Java Exception Handling
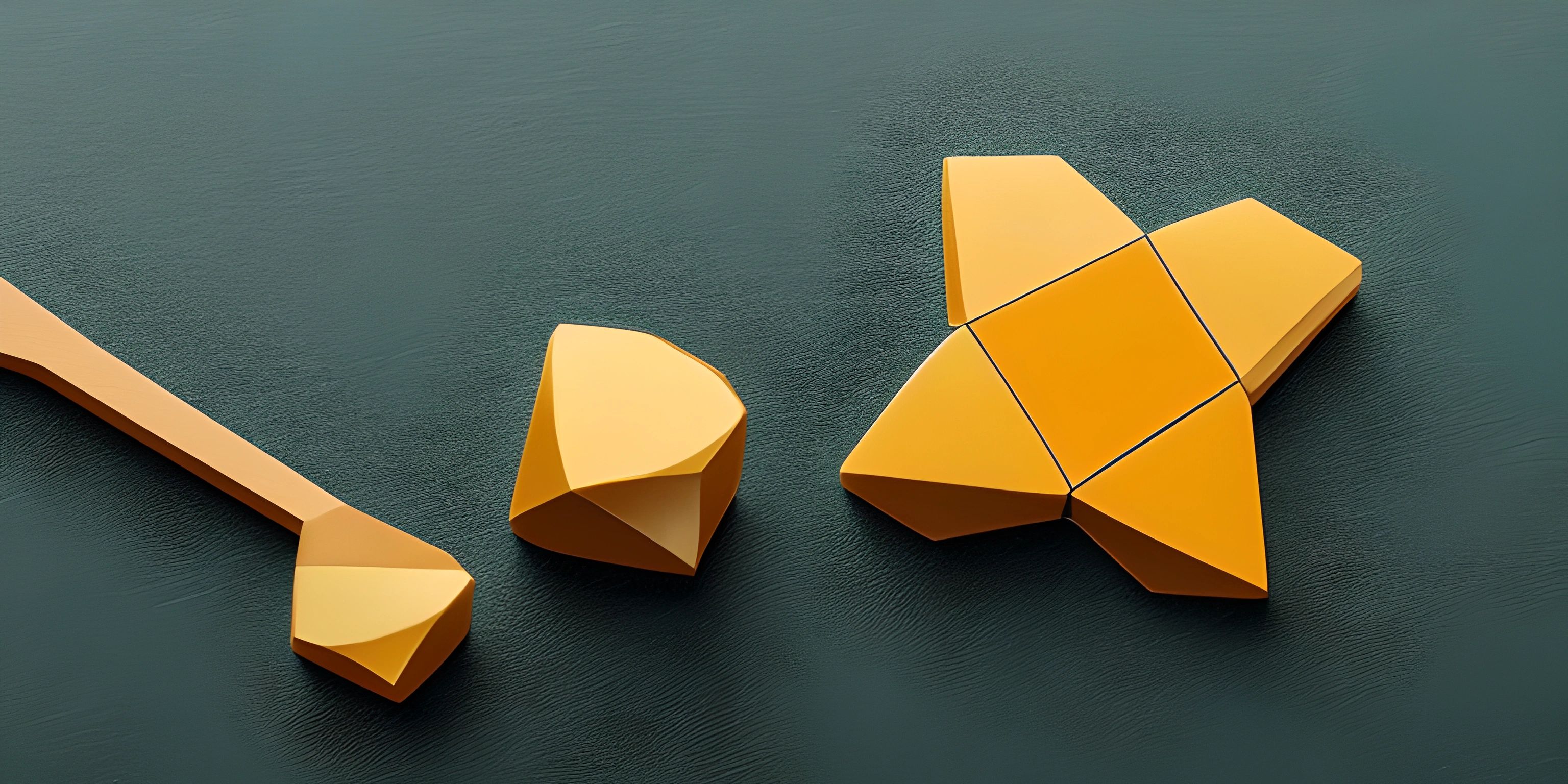
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Bumps in the road are inevitable when programming, and whether it's a user input error or a wrong file path, exceptions will happen. In Java, exception handling allows you to create robust and error-resistant programs. Let's explore how exception handling in Java works and how to harness its power effectively.
Understanding Exceptions
An exception is an event that occurs during the execution of a program and disrupts the normal flow of instructions. Exceptions are Java's way of saying, "Hey, something went wrong! Let's deal with it." Java provides a built-in mechanism to handle errors and exceptions, using try-catch-finally blocks and the throw and throws keywords.
Try-Catch-Finally
A try-catch-finally block is the foundation of exception handling in Java. In simple terms, it's like saying, "Try to do this, but if something goes wrong, catch it and do this instead, and finally, clean up."
Here's an example:
try { // Code that might throw an exception int result = 8 / 0; } catch (ArithmeticException e) { // Code to handle the exception System.out.println("Oops! Division by zero is not allowed."); } finally { // Code to clean up, always executed regardless of an exception System.out.println("End of the try-catch block."); }
In this example, we're trying to perform a division by zero, which will throw an ArithmeticException
. The catch block handles the exception and displays a message, while the finally block executes at the end, regardless of whether an exception occurred or not.
Catching Multiple Exceptions
Java allows you to catch multiple exceptions in a single try-catch block. You can achieve this by using multiple catch blocks or using a multi-catch block.
try { // Code that might throw multiple exceptions } catch (IOException e) { // Handle IOException } catch (ClassNotFoundException e) { // Handle ClassNotFoundException } // Or using a multi-catch block try { // Code that might throw multiple exceptions } catch (IOException | ClassNotFoundException e) { // Handle both exceptions }
Throw and Throws
Sometimes, you might want to explicitly throw an exception or indicate that a method may throw an exception. Java provides two keywords for this: throw
and throws
.
Throw
Use the throw
keyword to explicitly throw an exception. For example:
void checkAge(int age) { if (age < 18) { throw new IllegalArgumentException("Age must be 18 or older."); } }
In this example, if the age is less than 18, an IllegalArgumentException
is thrown with a custom error message.
Throws
The throws
keyword is used to indicate that a method may throw one or more exceptions. This way, any method that calls the current method must handle these exceptions or declare that they might throw them as well. For example:
void readFile(String filePath) throws IOException, FileNotFoundException { // Code that might throw IOException or FileNotFoundException }
Here, the readFile
method indicates that it may throw IOException
and FileNotFoundException
.
Custom Exceptions
Java allows you to create your own custom exceptions by extending the Exception
class or one of its subclasses. This is useful when you want to create more meaningful and specific exceptions for your application.
class CustomException extends Exception { public CustomException(String message) { super(message); } }
In this example, a CustomException
class is created that extends the Exception
class. Now you can throw and catch this custom exception in your code.
try { // Code that might throw a CustomException throw new CustomException("This is a custom exception."); } catch (CustomException e) { // Handle the custom exception System.out.println(e.getMessage()); }
Now that you've mastered exception handling in Java, you can create more robust and error-resistant programs. Get ready to handle anything that comes your way! Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Chat App Backend (psst, it's free!).