Understanding Rust's Prelude
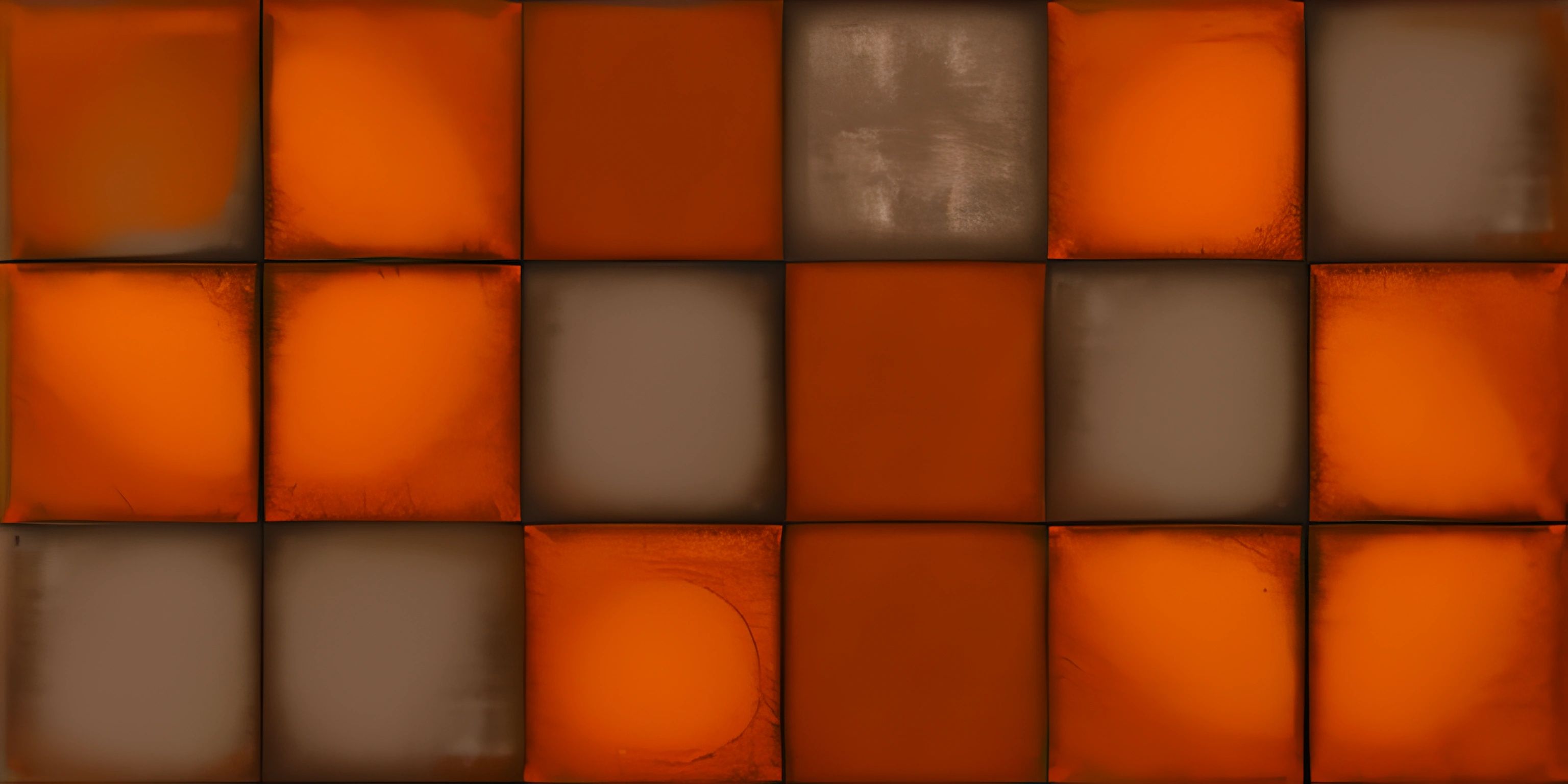
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
The Rust programming language is known for its safety, concurrent execution, and performance. Before diving into the depths of Rust, you should know about the magical world of the Rust Prelude. It's like the concierge desk of a fancy hotel, providing you with a set of default services and functionalities to make your stay (or coding) more enjoyable.
