Rust Standard Library
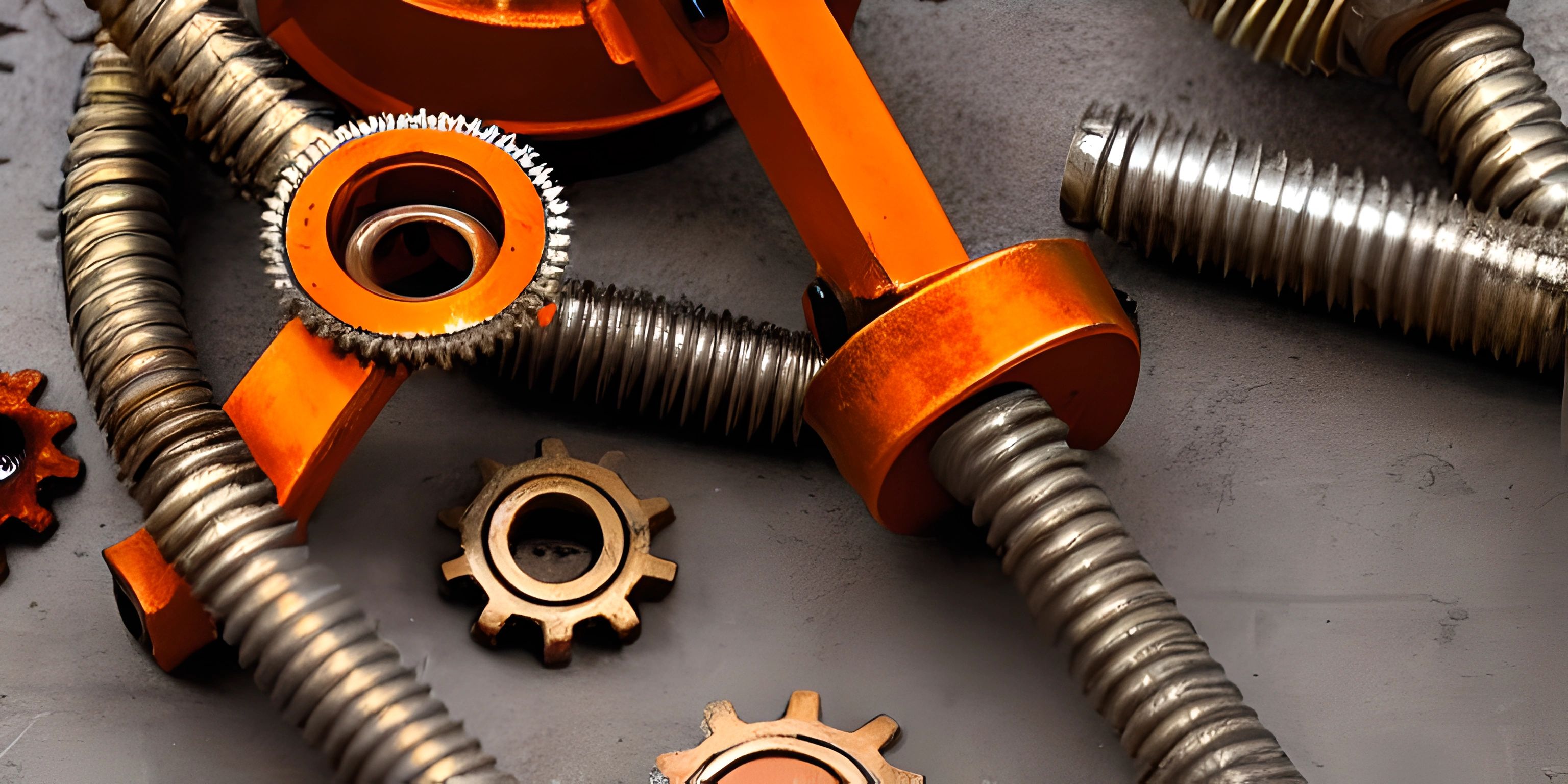
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
The Rust programming language is known for its safety, performance, and concurrency. In this magical journey, we'll explore the wondrous world of the Rust Standard Library, which provides many tools and features that will help you create powerful and efficient applications. So buckle up, and let's dive in!
What is the Rust Standard Library?
The Rust Standard Library, often referred to as std
, is a collection of pre-built functionality that comes with the language itself. It offers a wide range of tools, from basic data structures like Vectors and HashMaps, to more advanced features such as threading and I/O operations.
