Understanding Immediately Invoked Function Expressions (IIFE) in JavaScript
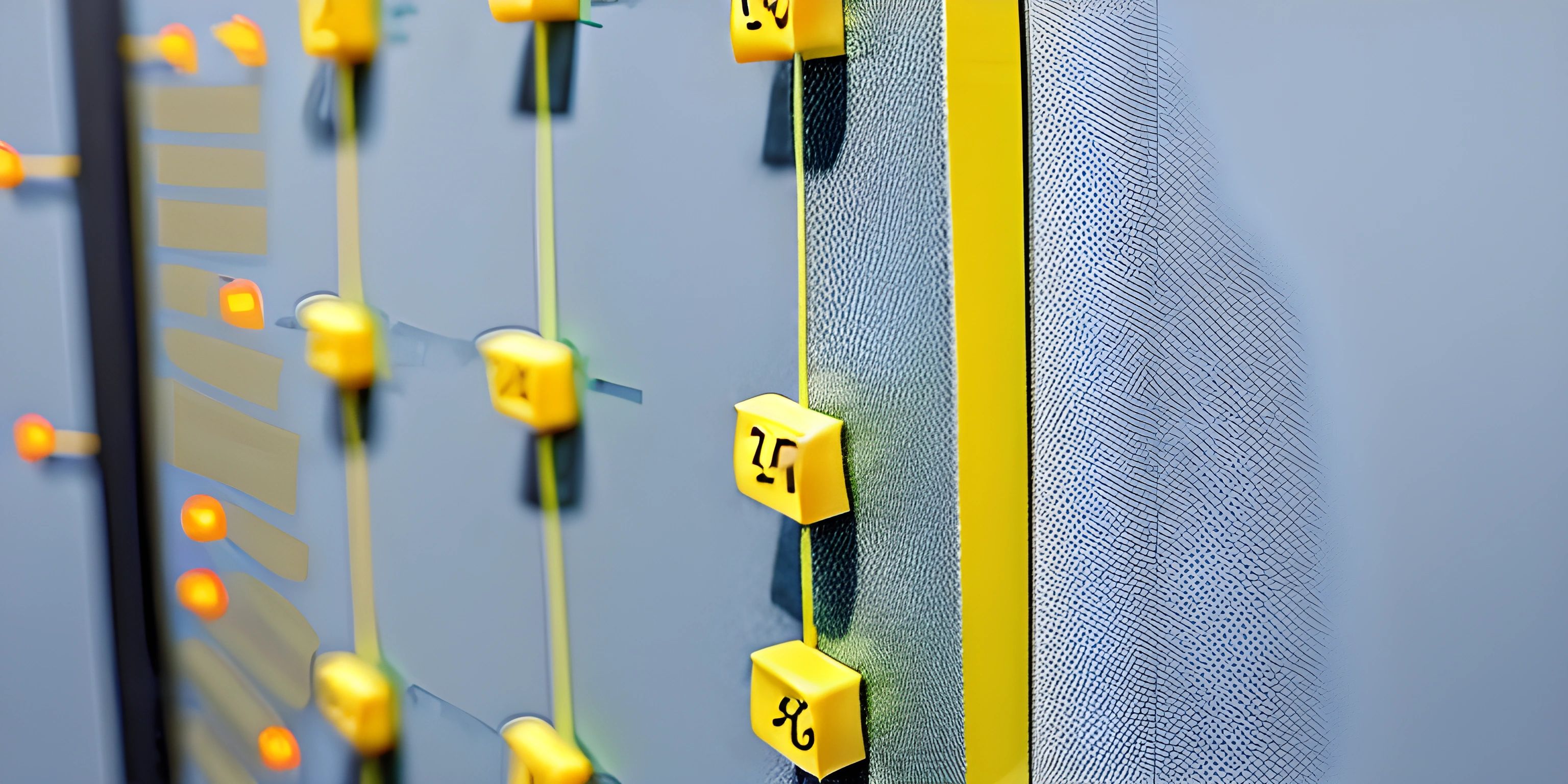
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you've ever tried to keep your secret chocolate stash safe from prying eyes, you might understand the need for the Immediately Invoked Function Expression (IIFE) in JavaScript. Just like hiding your chocolate in a secret compartment, IIFEs help encapsulate your code, making it private and safe from meddling. Let’s dive into the delicious world of IIFEs and see how they can keep your code secure and clean!
What is an IIFE?
