Rust Inline Assembly
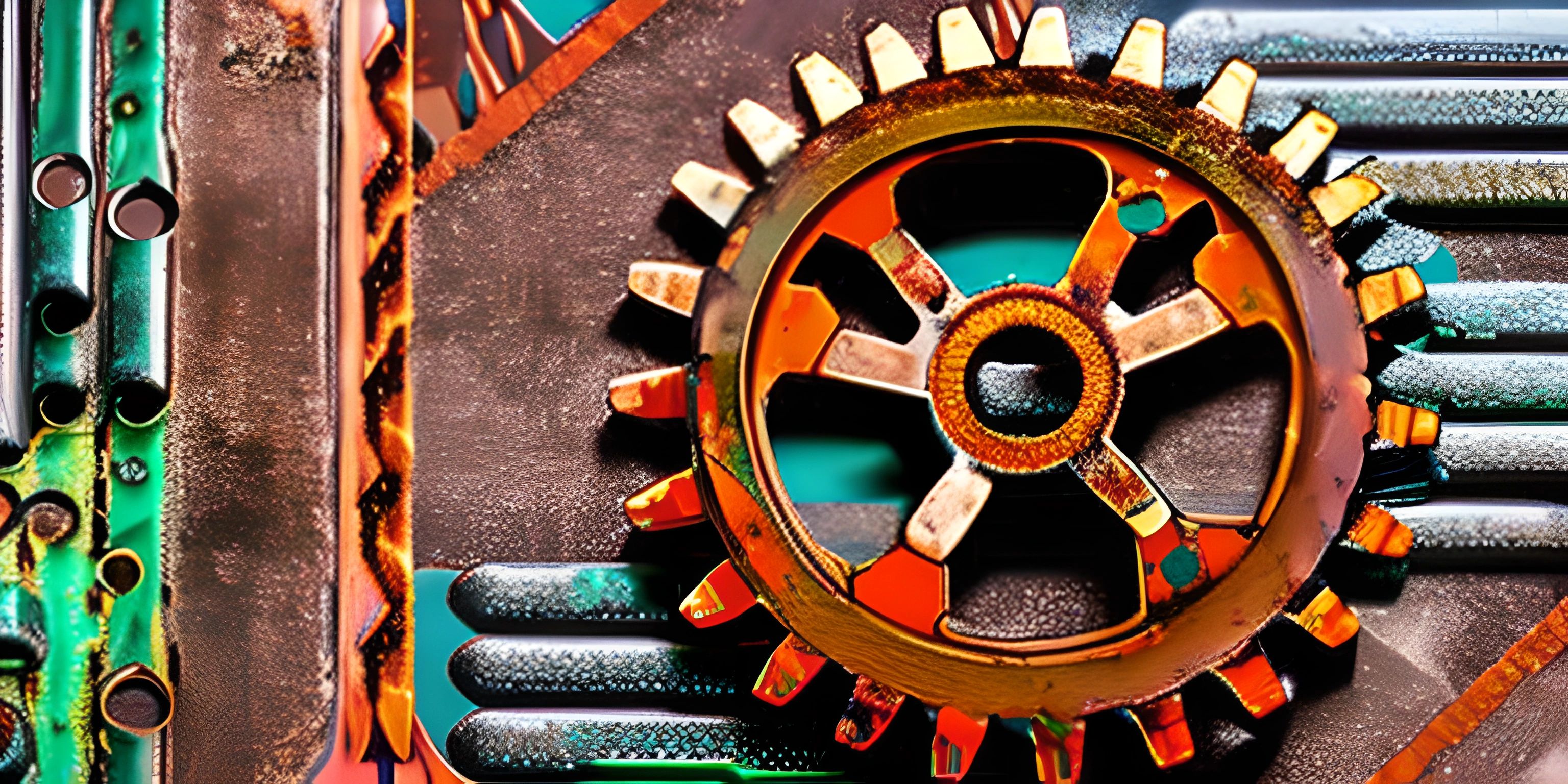
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Let's say that you're working on some high-performance Rust code and you realize that you can optimize it even more by using assembly. That's when inline assembly comes to the rescue! Inline assembly allows you to include assembly language instructions directly within your Rust function to make it more efficient, especially when working with vectors.
