js"></script> <script src="your-script.js"></script> </head>
- In your JavaScript file, start writing your p5.js code by defining the
setup()
anddraw()
functions:
function setup() { createCanvas(800, 600); } function draw() { // Your generative art code goes here }
How can I incorporate randomness in my generative art with p5.js?
You can use the built-in random()
function in p5.js to generate random numbers within a specified range. For example, to create random colors, you can use random()
to set the red, green, and blue components of a color:
function draw() { let r = random(255); let g = random(255); let b = random(255); fill(r, g, b); // Draw shapes and patterns with this random color }
You can also use random()
to control the size, position, and other attributes of the shapes and patterns you create, resulting in unique and ever-changing generative art.
Can I use p5.js to create interactive generative art based on user input?
Yes, p5.js offers various functions to handle user input, such as mouse and keyboard events. You can use these functions to create interactive generative art that responds to the user's actions. For example, to draw a circle that follows the mouse cursor, you can use the built-in mouseX
and mouseY
variables to get the current mouse position:
function draw() { // Set circle color, size, etc. ellipse(mouseX, mouseY, 50, 50); }
You can also use functions like mousePressed()
, mouseReleased()
, keyPressed()
, and keyReleased()
to create more complex interactions in your generative art project.