Introduction to the p5.js Setup Function
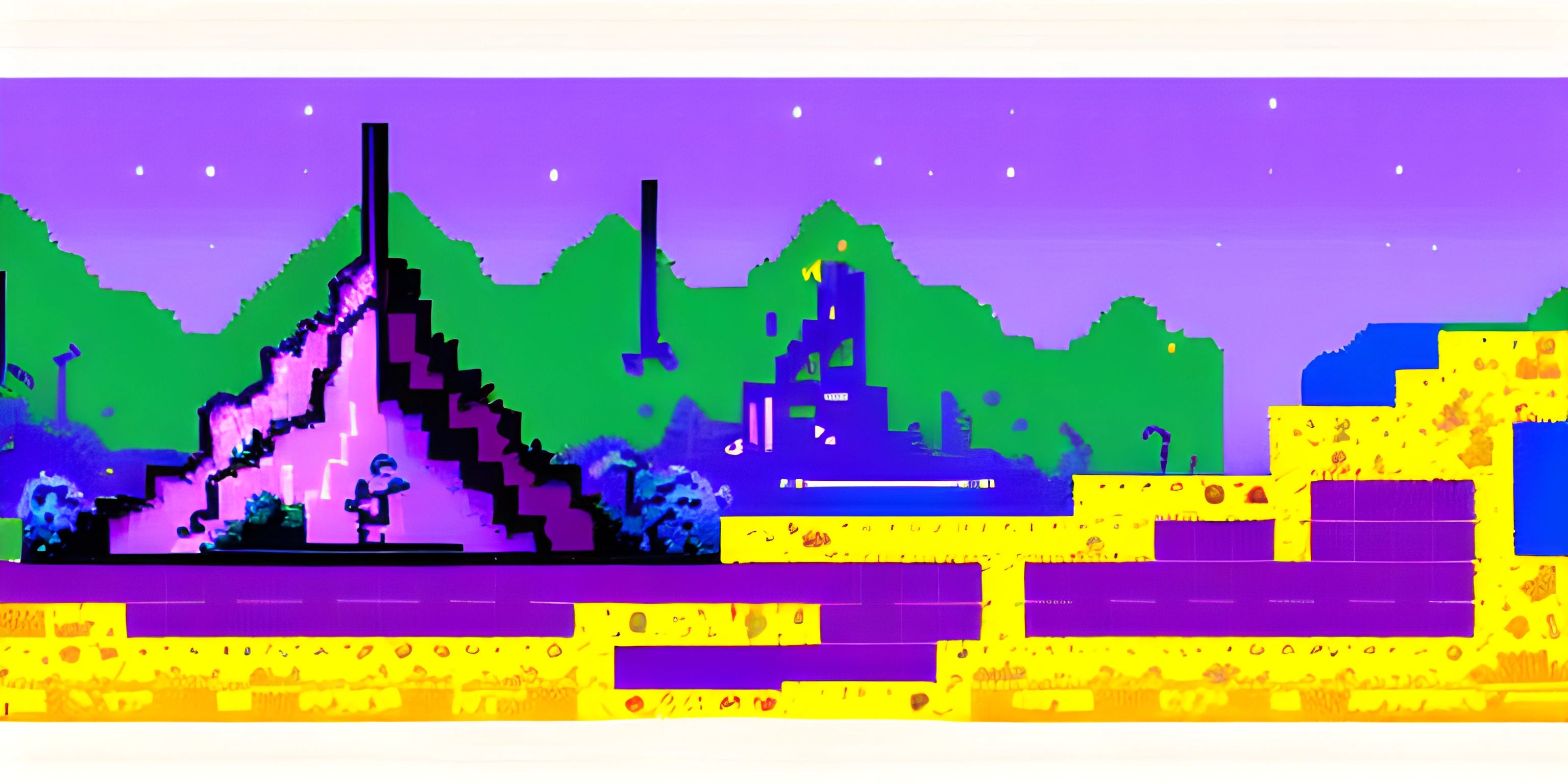
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Before we dive into the wonderful world of p5.js, let’s start with something fundamental: the setup function. Think of the setup function as the grand opening of a theater performance. The curtains rise, the lights dim, and the stage is set. This is where your sketches will come to life!
