User Input with p5.js
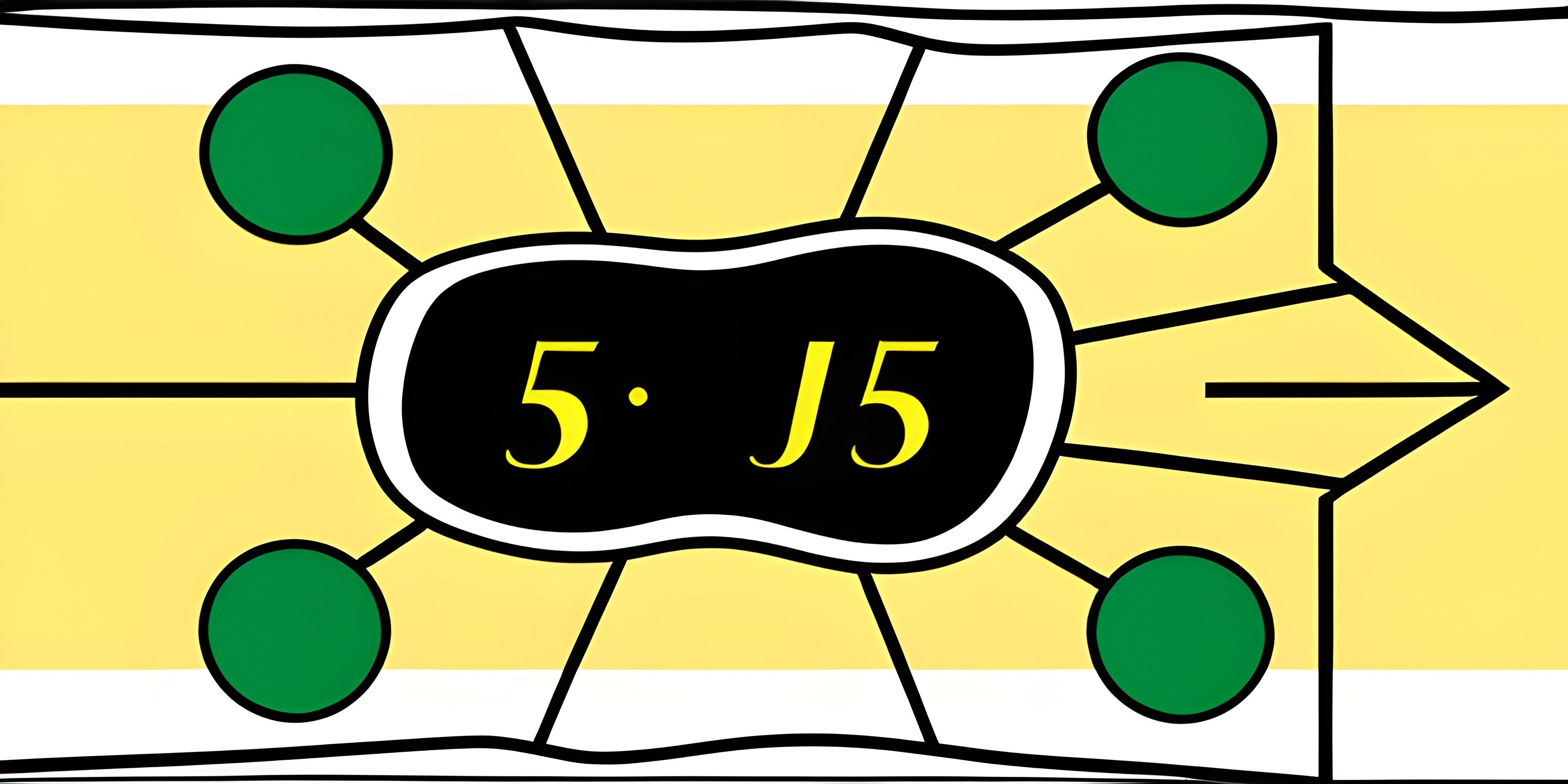
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Creating interactive sketches with p5.js is all about handling user input. To make your sketch come to life and respond to users, you'll need to listen for events such as mouse clicks, keyboard presses, and more. Let's dive in and see how we can add user input to our p5.js sketches.
Responding to Mouse Input
In p5.js, there are built-in functions that automatically get called when certain mouse events occur. You can define what should happen during these events by implementing the corresponding functions in your sketch.