An Immediately Invoked Function Expression (IIFE) is a function that is executed right after it's defined. This clever trick is accomplished by wrapping the function in parentheses and then immediately invoking it with another set of parentheses. It's like setting a trap for your code gremlins; they get caught before they even know what hit them!
Basic Structure
Here’s what an IIFE looks like:
(function() { console.log("Hello from the secret compartment!"); })();
In this example, the function logs a message to the console and is immediately executed after being defined. The extra parentheses at the end (()
) are what trigger the function to run instantly.
Why Use IIFEs?
IIFEs are useful for several reasons, including avoiding variable collisions, creating closures, and managing scope. Think of IIFEs as a way to build a sturdy fence around your backyard, ensuring that your variables and functions stay within their designated areas.
Avoiding Variable Collisions
One of the primary benefits of using an IIFE is to avoid variable collisions. In JavaScript, variables defined within an IIFE are not accessible outside of it, and vice versa. This prevents unintended interactions between different parts of your code.
var secret = "Top Secret"; (function() { var secret = "IIFE Secret"; console.log(secret); // Outputs: IIFE Secret })(); console.log(secret); // Outputs: Top Secret
In this example, the secret
variable inside the IIFE is completely separate from the secret
variable outside of it. They don’t step on each other's toes, much like your secret chocolate stash and your sibling's hidden candy.
Creating Closures
IIFEs are also helpful for creating closures. A closure is a function that remembers the environment in which it was created. By using an IIFE, you can create a private environment for your functions and variables.
var getCounter = (function() { var counter = 0; return function() { return counter += 1; }; })(); console.log(getCounter()); // Outputs: 1 console.log(getCounter()); // Outputs: 2
In this example, the counter
variable is enclosed within the IIFE and is only accessible through the returned function. This ensures that the counter
variable is private and cannot be tampered with from outside the IIFE.
Syntax Variations
There are different ways to write IIFEs, but they all achieve the same goal. Here are some common variations:
Classic IIFE
(function() { console.log("Classic IIFE"); })();
Using the Unary Operator
+function() { console.log("Unary Operator IIFE"); }();
Using the Not Operator
!function() { console.log("Not Operator IIFE"); }();
Arrow Function IIFE (ES6)
(() => { console.log("Arrow Function IIFE"); })();
Each of these variations serves the same purpose: to create and immediately invoke a function, protecting its scope from the outside world.
Practical Uses of IIFEs
Now that we've built our secret compartments, let's see how IIFEs can be useful in real-world scenarios.
Module Pattern
The Module Pattern is a design pattern that uses IIFEs to create private and public methods and variables. This helps in organizing code and preventing global namespace pollution.
var myModule = (function() { var privateVariable = "I am private"; function privateMethod() { console.log(privateVariable); } return { publicMethod: function() { privateMethod(); } }; })(); myModule.publicMethod(); // Outputs: I am private
In this example, myModule
exposes a publicMethod
that can access the private privateVariable
and privateMethod
, but those private members are not accessible directly from outside the IIFE.
Initialization Code
IIFEs are often used for initialization code that needs to run once and doesn't pollute the global scope.
(function() { var someInitialization = function() { console.log("Initializing..."); }; someInitialization(); })();
This ensures that the initialization logic runs immediately and stays self-contained, without leaving behind any global variables.
Conclusion
IIFEs are a powerful tool in JavaScript for managing scope, avoiding variable collisions, and creating closures. They help you keep your code organized and free from unwanted interactions, much like a well-kept secret compartment. So, the next time you need to protect your code, consider using an IIFE and enjoy the peace of mind it brings.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Palindromes (psst, it's free!).
FAQ
What does IIFE stand for?
IIFE stands for Immediately Invoked Function Expression. It's a JavaScript function that gets executed right after it's defined.
Why should I use an IIFE?
IIFEs are useful for avoiding variable collisions, creating closures, and managing scope. They help keep your code organized and prevent unintended interactions between different parts of your code.
Can I use arrow functions with IIFEs?
Yes, you can use arrow functions with IIFEs. Here's an example:
(() => { console.log("Arrow Function IIFE"); })();
What is the Module Pattern?
The Module Pattern is a design pattern that uses IIFEs to create private and public methods and variables. It helps in organizing code and preventing global namespace pollution.
Are there different ways to write IIFEs?
Yes, there are different ways to write IIFEs, including the classic IIFE, using the unary operator, using the not operator, and using arrow functions. Each variation achieves the same goal of creating and immediately invoking a function.