Working with Files and Arrays in Node.js
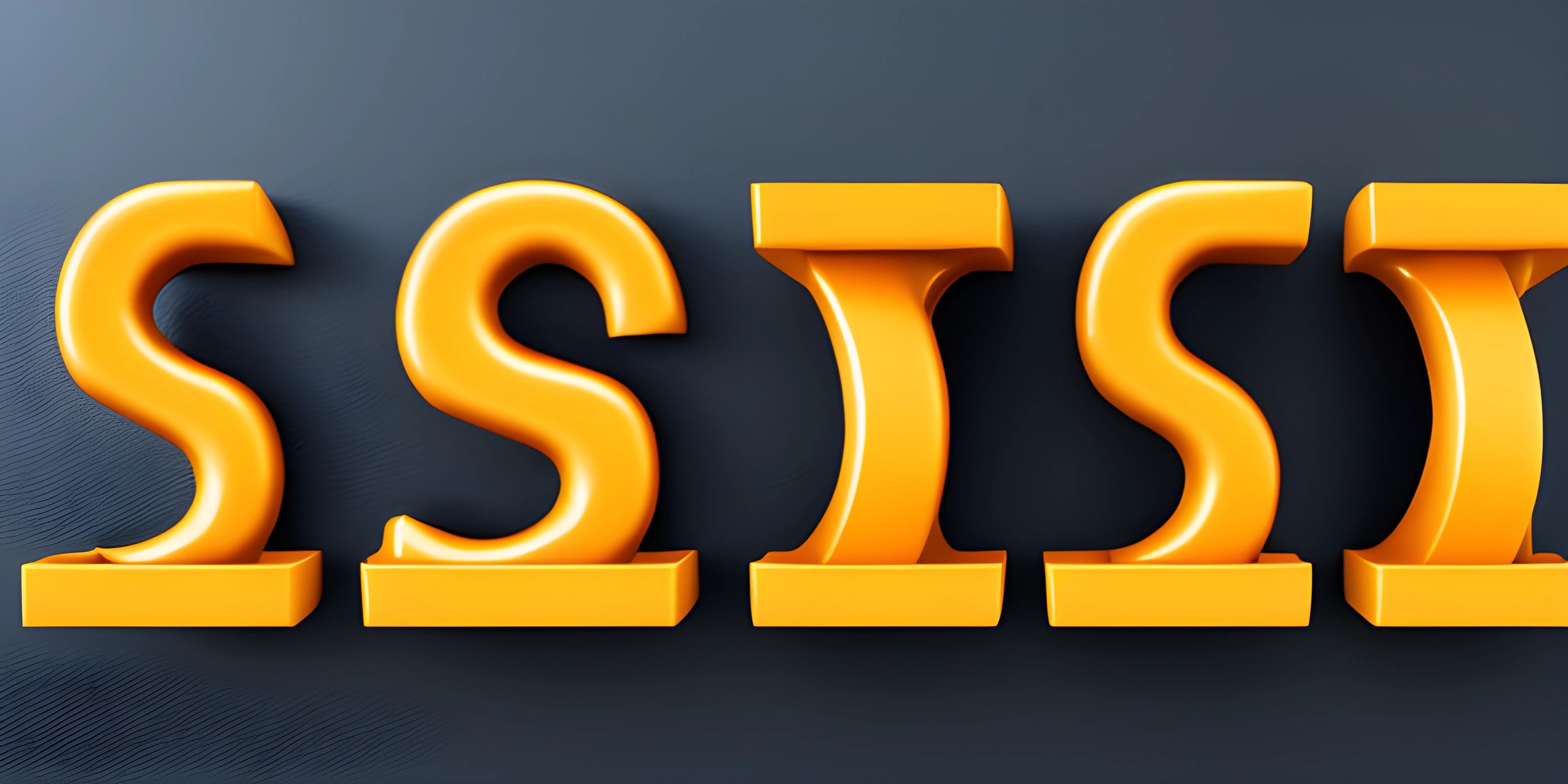
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Breaking code into smaller, simpler parts has always been a good principle to follow in programming. Today, we'll tackle a common scenario that involves reading a file, splitting its contents into an array, and then transforming those array elements. This scenario is often encountered when processing data files in Node.js, a popular JavaScript runtime.
Reading a File in Node.js
Node.js provides us with an inbuilt module called fs
(which stands for file system) to work with files on your computer. To read a file, we use the readFile
method provided by this module. This is how it looks in practice:
const fs = require("fs"); fs.readFile("example.txt", "utf8", (err, data) => { if (err) throw err; console.log(data); });
In this example, example.txt
is the file we're reading, and utf8
is the character encoding we're using. If the file is successfully read, its contents are logged to the console. If there's an error, it's thrown and the program stops executing.
Splitting a String into an Array
Once you've read a file, you might want to process its contents. Let's say our file contains a list of numbers separated by commas. We can use the split
method to turn this string into an array:
const array = data.split(",");
Now we have an array where each element is a string representing a number from our file.
Converting Strings into Numbers
The last piece of our puzzle involves converting these string numbers into actual numbers. We can achieve this using the map
method combined with the Number
function:
const numbers = array.map(Number);
Now, the numbers
array contains actual numbers that we can use in mathematical operations.
And voila! You've just learned how to read a file, split its contents into an array, and convert those strings into numbers using Node.js.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: File I/O (Reading) (psst, it's free!).
FAQ
How do I read a file in Node.js?
In Node.js, you can read a file using the fs
(file system) module's readFile
method. This method takes the name of the file and the character encoding as arguments, and a callback function to handle the file's contents or any potential error.
How do I split a string into an array in JavaScript?
In JavaScript, you can split a string into an array using the split
method. This method takes a separator as an argument and returns an array of substrings.
How do I convert strings into numbers in JavaScript?
In JavaScript, you can convert strings into numbers using the Number
function. If the string cannot be converted into a number, NaN
(Not a Number) is returned.
What is the `map` method in JavaScript?
The map
method in JavaScript creates a new array by calling a provided function on every element in the original array. It's commonly used to transform the elements of an array.
What is the `fs` module in Node.js?
The fs
module in Node.js is a core module that provides an API to interact with the file system. You can use it to read and write files, among other operations.