What is the Prelude?
In Rust, the Prelude is a small collection of commonly used items from the standard library that are automatically imported into every Rust program. This means that you can use these items without explicitly importing them, making your code cleaner and more concise.
The Rust Prelude is part of the std
(standard) library and can be found under std::prelude
. It includes essential traits, functions, and types that are commonly used across various Rust programs.
Why is the Prelude Important?
The Prelude's main purpose is to reduce boilerplate code and make your Rust programming experience smoother. By importing common items by default, it saves you time and effort in typing out import statements for frequently used functionalities.
Imagine having to import essential items like Option
, Result
, or Vec
in every single file you write. It would be like having to carry your own chair to every room of the hotel! The Prelude takes care of this for you, allowing you to focus on the actual logic of your program.
What's Included in the Prelude?
While the Prelude includes a variety of items, some of the most commonly used ones are:
- The
Option
andResult
types: These are essential for error handling and dealing with the possibility of missing values. - The
Vec
andString
types: These are widely used data structures for holding collections and text data. - The
Drop
andClone
traits: These are fundamental for managing the life cycle of your structs and objects. - The
Iterator
and related traits: These allow you to create and work with iterators, a powerful way to process collections of data.
Remember, the Prelude is not exhaustive, and you may still need to import additional items from the standard library or external crates to meet your specific needs.
In Conclusion
The Rust Prelude is like the friendly hotel staff, always there to make your stay more comfortable. It simplifies your Rust programming life by providing you with a default set of commonly used items from the standard library. This way, you can focus on writing your code and marveling at Rust's powerful features, without getting bogged down by repetitive import statements.
So, the next time you write a Rust program, remember to give a little nod to the Prelude for making your coding journey smoother and more enjoyable.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What is Rust's Prelude?
Rust's Prelude is a small collection of commonly used items that are automatically imported into every Rust program. It simplifies the Rust programming experience by making it unnecessary to manually import these common items, such as traits, macros, and types, in each source file.
Why does Rust have a Prelude?
Rust has a Prelude to streamline the development process and reduce boilerplate code. By automatically importing frequently used items, Rust's Prelude saves developers the effort of repeatedly writing import statements for these common items. This allows developers to focus on writing the actual logic of their programs and makes the code more concise.
Can I customize Rust's Prelude?
Yes, you can customize Rust's Prelude. While the default Prelude is designed to suit most use cases, you may have specific requirements that necessitate custom imports. To create your own Prelude, you can define a prelude
module in your project and then use the pub use
keyword to re-export items. For example:
mod my_custom_prelude { pub use std::io::{self, Read, Write}; // Re-exporting items from the standard library pub use crate::my_custom_trait::MyTrait; // Re-exporting a custom trait from your crate }
To use your custom Prelude, add a use
statement in your source files:
use crate::my_custom_prelude::*;
Can I disable Rust's Prelude?
Yes, you can disable Rust's Prelude if you want complete control over the imports in your Rust program. To disable the default Prelude, add the following attribute to your crate root:
#![no_implicit_prelude]
Keep in mind that disabling the Prelude means you'll have to manually import all the required items in each source file.
What are some common items found in Rust's Prelude?
Rust's Prelude includes several commonly used items, such as standard traits, types, and macros. Some examples include:
std::marker::{Copy, Send, Sized, Sync}
std::ops::{Drop, Fn, FnMut, FnOnce}
std::mem::drop
std::boxed::Box
std::clone::Clone
std::cmp::{PartialEq, PartialOrd, Eq, Ord}
For a complete list of items found in Rust's Prelude, refer to the official documentation.