Introduction to the Logo Programming Language
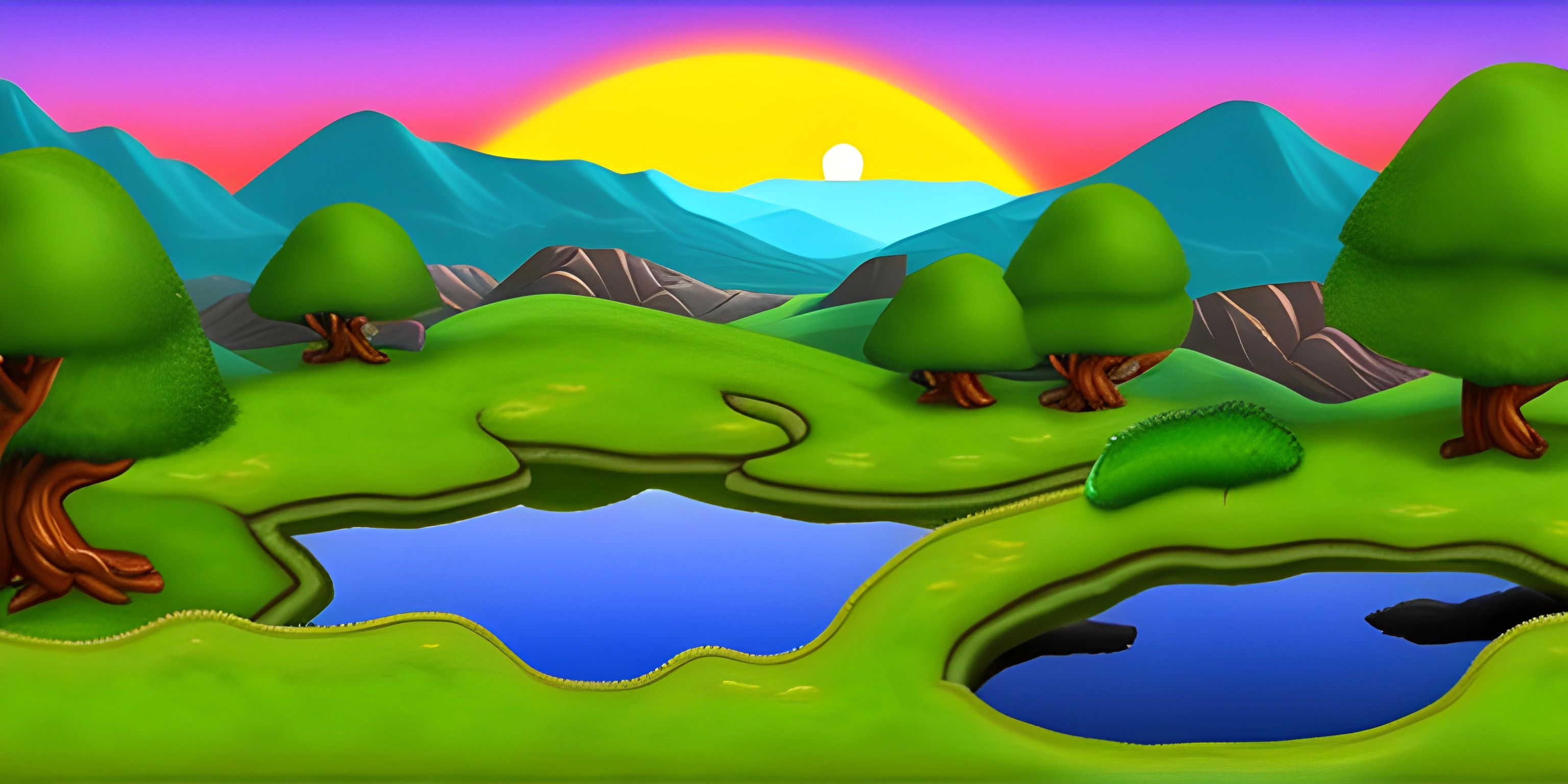
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the whimsical world of Logo! Imagine a friendly little turtle with a pen attached to its tail, drawing intricate patterns and shapes as it scuttles across the screen. Sounds fun, right? That's the essence of Logo, a programming language that's all about learning through creativity and visual feedback.
What is Logo?
Logo is a high-level programming language designed primarily for educational purposes. Developed in the late 1960s, Logo was originally intended to teach children the fundamentals of programming and mathematics. Its most distinctive feature is turtle graphics, a simple yet powerful way to create drawings and patterns by controlling a "turtle" that moves around the screen.
