Introduction to Apache Commons Lang
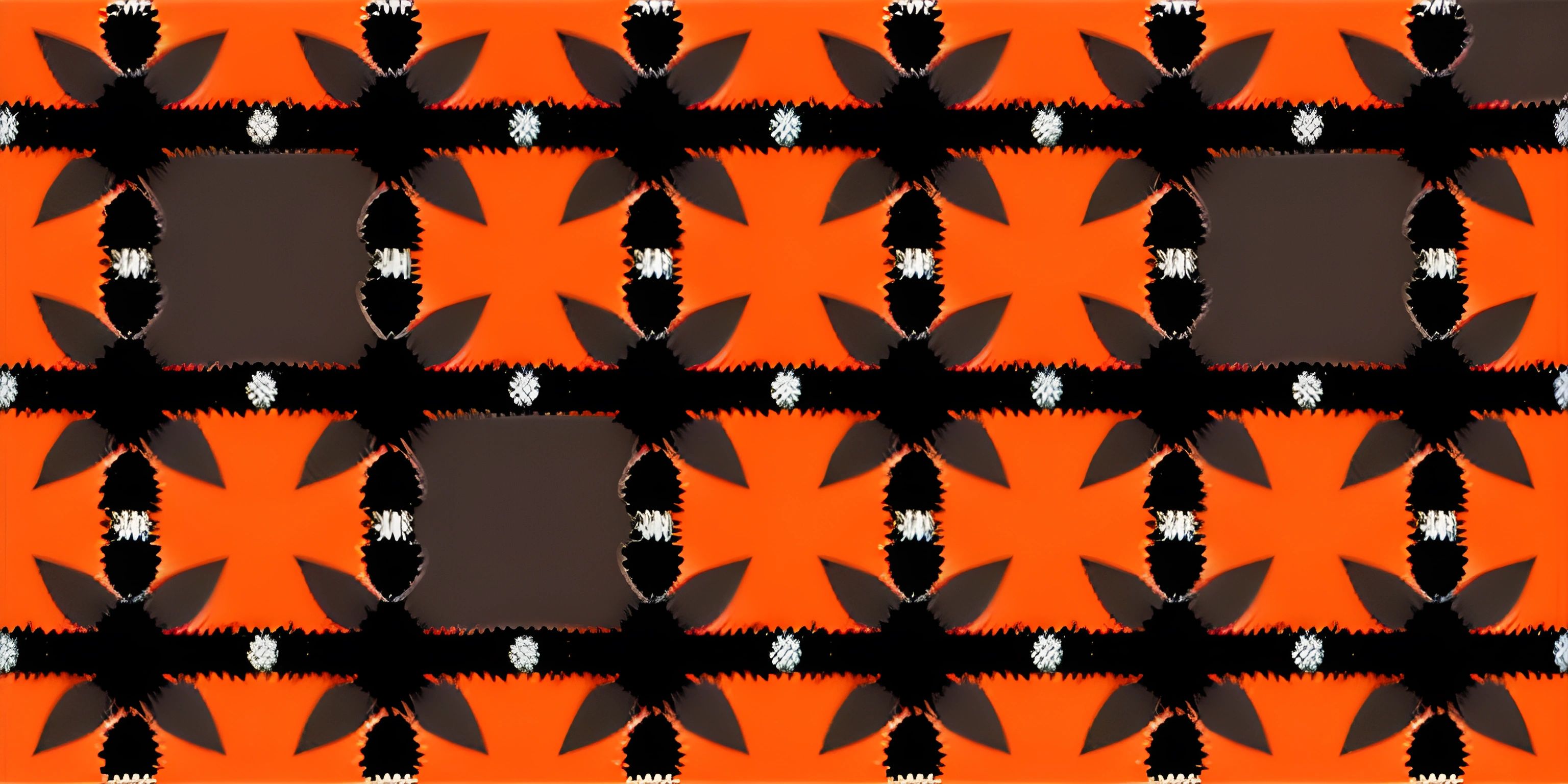
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Apache Commons Lang is a popular and widely-used Java library that provides a host of utility classes and methods to make your life as a developer easier. This library helps you deal with some of the most common programming tasks, such as working with strings, dates, and times, as well as providing additional functionality for working with other data types.
