Understanding Closures in JavaScript
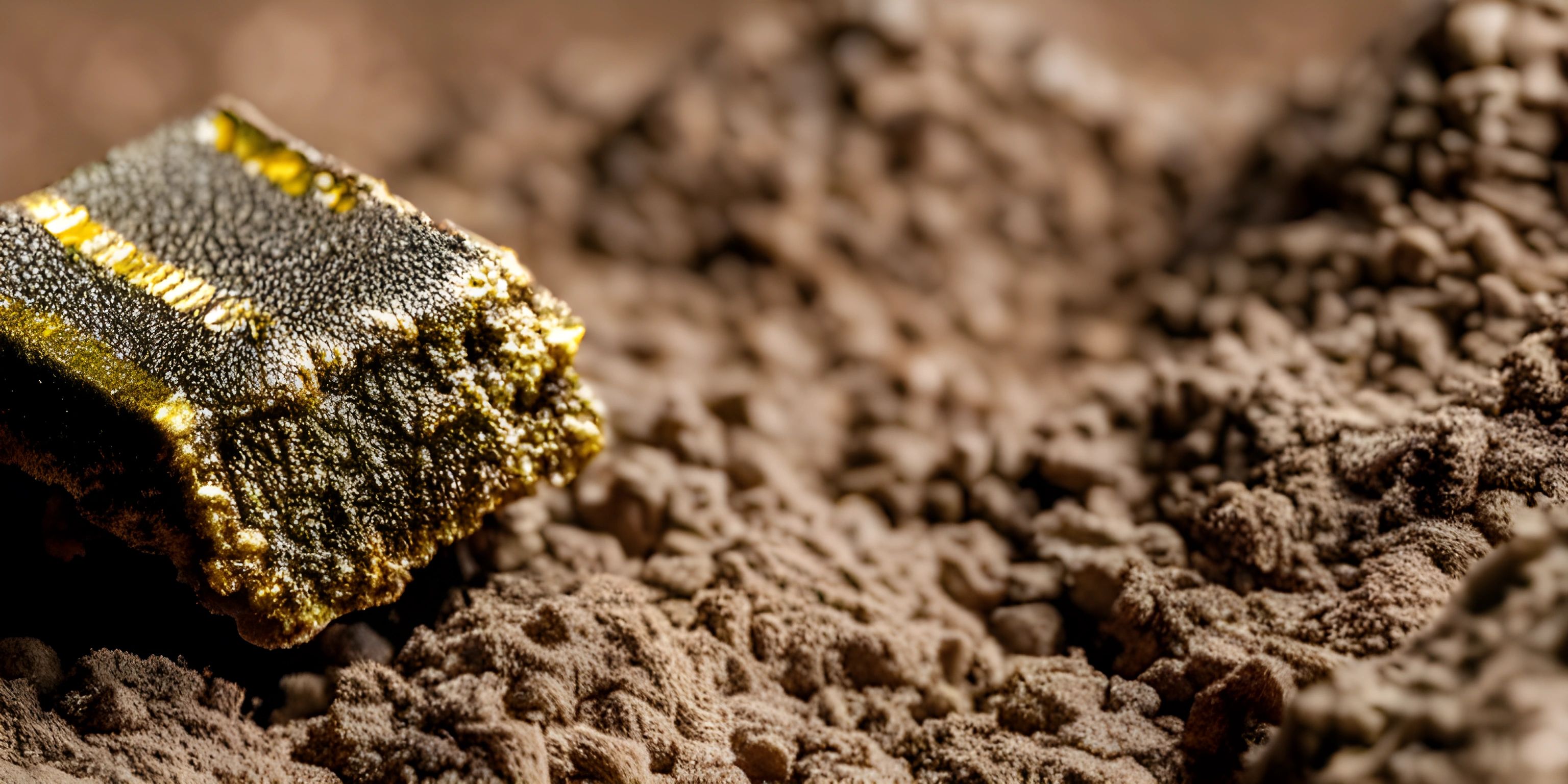
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
JavaScript closures are like the secret sauce of the language, giving your code an extra flavor of power and flexibility. If you've ever wondered how some JavaScript wizards manage to create such elegant and efficient code, closures are likely one of their tricks. Let's dig in and uncover the magic behind closures in JavaScript.
What is a Closure?
A closure is a function that remembers the environment in which it was created. This includes any variables that were in scope at the time the closure was created. In simpler terms, a closure gives you access to an outer function’s scope from an inner function. But why is this useful?