Turtle Graphics
Turtle graphics is the heart and soul of Logo. The turtle is an on-screen cursor that draws as it moves, allowing users to create complex designs with just a few simple commands. Imagine commanding a real turtle to draw shapes on the sand – that's pretty much what you're doing here!
Here's a simple example to get you started:
; Move the turtle forward by 100 units FORWARD 100 ; Turn the turtle 90 degrees to the right RIGHT 90 ; Move the turtle forward by another 100 units FORWARD 100
In this example, the turtle will draw an "L" shape. The FORWARD
command moves the turtle forward by a specified number of units, while the RIGHT
command turns the turtle to the right by a specified number of degrees.
Loops and Repetition
Logo makes it easy to create repetitive patterns using loops. For instance, if you wanted to draw a square, you could use the following code:
; Repeat the following commands 4 times REPEAT 4 [ FORWARD 100 RIGHT 90 ]
This code tells the turtle to move forward by 100 units and turn right by 90 degrees, repeating the process four times to complete a square. Notice how loops save you from writing repetitive code, making your life a whole lot easier!
Procedures and Functions
Just like in other programming languages, Logo allows you to define your own procedures (or functions). Procedures help you organize your code and reuse common patterns. Here's an example of defining a procedure to draw a square:
; Define a procedure to draw a square TO SQUARE :size REPEAT 4 [ FORWARD :size RIGHT 90 ] END ; Call the procedure to draw a square with a side length of 100 units SQUARE 100
In this example, the TO
keyword defines a new procedure called SQUARE
, which takes a parameter :size
. The REPEAT
loop inside the procedure uses this parameter to draw a square of the specified size. Finally, we call the SQUARE
procedure with a side length of 100 units.
Variables
Variables in Logo are used to store values that can be referenced and modified throughout your program. They're like containers that hold information. To define a variable, you use the MAKE
command:
; Define a variable called "sideLength" and set its value to 100 MAKE "sideLength 100 ; Use the variable in a command FORWARD :sideLength RIGHT 90 FORWARD :sideLength
In this example, we define a variable called sideLength
and set its value to 100. The :
symbol is used to reference the variable in subsequent commands.
Advanced Turtle Graphics
Once you're comfortable with the basics, you can start exploring more advanced turtle graphics techniques. For instance, you can create intricate patterns using nested loops and procedures. Here's an example of drawing a spiral:
; Define a procedure to draw a spiral TO SPIRAL :size :angle :increment IF :size > 200 [STOP] FORWARD :size RIGHT :angle SPIRAL :size + :increment :angle :increment END ; Call the procedure to draw a spiral SPIRAL 10 30 5
In this example, the SPIRAL
procedure draws a spiral by continuously moving forward and turning right, with the size of each step increasing incrementally. The IF
statement stops the procedure once the size exceeds 200 units.
Recursive Procedures
Logo also supports recursion, which is when a procedure calls itself. This can be a powerful tool for creating complex patterns and shapes. Here's an example of a recursive procedure to draw a tree:
; Define a procedure to draw a tree TO TREE :length IF :length < 10 [STOP] FORWARD :length RIGHT 30 TREE :length * 0.7 LEFT 60 TREE :length * 0.7 RIGHT 30 BACK :length END ; Call the procedure to draw a tree TREE 100
In this example, the TREE
procedure draws a tree by moving forward and branching off recursively. The IF
statement stops the recursion once the branch length is less than 10 units.
Conclusion
Logo is a delightful language that combines the joy of creativity with the rigor of programming. Its simplicity and visual feedback make it an excellent tool for beginners and educators alike. Whether you're drawing spirals, trees, or intricate patterns, Logo offers endless opportunities for exploration and learning.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is Logo programming language?
Logo is a high-level programming language designed primarily for educational purposes. It is known for its turtle graphics, which allows users to create drawings and patterns by controlling a "turtle" that moves around the screen.
How do turtle graphics work in Logo?
Turtle graphics involve controlling an on-screen cursor (the turtle) that draws as it moves. You can command the turtle to move forward, turn, and create complex designs with simple commands like FORWARD and RIGHT.
What are procedures in Logo?
Procedures in Logo are similar to functions in other programming languages. They help organize code and allow for the reuse of common patterns. Procedures are defined using the TO keyword and can take parameters.
Can you use variables in Logo?
Yes, Logo supports variables, which are used to store values that can be referenced and modified throughout your program. Variables are defined using the MAKE command and referenced using the : symbol.
What is recursion in Logo?
Recursion in Logo is when a procedure calls itself. It is a powerful tool for creating complex patterns and shapes. Recursive procedures can be used to draw structures like trees by repeatedly calling themselves with modified parameters.