Why Use Apache Commons Lang?
As a developer, you're often presented with tasks that require you to handle various data types, manipulate them, and perform operations on them. Java, as a language, already provides some basic functionality, but it can be lacking in certain areas or can be cumbersome to use.
That's where Apache Commons Lang comes in; it supplements the Java standard library with additional utility classes and methods, making it more convenient to accomplish these tasks. Let's take a look at some of the most useful features this library has to offer.
String Manipulation
Working with strings is a common task in any programming language, but sometimes the standard library just doesn't provide enough functionality. Apache Commons Lang fills in the gaps with its StringUtils
class, which offers a multitude of methods for string manipulation. Some examples include:
StringUtils.isBlank()
andStringUtils.isNotBlank()
: These methods check if a string is blank (consisting of only whitespace characters) or not.StringUtils.substringBetween()
: Extracts a substring between two specified strings.StringUtils.capitalize()
: Capitalizes the first character of a string.StringUtils.join()
: Joins an array of strings or a collection of objects into a single string.
String input = " Cratecode is awesome! "; String trimmed = StringUtils.trim(input); // "Cratecode is awesome!" String reversed = StringUtils.reverse(trimmed); // "!emosewa si edoctarC"
Date and Time Utilities
Dealing with dates and times can be tricky, but Apache Commons Lang provides the DateUtils
class to make it a little easier. Some of the methods you'll find in this class include:
DateUtils.addDays()
,DateUtils.addHours()
,DateUtils.addMinutes()
: These methods allow you to add or subtract a specified amount of time to a given date.DateUtils.truncate()
: Truncates a date object to a specified field, such as day, hour, or minute.DateUtils.isSameDay()
: Determines if two dates are on the same day, regardless of the time.
Date now = new Date(); Date tomorrow = DateUtils.addDays(now, 1); Date startOfDay = DateUtils.truncate(now, Calendar.DAY_OF_MONTH);
Additional Utilities
Apache Commons Lang doesn't stop at just strings and dates; you'll find utility classes for various other data types as well. Some examples include:
ArrayUtils
: Contains methods for working with arrays, such as adding or removing elements, checking if an array contains a specific element, and converting arrays to other data structures.NumberUtils
: Offers methods for working with numbers, such as parsing strings into numbers, checking if a string is a valid number, and finding minimum or maximum values.ObjectUtils
: Provides methods for working with objects, such as checking for null values and performing null-safe comparisons.
int[] numbers = {1, 2, 3, 4, 5}; int[] updatedNumbers = ArrayUtils.add(numbers, 6); // {1, 2, 3, 4, 5, 6} int maxValue = NumberUtils.max(updatedNumbers); // 6
Conclusion
Apache Commons Lang is a powerful and versatile library that can make your life as a Java developer much easier. By providing a wealth of utility methods for handling strings, dates, times, and other data types, it allows you to focus on your application's logic instead of reinventing the wheel when it comes to common programming tasks.
FAQ
What is Apache Commons Lang?
Apache Commons Lang is a widely-used Java library that provides utility classes and methods to simplify and enhance various common programming tasks, such as working with strings, dates, times, and other data types.
In which programming language is Apache Commons Lang available?
Apache Commons Lang is available for the Java programming language.
What are some useful features of the StringUtils class in Apache Commons Lang?
StringUtils class in Apache Commons Lang provides methods for string manipulation, such as checking if a string is blank or not, extracting substrings between specified strings, capitalizing the first character of a string, and joining arrays or collections of strings.
How can Apache Commons Lang help with date and time manipulation?
Apache Commons Lang provides the DateUtils class, which offers methods to add or subtract time to a given date, truncate a date object to a specified field, determine if two dates are on the same day, and more.