Imagine you have a function that needs to keep track of a count. You could use a global variable, but that’s not very elegant or safe. Instead, you can use a closure to encapsulate the variable, keeping it private and safe from the rest of your code.
Creating a Closure
Let's start with a basic example to see closures in action:
function createCounter() { let count = 0; // This variable is enclosed return function() { count++; return count; } } const counter = createCounter(); // createCounter's scope is remembered console.log(counter()); // 1 console.log(counter()); // 2 console.log(counter()); // 3
Here, createCounter
is a function that returns another function. When createCounter
is called, it creates a new count
variable and returns a function that increments and returns that count. The inner function forms a closure, which means it "closes over" the count
variable and keeps a reference to it even after createCounter
has finished executing.
Why Use Closures?
Closures can be used for several reasons, including:
- Data Privacy: By using closures, you can create private variables that are only accessible within a specific function. This can help prevent accidental modification of variables and make your code more robust.
- Memoization: Closures can be used to store the results of expensive function calls and return the cached result when the same inputs occur again, improving performance.
- Event Handling: Closures are often used in event handlers to maintain state between event fires.
- Function Factories: You can create functions with customized behavior by using closures to encapsulate configuration or state.
Closures in Loops
A common pitfall with closures is when they are used inside loops. Let's take a look at what can go wrong and how to fix it:
for (var i = 1; i <= 3; i++) { setTimeout(function() { console.log(i); // All three will log '4' }, 1000); }
Here, the setTimeout
function references the same i
variable, which has the value 4
by the time the timeouts execute. To fix this, we can use an immediately invoked function expression (IIFE) to create a new scope for each iteration:
for (var i = 1; i <= 3; i++) { (function(i) { setTimeout(function() { console.log(i); // 1, 2, 3 }, 1000); })(i); }
Alternatively, using let
instead of var
will also create block scope:
for (let i = 1; i <= 3; i++) { setTimeout(function() { console.log(i); // 1, 2, 3 }, 1000); }
Lexical Scoping and Closures
Closures rely on lexical scoping to function. Lexical scoping means that the scope of a variable is determined by its position within the source code, and nested functions have access to variables declared in their outer scope.
Consider this example:
function outer() { let outerVar = "I am from the outer scope!"; function inner() { console.log(outerVar); // "I am from the outer scope!" } return inner; } const innerFunc = outer(); innerFunc();
Here, inner
function has access to outerVar
because it was declared in the outer scope. Even after outer
has finished executing, innerFunc
retains access to outerVar
thanks to the closure.
Practical Uses of Closures
Data Encapsulation
One practical use of closures is to encapsulate data. This is often seen in module patterns where data is kept private and only accessible through exposed methods.
function createPerson(name) { let age = 0; return { getName: function() { return name; }, getAge: function() { return age; }, birthday: function() { age++; } }; } const person = createPerson("Alice"); console.log(person.getName()); // "Alice" console.log(person.getAge()); // 0 person.birthday(); console.log(person.getAge()); // 1
Function Factories
Closures can also be used to create factory functions that generate new functions with customized behavior.
function createMultiplier(multiplier) { return function(value) { return value * multiplier; }; } const double = createMultiplier(2); const triple = createMultiplier(3); console.log(double(5)); // 10 console.log(triple(5)); // 15
Event Handlers
Closures are handy in event handlers to maintain state between events.
function setupButton() { let clickCount = 0; document.querySelector("button").addEventListener("click", function() { clickCount++; console.log(`Button clicked ${clickCount} times`); }); } setupButton();
Conclusion
Closures are a powerful feature in JavaScript that allow functions to access variables from their enclosing scope. They enable data encapsulation, stateful functions, and more. By understanding closures, you can write more robust, maintainable, and efficient code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Palindromes (psst, it's free!).
FAQ
What is a closure in JavaScript?
A closure is a function that retains access to its enclosing scope, even after the outer function has finished executing. This allows the inner function to access variables and arguments of the outer function.
How do closures help with data privacy?
Closures allow variables to be encapsulated within a function, making them private and only accessible through specific functions. This prevents accidental modification and keeps the data safe from the global scope.
Can closures be used inside loops?
Yes, closures can be used inside loops, but you need to be careful with variable scoping. Using an IIFE or let
instead of var
can help create the correct scope for each iteration.
What is lexical scoping?
Lexical scoping means the scope of a variable is determined by its position within the source code. Nested functions have access to variables declared in their outer scope, which is essential for closures to work.
What are some practical uses of closures?
Practical uses of closures include data encapsulation, creating function factories, and maintaining state in event handlers. These uses help in writing more efficient and maintainable code.