How to Use the Rust Standard Library
To use the Rust Standard Library, simply include the use std::
statement in your code, followed by the specific module or function you want to import. For example, to use the println!
macro, you would write:
use std::println; fn main() { println!("Hello, world!"); }
However, you don't always have to include the use std::
statement, as Rust automatically imports the prelude, which contains a subset of commonly used items from the Standard Library. For example, the println!
macro is part of the prelude, so you can use it without explicitly importing it.
Key Features of the Rust Standard Library
Here are some of the most popular and powerful features that the Rust Standard Library has to offer:
Collections
Rust provides several useful data structures in the std::collections
module, such as Vec
, HashMap
, HashSet
, and LinkedList
. These collections are designed to be flexible, efficient, and safe to use.
For example, here's how to create and manipulate a Vec
(short for vector), which is a dynamic array:
use std::vec::Vec; fn main() { let mut numbers = Vec::new(); numbers.push(42); numbers.push(7); for number in &numbers { println!("{}", number); } }
Error Handling
The Rust Standard Library offers a powerful and ergonomic way to handle errors through the Result
and Option
enums. These two types allow you to write expressive and safe code that can recover gracefully from errors.
Here's an example of using the Result
enum to handle file I/O operations:
use std::fs::File; use std::io::Read; fn main() { let mut file = match File::open("example.txt") { Ok(file) => file, Err(error) => panic!("Error opening file: {}", error), }; let mut contents = String::new(); match file.read_to_string(&mut contents) { Ok(_) => println!("File contents: {}", contents), Err(error) => panic!("Error reading file: {}", error), } }
Concurrency
Rust's Standard Library provides excellent support for concurrency through the std::thread
module, allowing you to create and manage threads with ease. Additionally, Rust's type system and ownership model ensure that your concurrent code is free from data races and other common pitfalls.
Here's a simple example of running a function in a separate thread:
use std::thread; use std::time::Duration; fn main() { let handle = thread::spawn(|| { for i in 1..10 { println!("Hello from spawned thread! ({})", i); thread::sleep(Duration::from_millis(500)); } }); for i in 1..5 { println!("Hello from main thread! ({})", i); thread::sleep(Duration::from_millis(1000)); } handle.join().unwrap(); }
As you can see, the Rust Standard Library offers a wealth of tools and features to help you create powerful and efficient applications. Don't be afraid to explore its vast landscape, and remember - with great power comes great responsibility. Happy coding!
FAQ
What is the Rust Standard Library?
The Rust Standard Library is a collection of tools, utilities, and pre-defined functions that make it easier for developers to build robust and efficient applications using the Rust programming language. It includes support for data structures, file handling, concurrency, and much more.
How can I access and use the Rust Standard Library in my project?
To access and use the Rust Standard Library, you simply need to include the std
keyword in your code. The library is included by default in every Rust program. For example, to use the std::collections::HashMap
data structure, you would write:
use std::collections::HashMap; fn main() { let mut my_map = HashMap::new(); my_map.insert("key", "value"); println!("{:?}", my_map); }
What are some essential features provided by the Rust Standard Library?
The Rust Standard Library offers a wide range of features, some of which include:
- Data structures like vectors, hash maps, and linked lists.
- File handling and I/O operations.
- Concurrency primitives like threads, mutexes, and atomic operations.
- Error handling with the
Result
andOption
enums. - Utility functions for manipulating strings, numbers, and other types.
How does Rust Standard Library help with error handling?
The Rust Standard Library provides two powerful enums, Result
and Option
, which help in error handling and avoiding the use of null values. These enums work with Rust's pattern matching to handle errors gracefully and make your code more robust. For example, here's how you might use the Result
enum to handle file reading errors:
use std::fs::File; use std::io::Read; use std::io; fn read_file(filename: &str) -> Result<String, io::Error> { let mut file = File::open(filename)?; let mut content = String::new(); file.read_to_string(&mut content)?; Ok(content) } fn main() { match read_file("example.txt") { Ok(content) => println!("File content: {}", content), Err(e) => println!("Error reading file: {}", e), } }
Can I use external libraries along with the Rust Standard Library?
Yes, you can use external libraries in your Rust project in addition to the Rust Standard Library. To do so, you need to add the required libraries to your Cargo.toml
file in the [dependencies]
section, and then use the use
keyword to include them in your code.