Understanding Java NullPointerException and How to Fix It
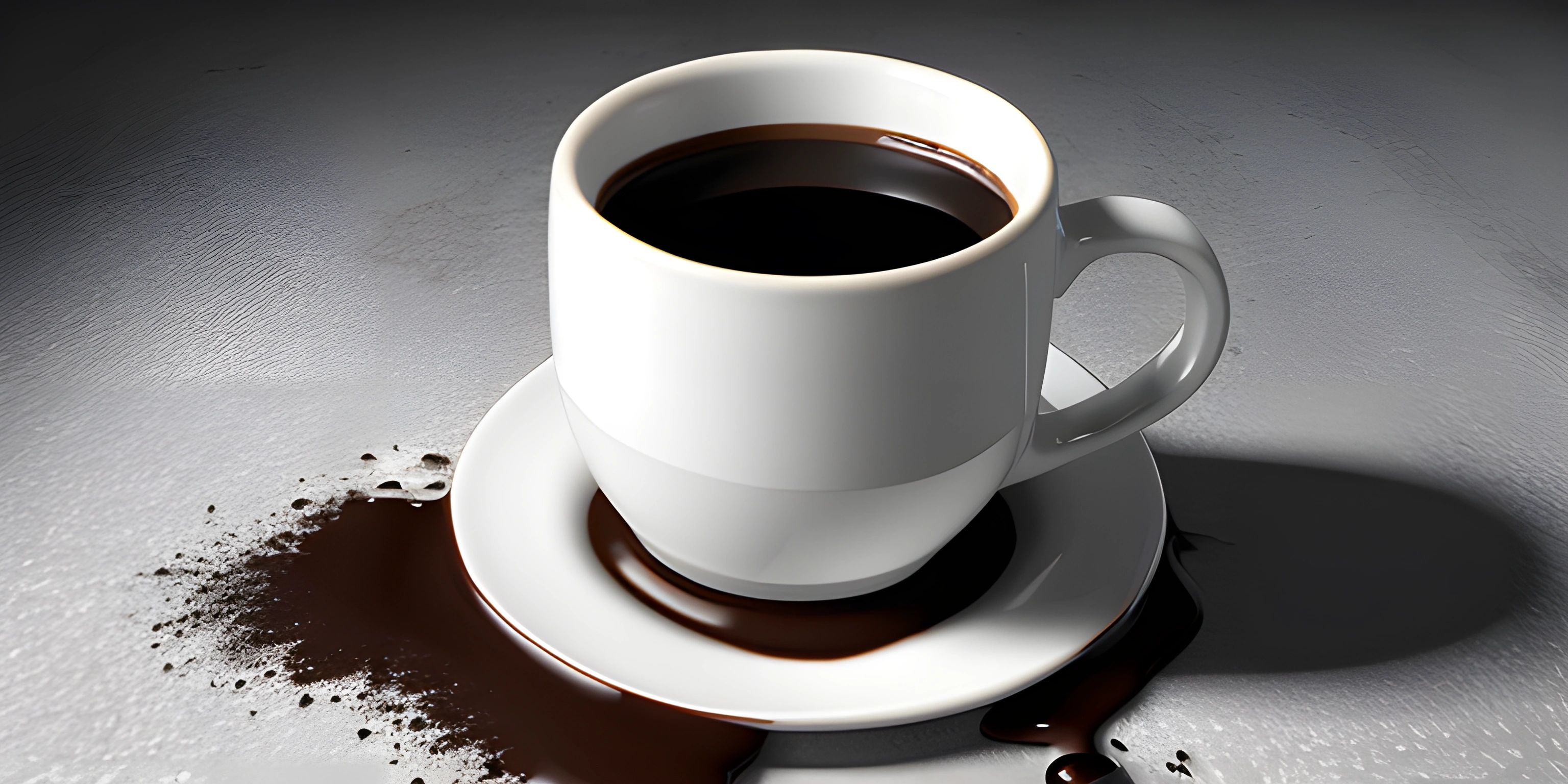
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
NullPointerException, the infamous nightmare of every Java programmer, is an exception that occurs when a reference variable points to a null value instead of an actual object. It's like trying to call a friend on the phone, but instead of dialing their number, you dial "null" and expect the call to go through. In this case, Java will throw a NullPointerException to let you know something went wrong.
Causes of NullPointerException
There are several common scenarios that can cause a NullPointerException. Here are a few:
- Calling a method on a null object reference. This is the most common cause. When you try to call a method on an object that is null, Java will throw a NullPointerException.
- Accessing or modifying a null object's field. Just like with methods, if you try to access or modify a field on a null object, a NullPointerException will occur.
- Throwing null as an exception. In some cases, you might explicitly throw a null value as an exception, which will also result in a NullPointerException.
How to Fix NullPointerException
Now that we've looked at the causes, let's dive into the solutions.
1. Check for null before calling a method or accessing a field
The most straightforward way to avoid NullPointerException is to check if an object is null before calling any methods or accessing its fields.
if (object != null) { object.method(); } else { System.out.println("Object is null"); }
2. Use Optional class
Java 8 introduced the Optional
class, which is a container object that may or may not contain a non-null value. It's a great way to avoid NullPointerExceptions by explicitly handling null cases.
import java.util.Optional; Optional<String> optionalString = Optional.ofNullable(getString()); if (optionalString.isPresent()) { System.out.println(optionalString.get()); } else { System.out.println("String is null"); }
3. Use the null-safe operator
Another approach is to use the null-safe operator ?.
, which is available in some languages like Kotlin and Groovy, but not in Java by default. However, you can use third-party libraries like Apache Commons Lang to achieve similar behavior.
import org.apache.commons.lang3.ObjectUtils; String result = ObjectUtils.defaultIfNull(getString(), "Default value"); System.out.println(result);
NullPointerException Best Practices
- Always initialize your variables. Avoid leaving variables uninitialized, as this can lead to NullPointerExceptions.
- Use the @NonNull annotation. This annotation tells the compiler that a variable should never be null, helping to prevent NullPointerExceptions.
- Write unit tests. Writing unit tests for your code can help catch NullPointerExceptions before they become an issue in production.
FAQ
What is a NullPointerException?
A NullPointerException is a runtime exception in Java that occurs when a reference variable points to a null value instead of an actual object. It is a common error that many Java developers face during their programming journey.
What are some common causes of NullPointerException?
Common causes of NullPointerException include calling a method on a null object reference, accessing or modifying a null object's field, and throwing null as an exception.
How can I fix a NullPointerException?
To fix a NullPointerException, you can check for null before calling a method or accessing a field, use the Optional class to handle null cases explicitly, or use a null-safe operator or third-party library to handle null values automatically.
What are some best practices to avoid NullPointerExceptions?
Some best practices to avoid NullPointerExceptions include always initializing your variables, using the @NonNull annotation to indicate that a variable should never be null, and writing unit tests to catch potential NullPointerExceptions before they become an issue in production.