MATLAB Control Flow
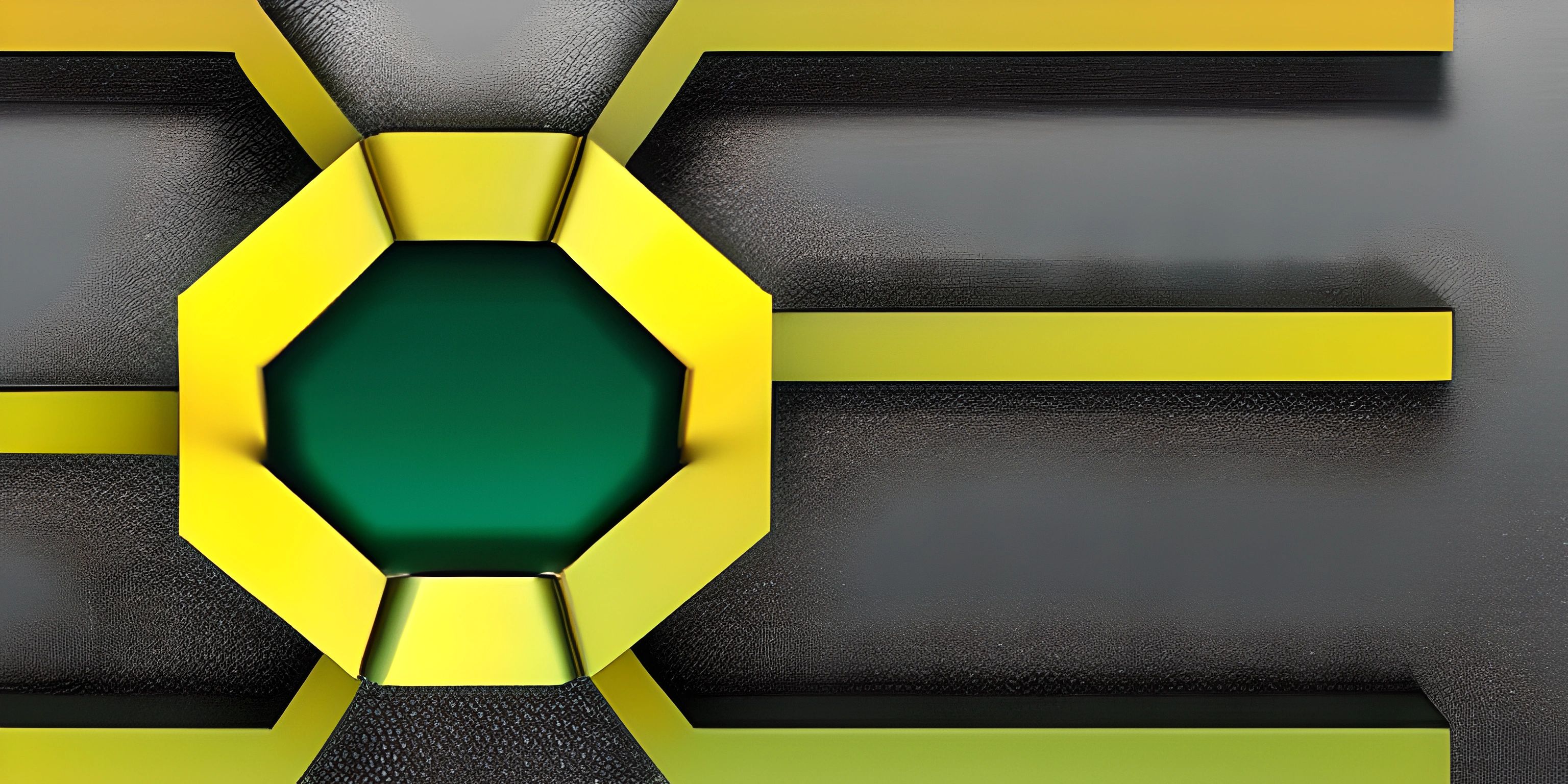
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In programming, control flow statements are essential for creating dynamic and efficient programs. Control flow statements allow you to change the behavior of your code based on certain conditions or to perform repeated actions. In this article, we'll discuss the most common control flow statements used in MATLAB, including if-else statements, loops, and switches.
If-else Statements
In MATLAB, if-else statements are used to make decisions based on conditions. The condition can be anything that evaluates to true or false. Let's take a look at the syntax and an example:
if condition % Code to execute if the condition is true else % Code to execute if the condition is false end
Here's an example:
x = 42; if x > 50 disp('x is greater than 50'); else disp('x is not greater than 50'); end
In this case, the output will be "x is not greater than 50".
You can also use "elseif" to add more conditions:
x = 42; if x > 50 disp('x is greater than 50'); elseif x == 42 disp('x is equal to 42'); else disp('x is neither greater than 50 nor equal to 42'); end
Loops
Loops in MATLAB are used to execute a block of code multiple times. There are two types of loops: for loops and while loops.
For Loops
A for loop iterates over a range of values. Here's the syntax and an example:
for variable = start:increment:end % Code to execute for each value in the range end
Example:
for i = 1:5 disp(['Iteration: ', num2str(i)]); end
Output:
Iteration: 1 Iteration: 2 Iteration: 3 Iteration: 4 Iteration: 5
While Loops
A while loop executes a block of code as long as a condition remains true. Here's the syntax and an example:
while condition % Code to execute while the condition is true end
Example:
counter = 0; while counter < 5 counter = counter + 1; disp(['Counter: ', num2str(counter)]); end
Output:
Counter: 1 Counter: 2 Counter: 3 Counter: 4 Counter: 5
Switch Statement
A switch statement is used to select one of many code blocks to be executed, based on the value of a variable or expression. Here's the syntax and an example:
switch variable case value1 % Code to execute if the variable equals value1 case value2 % Code to execute if the variable equals value2 otherwise % Code to execute if the variable does not equal any of the values end
Example:
fruit = 'apple'; switch fruit case 'banana' disp('The fruit is a banana'); case 'apple' disp('The fruit is an apple'); otherwise disp('Unknown fruit'); end
Output:
The fruit is an apple
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
How do I use if-else statements in MATLAB?
In MATLAB, if-else statements use the following syntax: if condition, then execute a block of code; else, execute another block of code. You can also use "elseif" to add more conditions. The blocks of code are executed based on the truth value of the conditions.
What are loops in MATLAB and how do I use them?
Loops in MATLAB are used to execute a block of code multiple times. There are two types of loops: for loops and while loops. A for loop iterates over a range of values, while a while loop executes a block of code as long as a condition remains true.
How do I use switch statements in MATLAB?
Switch statements in MATLAB are used to select one of many code blocks to be executed, based on the value of a variable or expression. You can use the "switch" keyword followed by "case" for each possible value and an "otherwise" block for when the variable does not match any of the values.