Mouse Pressed
To detect when the mouse is pressed, we can use the mousePressed()
function. This function gets called whenever the user presses any mouse button. Here's an example:
function setup() { createCanvas(400, 400); } function draw() { background(220); } function mousePressed() { ellipse(mouseX, mouseY, 50, 50); }
In this example, a circle will be drawn at the mouse position whenever the user presses a mouse button.
Mouse Released
Similarly, the mouseReleased()
function gets called when any mouse button is released. You can implement this function to add interactivity to your sketch when the user releases the mouse button.
function setup() { createCanvas(400, 400); } function draw() { background(220); } function mouseReleased() { rect(mouseX, mouseY, 50, 50); }
Now, a rectangle will be drawn at the mouse position when the user releases the mouse button.
Responding to Keyboard Input
Just like mouse input, p5.js provides built-in functions for handling keyboard events. Let's see how we can add keyboard interactions to our sketch.
Key Pressed
The keyPressed()
function is called whenever a key on the keyboard is pressed. You can use this function to perform actions based on specific key presses.
function setup() { createCanvas(400, 400); } function draw() { background(220); } function keyPressed() { if (key === "A" || key === "a") { ellipse(random(width), random(height), 50, 50); } }
In this example, when the user presses the "A" key, a circle will be drawn at a random position on the canvas.
Key Released
The keyReleased()
function is called when a key is released. You can use this function to add interactivity based on key releases.
function setup() { createCanvas(400, 400); } function draw() { background(220); } function keyReleased() { if (key === "R" || key === "r") { rect(random(width), random(height), 50, 50); } }
Now, when the user releases the "R" key, a rectangle will be drawn at a random position on the canvas.
Wrapping Up
By implementing these built-in functions for mouse and keyboard events, you can create engaging and interactive p5.js sketches. Don't be afraid to experiment with different events and combinations to design unique experiences for your users. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Taking User Input (psst, it's free!).
FAQ
How do I create an interactive sketch with p5.js?
To create an interactive sketch with p5.js, you need to handle user input and respond to events. You can use p5.js built-in event handling functions, like mousePressed()
, mouseReleased()
, keyPressed()
, and keyReleased()
. These functions are automatically called by the library when the corresponding events occur. For example, to change the background color when the mouse is clicked:
function setup() { createCanvas(400, 400); } function draw() { background(220); } function mousePressed() { background(random(255), random(255), random(255)); }
How can I detect if a specific keyboard key is pressed in p5.js?
You can detect if a specific keyboard key is pressed using the key
or keyCode
variables inside the keyPressed()
or keyReleased()
functions. The key
variable stores the character representation of the key, while keyCode
stores the numeric key code. Here's an example of how to detect if the spacebar is pressed:
function keyPressed() { if (key == " " || keyCode === 32) { console.log("Spacebar pressed!"); } }
How do I get the mouse's current position in p5.js?
To get the mouse's current position in p5.js, you can use the built-in variables mouseX
and mouseY
. These variables store the current x and y coordinates of the mouse cursor. You can use them to draw shapes or text at the mouse position, like this:
function draw() { background(220); fill(255, 0, 0); ellipse(mouseX, mouseY, 50, 50); }
Can I detect if the mouse is being dragged in p5.js?
Yes, you can detect if the mouse is being dragged by using the mouseIsPressed
variable in combination with the mouseX
and mouseY
variables. The mouseIsPressed
variable is true when any mouse button is pressed and false otherwise. Here's an example of how to draw a line while the mouse is being dragged:
let prevX, prevY; function setup() { createCanvas(400, 400); prevX = mouseX; prevY = mouseY; } function draw() { if (mouseIsPressed) { line(prevX, prevY, mouseX, mouseY); } prevX = mouseX; prevY = mouseY; }
How can I create custom event listeners in p5.js?
You can create custom event listeners by using the createButton()
or createSlider()
functions to generate HTML elements, and then attaching event listeners using the .mousePressed()
, .mouseReleased()
, or .changed()
methods. For example, to create a button that changes the background color when clicked:
function setup() { createCanvas(400, 400); let button = createButton("Change Color"); button.mousePressed(changeColor); } function draw() { background(220); } function changeColor() { background(random(255), random(255), random(255)); }