Introducing the Julia Programming Language
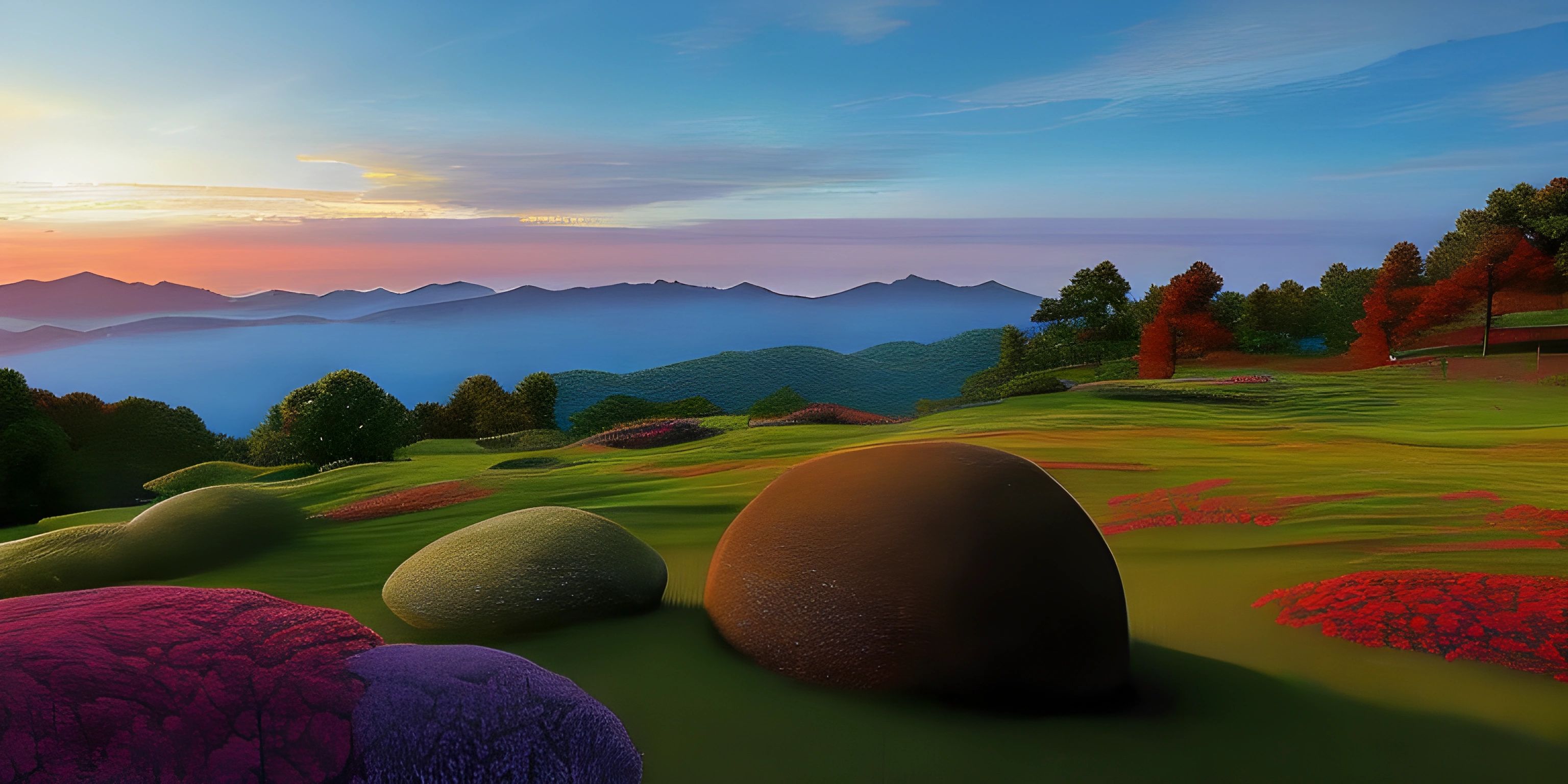
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
What if I told you there was a programming language that combined the ease of Python with the speed of C? You'd probably think I was pulling your leg, but guess what? Such a language exists, and it's called Julia! Known for its high performance and ease of use, Julia is making waves in the world of technical computing. So, buckle up as we take a joyride through the fascinating features of Julia!
What Makes Julia Special?