The setup function in p5.js is called once when the program starts. It’s used to define initial environment properties such as screen size, background color, and to load media such as images and fonts. Without further ado, let’s jump into this magical world and learn how to set the stage!
The Stage: Setting Up the Canvas
The first order of business in the setup function is usually to create the canvas. The canvas is your drawing board, your blank slate, the place where all the magic happens.
function setup() { createCanvas(800, 600); background(200); }
In the example above, we’re creating a canvas that is 800 pixels wide and 600 pixels tall. The createCanvas
function is like setting the dimensions of your stage. The background
function sets the initial color of your canvas to a shade of gray (where 200 is the gray value).
Adding Some Flair: Customizing the Setup
Let’s say you want to add a bit more pizzazz to your stage. You can customize the setup function to suit your artistic needs. Here are a few examples:
Setting Background Color
You can set the background color using RGB values:
function setup() { createCanvas(800, 600); background(0, 255, 0); // Green background }
Or even use named colors:
function setup() { createCanvas(800, 600); background("skyblue"); }
Loading Media
If your sketch involves images or fonts, the setup function is the perfect place to load them. Loading media in the setup function ensures that they are ready to go when needed.
let img; function preload() { img = loadImage("path/to/image.jpg"); } function setup() { createCanvas(800, 600); image(img, 0, 0); }
In this case, we use the preload
function to load the image before the setup function runs. This ensures the image is fully loaded and available when we draw it.
Let There Be Light: Drawing in Setup
While the primary purpose of the setup function is to set the stage, you can also draw shapes and lines directly in it. However, it’s generally better to use the setup function for initialization and reserve drawing for the draw
function, which is called repeatedly to create animations.
function setup() { createCanvas(800, 600); background(255); // Draw a line stroke(0); line(100, 100, 700, 500); // Draw a rectangle noFill(); rect(300, 200, 200, 100); }
In the example above, we draw a line and a rectangle directly in the setup function. This is perfectly fine for static sketches, but for dynamic content, you’ll want to harness the power of the draw function.
The Role of Global Variables
If you’re going to be using variables throughout your sketch, it’s a good idea to declare them globally. This makes them accessible in both the setup and draw functions.
let x, y; function setup() { createCanvas(800, 600); x = width / 2; y = height / 2; } function draw() { background(200); ellipse(x, y, 50, 50); }
In this case, x
and y
are declared globally and initialized in the setup function. They are then used in the draw function to position a circle.
Wrapping Up: Best Practices
Here are a few best practices to keep in mind when using the setup function:
- Initialize Variables: Declare and initialize variables you’ll use throughout your sketch.
- Set Environment Properties: Define properties like canvas size and background color.
- Load Media: Load images, fonts, and other media to ensure they are ready when needed.
- Avoid Heavy Processing: Keep heavy processing out of the setup function to ensure it runs quickly.
By following these best practices, you can ensure your sketches are well-organized and efficient.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).
FAQ
Can I draw shapes in the setup function?
Yes, you can draw shapes in the setup function. However, it's generally better to use the setup function for initialization and reserve drawing for the draw function, especially for dynamic content.
What is the purpose of the preload function?
The preload function is used to load media such as images and fonts before the setup function runs. This ensures that all media are fully loaded and available when needed.
Can I change the canvas size after the setup function runs?
No, the canvas size is typically set in the setup function using the createCanvas function. If you need to change the canvas size dynamically, you can use the resizeCanvas function in the draw function or other event handlers.
What happens if I don't include a setup function in my p5.js sketch?
If you don't include a setup function in your p5.js sketch, the canvas will not be created, and the default setup will not run. The setup function is essential for initializing your sketch.
Can I use multiple setup functions in a single p5.js sketch?
No, you can only have one setup function in a p5.js sketch. Having multiple setup functions will cause a conflict, and only one of them will run.