Python Variables: Unlocking the Power of Data Storage
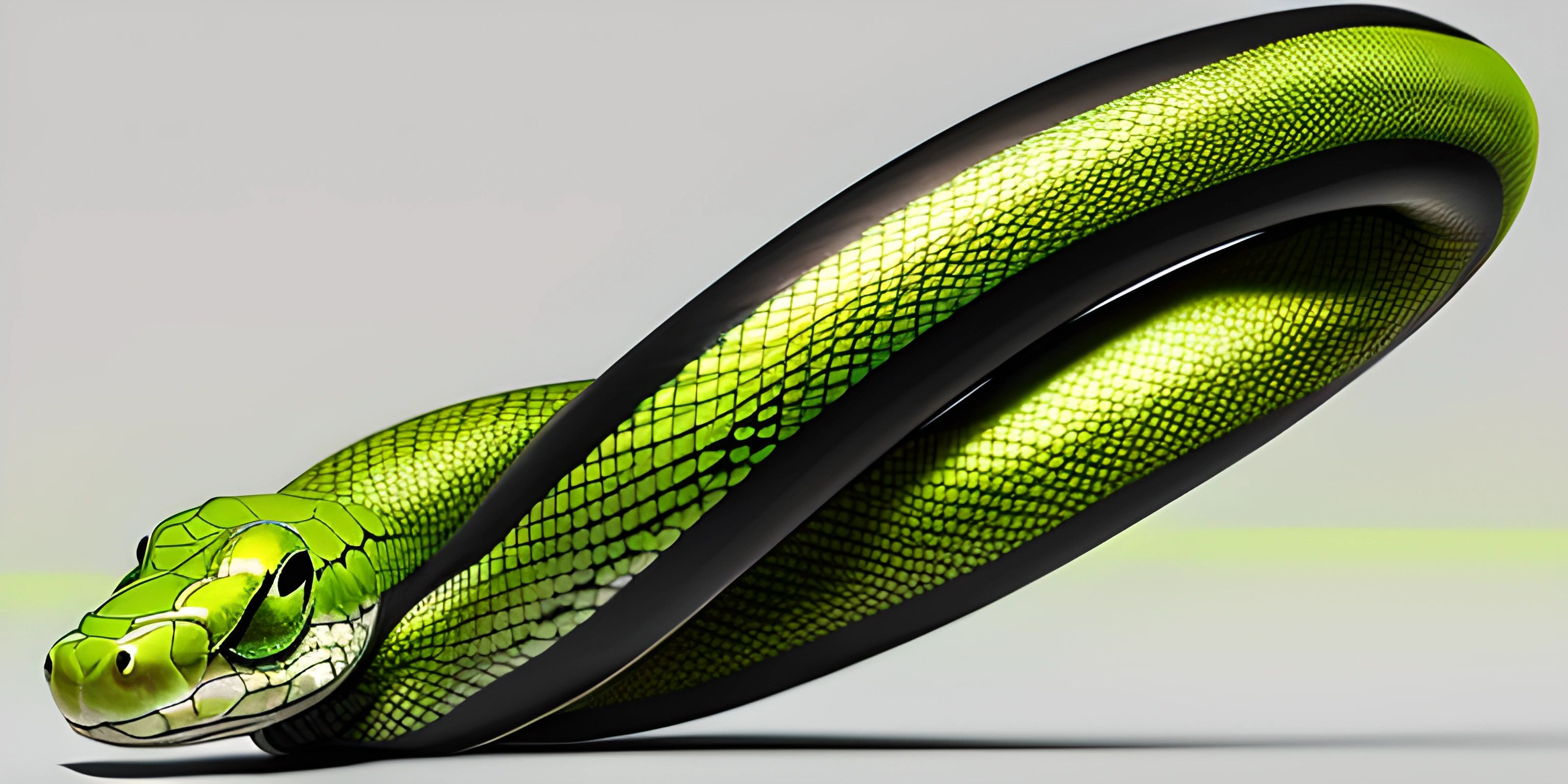
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When venturing into the world of Python programming, variables are like the trusty sidekicks that help you store and manage your data. They make your code more efficient, flexible, and way less prone to errors. Let's dive into the realm of variables in Python and see how they can make your life a whole lot easier.
What are Python Variables?
In Python, variables are like containers or labels that store data. They can hold a wide range of data types, such as numbers, strings, lists, and even complex objects. You can think of variables as name tags you put on data so that you can easily find and manipulate them later in your code.
Creating and Assigning Variables
Before you can use a variable, you need to create it and assign some data to it. You do this with the assignment operator, which is the equal sign (=
). Here's a quick example:
name = "Alice" age = 30
In this example, we've created two variables: name
and age
. We assigned the string "Alice"
to the name
variable and the number 30
to the age
variable.
Using Variables
Now that we have our variables set up, we can use them in various ways, such as printing their values, performing calculations, or passing them to functions.
print("Hello, my name is", name, "and I am", age, "years old.")
This code will output: Hello, my name is Alice and I am 30 years old.
Changing Variable Values
One of the great things about variables is that they're, well, variable. You can easily change their values throughout your code. Let's say Alice just had a birthday and is now 31 years old. You can update the age
variable like this:
age = 31
Now, if you were to print the same message from before, it would say: Hello, my name is Alice and I am 31 years old.
Variable Naming Rules
There are a few rules you should keep in mind when naming your Python variables:
- Variable names must start with a letter or an underscore (_).
- Variable names can contain letters, numbers, and underscores.
- Variable names are case-sensitive (
name
,Name
, andNAME
are three different variables).
It's also a good practice to give your variables descriptive names that indicate their purpose. For example, user_age
is more meaningful than x
.
Conclusion
Variables in Python are fundamental building blocks of your code, allowing you to store and manipulate data with ease. By creating, assigning, and using variables, you can make your code more efficient, flexible, and less error-prone. So, start harnessing the power of Python variables and watch your code soar to new heights!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What is a variable in Python?
A variable in Python is like a container or a placeholder to store and manipulate various types of data. It allows you to give a name to your data and refer to it later in your code. Variables can store different types of data, such as numbers, strings, lists, and more.
How do I create and assign a value to a variable in Python?
Creating and assigning a value to a variable in Python is quite simple. Just choose a name for the variable, followed by an equal sign (=), and then the value you want to assign. For example, to create a variable called my_variable
and assign the value 42
to it, you would write:
my_variable = 42
Can I assign multiple variables at once in Python?
Yes, you can assign multiple variables at once in Python using a single line of code. You just need to separate the variable names and their corresponding values with commas. For example:
variable1, variable2, variable3 = 10, "hello", 3.14
In this example, variable1
is assigned the value 10
, variable2
is assigned the value "hello"
, and variable3
is assigned the value 3.14
.
How do I change the value of a variable after it has been created?
To change the value of a variable in Python, simply reassign a new value to the variable using the equal sign (=) again. For example, let's say you have a variable called my_number
with the initial value of 10
. To change its value to 42
, you would write:
my_number = 42
What are the naming rules for Python variables?
When naming Python variables, you should follow these rules:
- Variable names must start with a letter or an underscore (_).
- They can only contain letters, digits, and underscores.
- Variable names are case-sensitive, meaning
myVariable
andmyvariable
are considered different variables. - Avoid using reserved Python keywords as variable names, such as
if
,else
,and
,while
, etc.