Julia is a high-level, high-performance programming language specifically designed for technical computing. But what does that mean exactly? Think of it as a sports car built to handle complex mathematical computations at breakneck speeds, yet easy enough for a novice to drive. Julia's syntax is both powerful and intuitive, making it an excellent choice for data scientists, engineers and researchers.
Speed and Performance
One of the standout features of Julia is its speed. Julia is designed from the ground up to be fast. It achieves this by compiling to efficient native code for multiple platforms via LLVM. This means that the code you write in Julia can often run as fast as, or even faster than, the equivalent code written in C or FORTRAN.
# Simple Julia code to calculate the sum of squares function sum_of_squares(n) total = 0 for i in 1:n total += i^2 end return total end println(sum_of_squares(10)) # Output: 385
Multiple Dispatch
Another unique feature of Julia is its support for multiple dispatch. In simple terms, this means that the function that gets executed is determined by the number and types of the arguments passed. This allows for more flexible and modular code.
# Example demonstrating multiple dispatch function area(shape::Circle) return π * shape.radius^2 end function area(shape::Square) return shape.side^2 end abstract type Shape end struct Circle <: Shape radius::Float64 end struct Square <: Shape side::Float64 end circle = Circle(5.0) square = Square(4.0) println(area(circle)) # Output: 78.53981633974483 println(area(square)) # Output: 16.0
Easy to Learn
If you're familiar with other high-level programming languages like Python or MATLAB, you'll find Julia's syntax to be straightforward and easy to pick up. This makes it an attractive option for beginners and experienced programmers alike.
# Julia's syntax is clean and easy to learn for i in 1:5 println("Hello, Julia!") end
Built for Technical Computing
Julia shines in technical computing, thanks to its extensive mathematical function library and its ability to easily call C and Fortran libraries. It's engineered to handle heavy computational tasks, making it a favorite among data scientists and engineers.
# Using built-in mathematical functions println(sqrt(16)) # Output: 4.0 println(sin(π/2)) # Output: 1.0 println(log(ℯ)) # Output: 1.0
Dynamic and Statically Typed
Julia gives you the best of both worlds with its dynamic and statically-typed nature. You can write code without declaring types, making it flexible, but you can also add type declarations to catch errors early and improve performance.
# Dynamic typing x = 42 println(x) # Static typing function add(a::Int, b::Int)::Int return a + b end println(add(10, 20)) # Output: 30
Ecosystem and Libraries
Julia has a rich ecosystem with a growing number of packages available for various tasks. From data manipulation and visualization to machine learning and optimization, Julia’s package ecosystem has you covered.
# Installing and using a package using Pkg Pkg.add("Plots") # Now you can use the Plots package for visualization using Plots plot([1, 2, 3, 4], [10, 20, 30, 40], title="Simple Plot")
Interoperability
One of the most fantastic things about Julia is its interoperability with other languages. You can easily call Python, C, and R code from Julia, making it a versatile tool in your programming toolkit.
# Calling Python code from Julia using PyCall py""" def hello(): print("Hello from Python!") """ py"hello()"
Community and Support
Julia has a vibrant and welcoming community. Whether you’re stuck on a problem or looking for ways to optimize your code, the Julia community is there to help. Plus, there are tons of online resources, tutorials, and documentation to get you up to speed.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
Is Julia suitable for web development?
While Julia is primarily designed for technical computing and high-performance tasks, it has packages like Genie.jl that support web development. However, for more traditional web development, languages like JavaScript or Python are more commonly used.
Can Julia replace Python?
Julia and Python serve different purposes and often complement each other. Julia excels in high-performance and technical computing tasks, whereas Python is more versatile for general-purpose programming and has a vast ecosystem of libraries for various applications.
How does Julia handle parallel computing?
Julia provides robust support for parallel and distributed computing. You can easily parallelize tasks using built-in macros like @parallel
and functions like pmap
. Distributed computing is also straightforward with Julia's Distributed
library.
Is Julia a good choice for machine learning?
Absolutely! Julia has several powerful libraries for machine learning, such as Flux.jl and MLJ.jl. Its high-performance nature makes it a strong contender for computationally intensive machine learning tasks.
How does Julia's multiple dispatch compare to object-oriented programming?
In object-oriented programming, methods are typically associated with objects. In Julia's multiple dispatch, methods are associated with function names and can be applied to different combinations of argument types. This allows for more modular and flexible code.