Introduction to Inline Assembly
In Rust, the asm!
macro is used to include inline assembly code. This powerful feature allows you to perform operations that are not available or optimal through Rust's standard library. When using inline assembly, it's essential to be cautious and aware of the potential risks, such as introducing security vulnerabilities, making your code less portable, and hindering readability. However, with great power comes great responsibility, so use it wisely!
Manipulating Vectors with Inline Assembly
Let's dive into an example of using inline assembly within a Rust function to manipulate vectors. We'll create a function that adds two vectors together element-wise and stores the result in a third vector. Here's the Rust code without inline assembly:
fn add_vectors(a: &[i32], b: &[i32], result: &mut [i32]) { for i in 0..a.len() { result[i] = a[i] + b[i]; } }
Now, let's see how we can use inline assembly to optimize this function:
fn add_vectors_asm(a: &[i32], b: &[i32], result: &mut [i32]) { let len = a.len(); let mut i = 0; while i < len { unsafe { asm!( "mov eax, [{} + 4*{}]", "add eax, [{} + 4*{}]", "mov [{} + 4*{}], eax", inout(reg) a.as_ptr() => _, inout(reg) b.as_ptr() => _, inout(reg) result.as_mut_ptr() => _, in(reg) i, ); } i += 1; } }
In this example, we use the asm!
macro to insert assembly instructions directly into our Rust function. The assembly code reads the elements from the vectors a
and b
, adds them together, and stores the result in the result
vector. We use the unsafe
keyword because inline assembly can have side effects that Rust's safety guarantees cannot account for.
Conclusion
Using inline assembly in Rust is a powerful optimization tool for low-level operations, such as manipulating vectors. However, it's essential to use it carefully and responsibly due to the potential risks involved. Remember, with great power comes great responsibility, and when used wisely, inline assembly can help you achieve amazing performance gains in your Rust code!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is inline assembly in Rust?
"Inline assembly" refers to the practice of embedding assembly code directly within a higher-level programming language like Rust. It allows developers to write low-level, processor-specific instructions within the Rust code, providing fine-grained control and performance optimizations. In Rust, the asm!
macro is used to write inline assembly code.
How do I use the `asm!` macro for inline assembly in Rust?
To use the asm!
macro for inline assembly in Rust, you need to follow these steps:
- Ensure you're using the nightly Rust compiler, as the
asm!
macro is still an unstable feature. - Add the
#![feature(asm)]
attribute to the top of your source file. - Use the
asm!
macro within your function, providing the assembly code as a string and specifying input/output operands. Here's an example:
#![feature(asm)] fn main() { let x: u64 = 1; let y: u64; unsafe { asm!( "add {0}, {1}, {2}", out(reg) y, in(reg) x, const 5 ); } println!("x + 5 = {}", y); }
Can I use inline assembly to manipulate vectors in Rust?
Yes, you can use inline assembly within Rust functions to efficiently manipulate vectors. This technique can be particularly useful when working with SIMD (Single Instruction, Multiple Data) operations, as you can use processor-specific SIMD instructions to perform operations on vectors in parallel. Make sure you're familiar with the target processor's instruction set, as well as any relevant Rust syntax and constraints, before attempting to write vector manipulation code using inline assembly.
What are the potential risks of using inline assembly in Rust?
While inline assembly in Rust can offer performance optimizations and fine-grained control, there are some potential risks and drawbacks to consider:
- Portability: Inline assembly code is processor-specific, which means that your code may not work on different processors or architectures without modification.
- Readability: Assembly code can be harder to read and understand than Rust code, potentially making your codebase more challenging to maintain.
- Safety: Writing incorrect or unsafe assembly code may introduce hard-to-debug issues or security vulnerabilities in your program. Always use caution and test your code thoroughly when working with inline assembly.